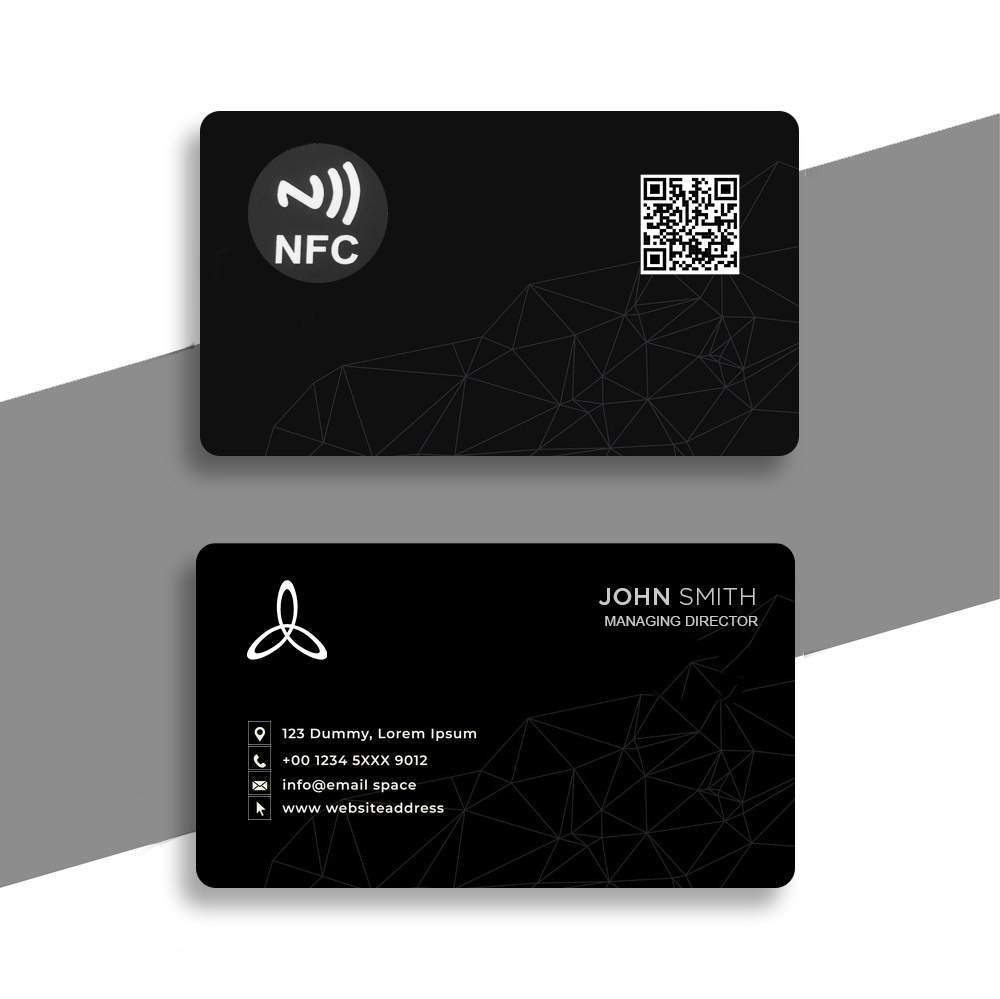
May 08, 2024
Exploring NFC Card Programming with JavaScript: A Beginner's Guide
In today's digital world, Near Field Communication (NFC) technology is becoming increasingly prevalent, especially in applications like contactless payments, access control systems, and identification. But did you know that you can harness the power of JavaScript to interact with NFC cards? In this blog post, we'll delve into the exciting realm of programming NFC cards using JavaScript, exploring the basics and providing a hands-on example to get you started.
Understanding NFC Technology
Before diving into programming, let's grasp the fundamentals of NFC technology. NFC allows for short-range communication between electronic devices, typically within a few centimeters. This technology enables devices to exchange data wirelessly, making it ideal for various applications, including mobile payments, smart posters, and data transfer between devices.
Programming NFC Cards with JavaScript
To interact with NFC cards using JavaScript, we can utilize web-based NFC APIs provided by modern web browsers. These APIs enable web applications to read and write data to NFC tags and cards directly from the browser, without the need for additional plugins or native code.
Example: Writing Data to an NFC Card
Let's walk through a simple example of how to write data to an NFC card using JavaScript. For this example, we'll create a basic web application that prompts the user to input some text and then writes this text to an NFC card when the card is tapped on the device.
Step 1: Setting Up the HTML Structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>NFC Card Programming with JavaScript</title>
</head>
<body>
<input type="text" id="textInput" placeholder="Enter text to write">
<button id="writeButton">Write to NFC Card</button>
<script src="script.js"></script>
</body>
</html>
Step 2: Implementing the JavaScript Logic
// script.js
// Function to handle writing data to the NFC card
async function writeToNFC(data) {
try {
const writer = new NDEFWriter();
await writer.write(data);
console.log("Data written to NFC card successfully!");
} catch (error) {
console.error("Error writing data to NFC card:", error);
}
}
// Event listener for the write button
document.getElementById("writeButton").addEventListener("click", async () => {
const textInput = document.getElementById("textInput").value;
if (textInput) {
// Convert text input to a NDEF record
const textRecord = new NDEFRecord("text", textInput);
// Write the NDEF record to the NFC card
await writeToNFC(textRecord);
} else {
console.warn("Please enter some text to write to the NFC card.");
}
});
Step 3: Testing the Application
Now, open the HTML file in a web browser that supports NFC APIs (such as Chrome on Android). When you tap an NFC card on your device and click the "Write to NFC Card" button after entering some text, the data will be written to the card.
Conclusion
In this blog post, we've explored the fascinating world of programming NFC cards with JavaScript. By leveraging web-based NFC APIs, developers can create powerful web applications capable of interacting with NFC-enabled devices and cards. While this example provides a basic introduction, there's much more to explore in the realm of NFC programming. So, grab your NFC-enabled device and start experimenting with JavaScript to unlock the potential of NFC technology!
315 views