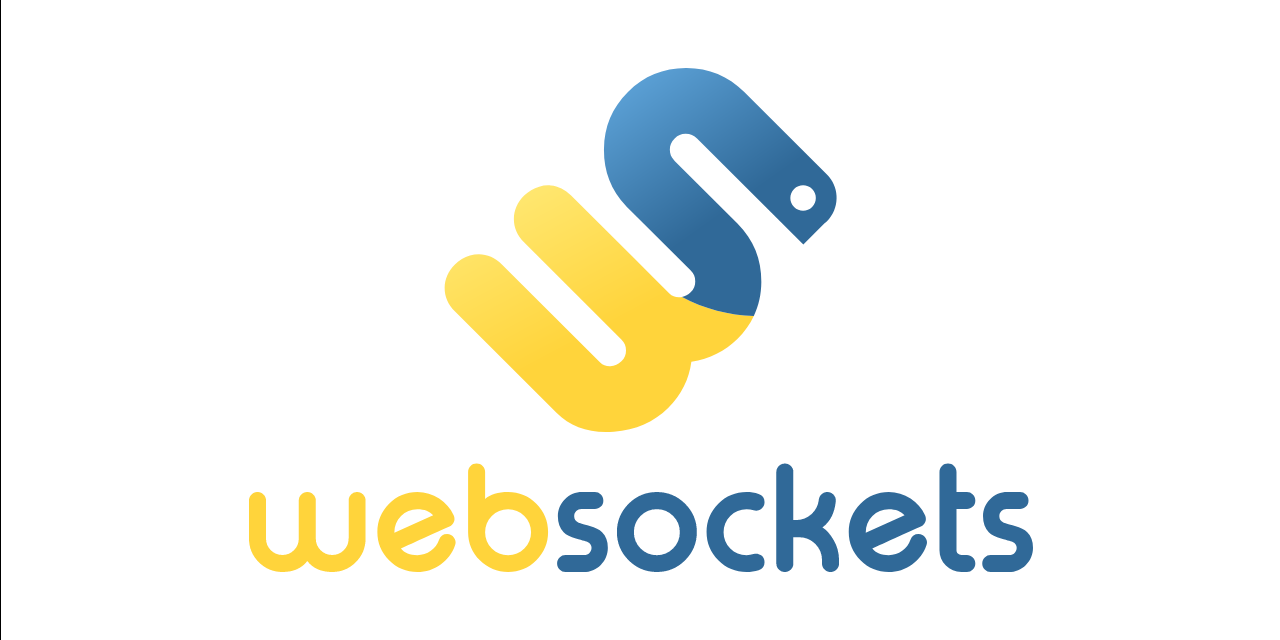
August 05, 2024
Achieving Seamless Real-Time Communication Between Frontend and Backend
In today's fast-paced digital world, users expect real-time interactions and updates within web applications. Whether it's a chat application, collaborative editing tool, or live data visualization, the ability to instantly communicate between frontend and backend is crucial for delivering a seamless user experience. Fortunately, with advancements in web technologies, achieving real-time communication has become more accessible than ever.
In this blog post, we'll explore different approaches to enable real-time communication between the frontend and backend of a web application, along with examples illustrating each method.
Traditional Approach: Polling
Polling involves the frontend making periodic requests to the backend to check for updates. While simple to implement, it's not efficient as it can lead to unnecessary network traffic and delays in receiving updates.
Example:
// Frontend code using polling
setInterval(() => {
fetch('/api/data')
.then(response => response.json())
.then(data => {
// Update UI with new data
})
.catch(error => {
console.error('Error fetching data:', error);
});
}, 5000); // Poll every 5 seconds
Long Polling
Long polling is an improvement over traditional polling where the backend holds the request open until new data is available or a timeout occurs. It reduces the number of unnecessary requests compared to regular polling.
Example:
// Frontend code using long polling
function fetchData() {
fetch('/api/data')
.then(response => response.json())
.then(data => {
// Update UI with new data
fetchData(); // Make another request
})
.catch(error => {
console.error('Error fetching data:', error);
setTimeout(fetchData, 5000); // Retry after delay
});
}
fetchData(); // Initial call to start long polling
WebSockets
WebSockets provide full-duplex communication channels over a single, long-lived TCP connection. They offer real-time, bi-directional communication between the frontend and backend, making them ideal for applications requiring instant updates.
Example:
// Frontend code using WebSockets
const socket = new WebSocket('ws://localhost:3000');
socket.addEventListener('open', () => {
console.log('Connected to WebSocket server');
});
socket.addEventListener('message', event => {
const data = JSON.parse(event.data);
// Update UI with new data
});
socket.addEventListener('close', () => {
console.log('Connection closed');
});
// Backend code (Node.js) handling WebSocket connections
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 3000 });
wss.on('connection', ws => {
console.log('Client connected');
ws.send(JSON.stringify({ message: 'Hello from server' }));
ws.on('message', message => {
console.log('Received message from client:', message);
});
});
Conclusion
Real-time communication between frontend and backend is essential for building modern web applications that deliver dynamic and engaging user experiences. While traditional polling methods have their place, technologies like long polling and WebSockets offer more efficient and responsive solutions.
When choosing the right approach for your application, consider factors such as scalability, latency, and complexity. Each method has its advantages and limitations, so it's essential to weigh them against your project requirements.
By leveraging these techniques effectively, you can create web applications that keep users engaged and informed in real-time, ultimately enhancing their overall experience.
636 views