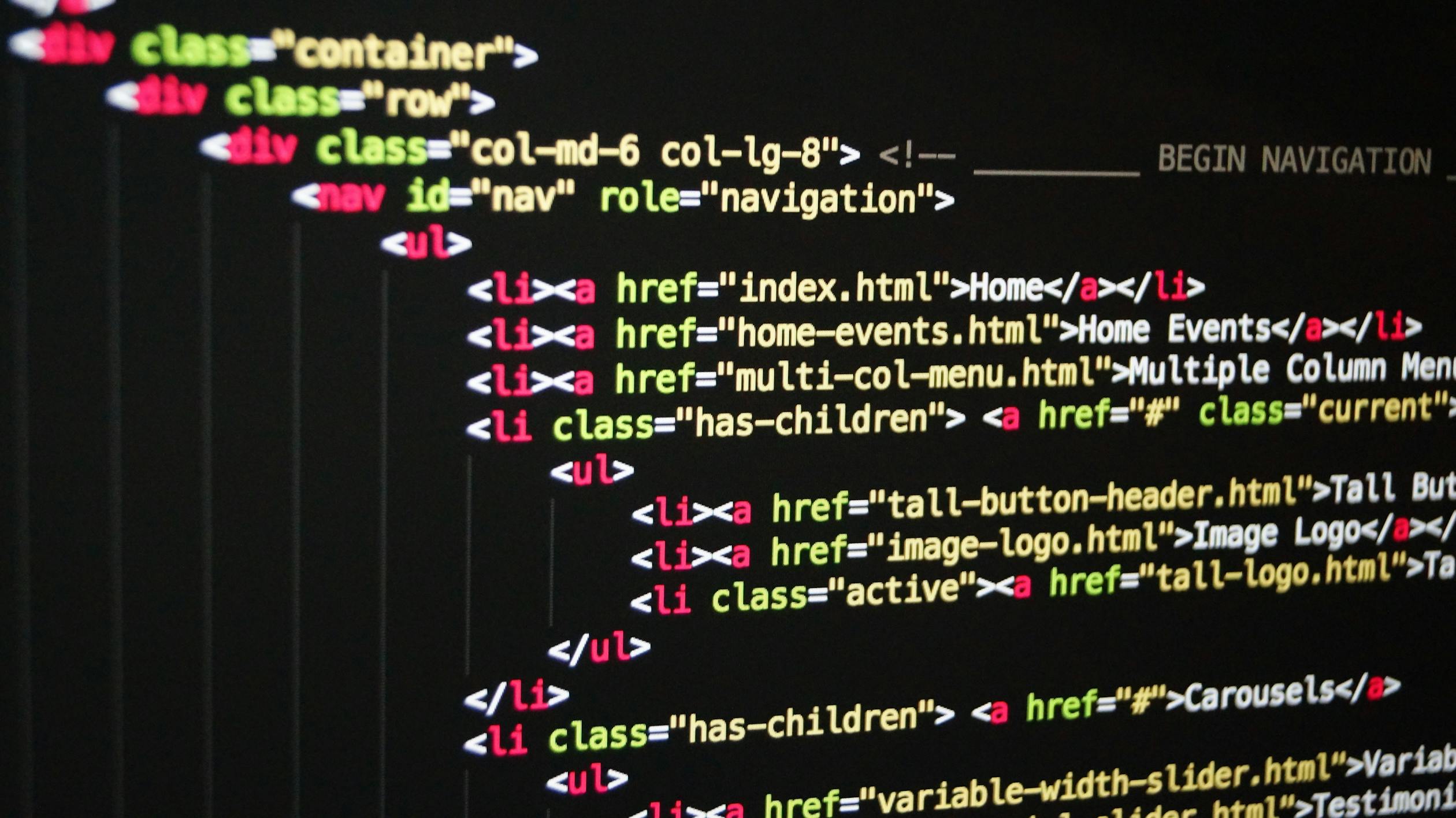
Polymorphic relationships are a powerful feature in Laravel that allow you to associate a model with more than one other type of model. In this tutorial, we are going to demonstrate how this versatile feature can be applied in a real-world scenario. Imagine you're building a content management system, and you have models for Article, Video, and Comment. In this context, an Article can have many comments, and a Video can also have many comments.
Polymorphic relationships come to the rescue by enabling us to create a flexible and reusable structure for handling comments across both articles and videos.
Join us as we dive into the world of Laravel polymorphic relationships and learn how to implement them step by step, complete with code examples
Create Models and Migrations
Generate the models and migrations for the Article, Video, and Comment models:
php artisan make:model Article -m
php artisan make:model Video -m
php artisan make:model Comment -m
Edit the generated migration files to define the schema for your tables. For example:
In create_articles_table.php:
public function up()
{
Schema::create('articles', function (Blueprint $table) {
$table->id();
$table->string('title');
// Add other article-specific columns here
$table->timestamps();
});
}
In create_videos_table.php:
public function up()
{
Schema::create('videos', function (Blueprint $table) {
$table->id();
$table->string('title');
// Add other video-specific columns here
$table->timestamps();
});
}
In create_comments_table.php:
public function up()
{
Schema::create('comments', function (Blueprint $table) {
$table->id();
$table->text('body');
$table->unsignedBigInteger('commentable_id');
$table->string('commentable_type');
$table->timestamps();
});
}
Run Migrations
Run the migrations to create the database tables:
php artisan migrate
Define Relationships
In your model files (Article.php, Video.php, and Comment.php), define the relationships:
In Article.php:
public function comments()
{
return $this->morphMany(Comment::class, 'commentable');
}
In Video.php:
public function comments()
{
return $this->morphMany(Comment::class, 'commentable');
}
In Comment.php:
public function commentable()
{
return $this->morphTo();
}
Create and Retrieve Comments
Now you can create and retrieve comments for both articles and videos using the polymorphic relationship.
To create a comment for an article:
$article = Article::find(1);
$comment = new Comment(['body' => 'A comment on an article.']);
$article->comments()->save($comment);
To create a comment for a video:
$video = Video::find(1);
$comment = new Comment(['body' => 'A comment on a video.']);
$video->comments()->save($comment);
To retrieve comments for an article or video:
$article = Article::find(1);
$comments = $article->comments;
$video = Video::find(1);
$comments = $video->comments;
Display Comments
In your views, you can display comments for both articles and videos.
For example, in your article view:
@foreach ($article->comments as $comment)
<div class="comment">
{{ $comment->body }}
</div>
@endforeach
And in your video view:
@foreach ($video->comments as $comment)
<div class="comment">
{{ $comment->body }}
</div>
@endforeach
Summary
Polymorphic relationships, as you've seen, are a vital tool in Laravel for building versatile applications where different models need to interact with a common feature, such as comments. They enable you to write cleaner and more maintainable code by reusing database tables and relationships across various parts of your application.
With the knowledge gained from this tutorial, you can now implement polymorphic relationships in your Laravel projects, improving their efficiency and maintainability. So, go ahead and apply this powerful Laravel feature to your own real-world projects and unlock new possibilities in your development journey.
207 views