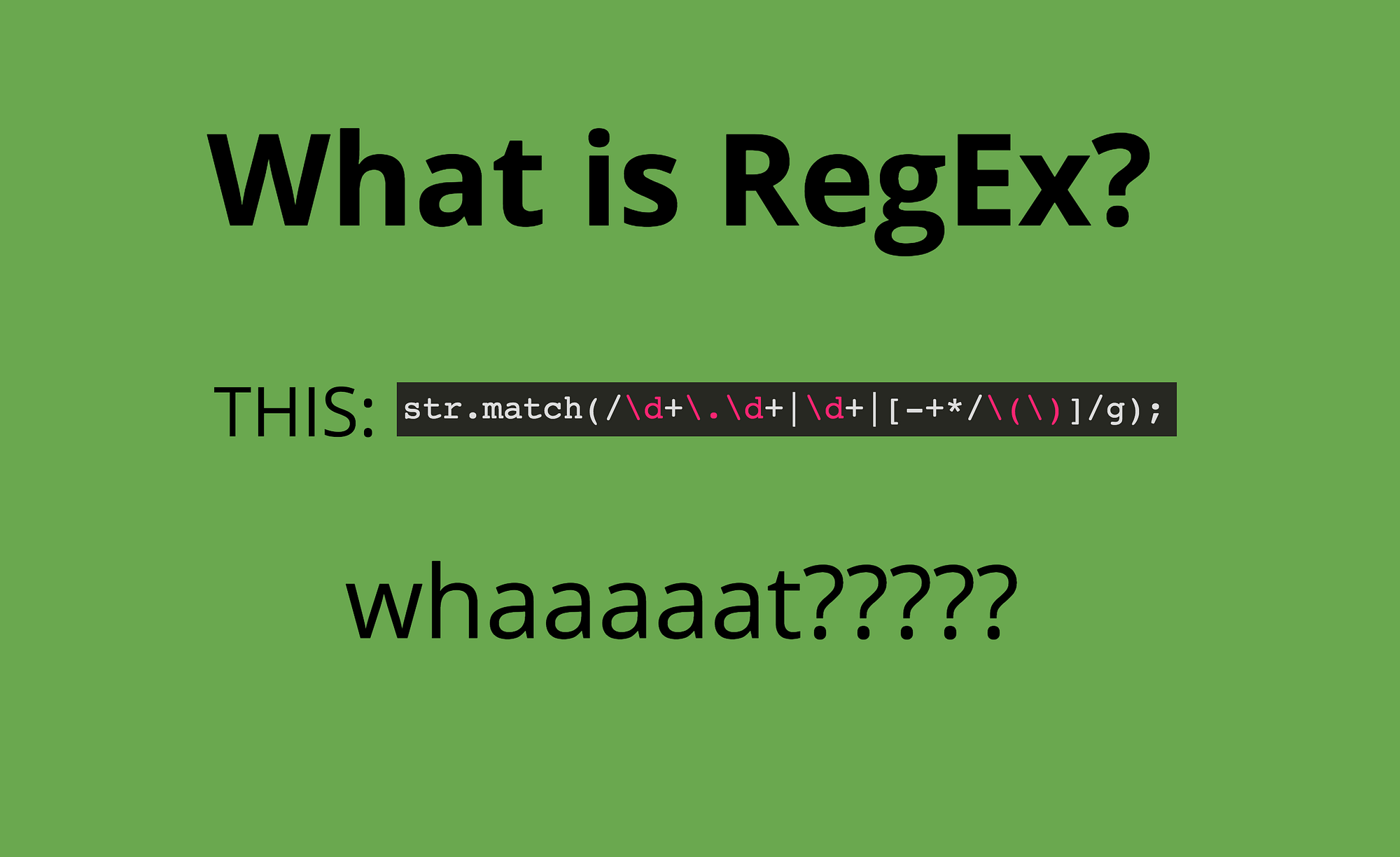
July 19, 2024
Unleashing the Power of Regular Expressions in JavaScript: A Comprehensive Guide
Introduction:
Regular expressions, commonly abbreviated as regex, are powerful tools for pattern matching and manipulation of strings in JavaScript. Despite their intimidating appearance, mastering regex can significantly enhance your ability to search, validate, and manipulate text data efficiently. In this guide, we'll explore the fundamentals of regex in JavaScript, from basic syntax to advanced techniques.
Understanding Regex Basics:
At its core, a regular expression is a sequence of characters that define a search pattern. In JavaScript, regex can be created using the RegExp constructor or written directly as a literal enclosed within forward slashes (/). For example:
// Using RegExp constructor
const regex = new RegExp('pattern');
// Using regex literal
const regex = /pattern/;
Regex patterns can include a combination of literal characters, metacharacters, quantifiers, and modifiers. Some common metacharacters include:
. (dot): Matches any single character except newline (\n).
\d: Matches any digit character (equivalent to [0-9]).
\w: Matches any word character (alphanumeric character plus underscore).
\s: Matches any whitespace character.
^: Matches the start of a string.
$: Matches the end of a string.
Basic Regex Examples:
Matching a specific word:
const regex = /hello/;
Matching any digit:
const regex = /\d/;
Matching an email pattern:
const regex = /\w+@\w+\.\w+/;
Using Regex Methods in JavaScript:
JavaScript provides several methods for working with regular expressions, including test(), exec(), match(), replace(), and search().
- test(): Checks if a string matches a regex pattern, returning true or false.
- exec(): Returns an array containing all matched groups, or null if no match is found.
- match(): Returns an array containing all matches, or null if no match is found.
- replace(): Replaces matched substrings with a new substring.
- search(): Returns the index of the first match, or -1 if no match is found.
Regex Examples Using Methods:
Testing if a string contains a specific pattern:
const regex = /hello/;
const str = 'hello world';
console.log(regex.test(str)); // Output: true
Extracting all matches from a string:
const regex = /\d+/;
const str = 'I have 3 apples and 5 oranges';
console.log(str.match(regex)); // Output: ["3", "5"]
Replacing matched patterns in a string:
const regex = /apples/g;
const str = 'I have apples and apples';
console.log(str.replace(regex, 'bananas')); // Output: "I have bananas and bananas"
Advanced Regex Techniques:
Regex can become quite complex, especially when dealing with more intricate patterns. Some advanced techniques include:
- Grouping: Using parentheses to group parts of a pattern together.
- Quantifiers: Specifying how many times a character or group should be repeated.
- Lookaheads and lookbehinds: Assertions that check for patterns ahead or behind the current position.
- Flags: Modifiers that alter regex behavior, such as case insensitivity (i), global matching (g), and multiline matching (m).
Conclusion:
Regular expressions are indispensable tools for text processing in JavaScript. By mastering regex fundamentals and exploring advanced techniques, you can streamline your code and handle string manipulation tasks with ease. While regex may seem daunting at first, practice and experimentation will lead to proficiency and open up new possibilities in your JavaScript projects.
653 views