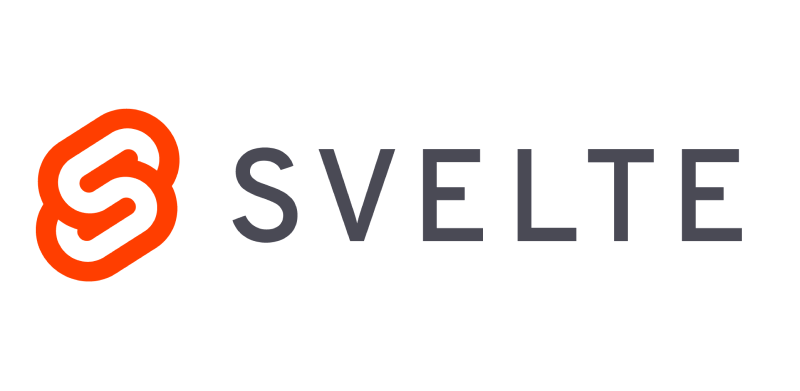
May 23, 2024
Mastering Web Development with Svelte: A Comprehensive Tutorial
Are you a web developer looking for a modern, efficient, and intuitive way to build web applications? Look no further than Svelte! In this tutorial, we'll dive deep into the world of Svelte, a radical new approach to building user interfaces.
What is Svelte?
Svelte is a radical new approach to building user interfaces. It shifts the work of rendering from the browser to the build step, producing highly optimized JavaScript code that updates the DOM when the state of your app changes. This results in smaller bundle sizes and better performance compared to traditional frameworks like React or Vue.
Why Svelte?
Svelte offers several advantages over traditional frameworks:
Easy to Learn: With its simple syntax and minimalistic approach, Svelte is easy to pick up for both beginners and experienced developers.
Performance: By moving the heavy lifting to the compilation step, Svelte generates highly optimized code that leads to faster load times and better runtime performance.
No Virtual DOM: Unlike other frameworks, Svelte doesn't rely on a virtual DOM for updating the UI. Instead, it directly updates the DOM, resulting in faster rendering.
Small Bundle Sizes: Svelte generates code that is highly optimized and doesn't include unnecessary runtime overhead, resulting in smaller bundle sizes.
Now, let's dive into a hands-on tutorial to get you started with Svelte.
Getting Started
To follow along with this tutorial, make sure you have Node.js and npm installed on your machine. You can install Svelte using npm by running the following command:
npm install -g degit # Install degit globally
npx degit sveltejs/template svelte-app # Create a new Svelte project
cd svelte-app # Navigate to the project directory
npm install # Install project dependencies
npm run dev # Start the development server
This will create a new Svelte project and start a development server. You can now access your Svelte app by navigating to http://localhost:5000 in your browser.
Creating Components
In Svelte, components are the building blocks of your application. Let's create a simple component that displays a greeting message.
Create a new file called Greeting.svelte in the src directory and add the following code:
<script>
let name = 'World';
</script>
<h1>Hello {name}!</h1>
<style>
h1 {
color: #333;
}
</style>
This component defines a variable name with a default value of 'World' and renders a greeting message using that variable.
Using Components
Now, let's use the Greeting component in our main app.
Open App.svelte in the src directory and replace its contents with the following code:
<script>
import Greeting from './Greeting.svelte';
</script>
<Greeting />
This imports the Greeting component and renders it inside the App component.
Adding Interactivity
Svelte makes it easy to add interactivity to your components. Let's update the Greeting component to allow the user to enter their name.
Replace the contents of Greeting.svelte with the following code:
<script>
let name = 'World';
function handleChange(event) {
name = event.target.value;
}
</script>
<h1>Hello {name}!</h1>
<input type="text" bind:value={name} on:input={handleChange} />
<style>
h1 {
color: #333;
}
</style>
Now, the Greeting component includes an input field that allows the user to enter their name. The greeting message updates in real-time as the user types.
Conclusion
In this tutorial, we've only scratched the surface of what Svelte can do. Its simple syntax, excellent performance, and easy-to-understand concepts make it a powerful tool for building modern web applications. I encourage you to explore the official Svelte documentation and experiment with building your own projects. Happy coding!
421 views