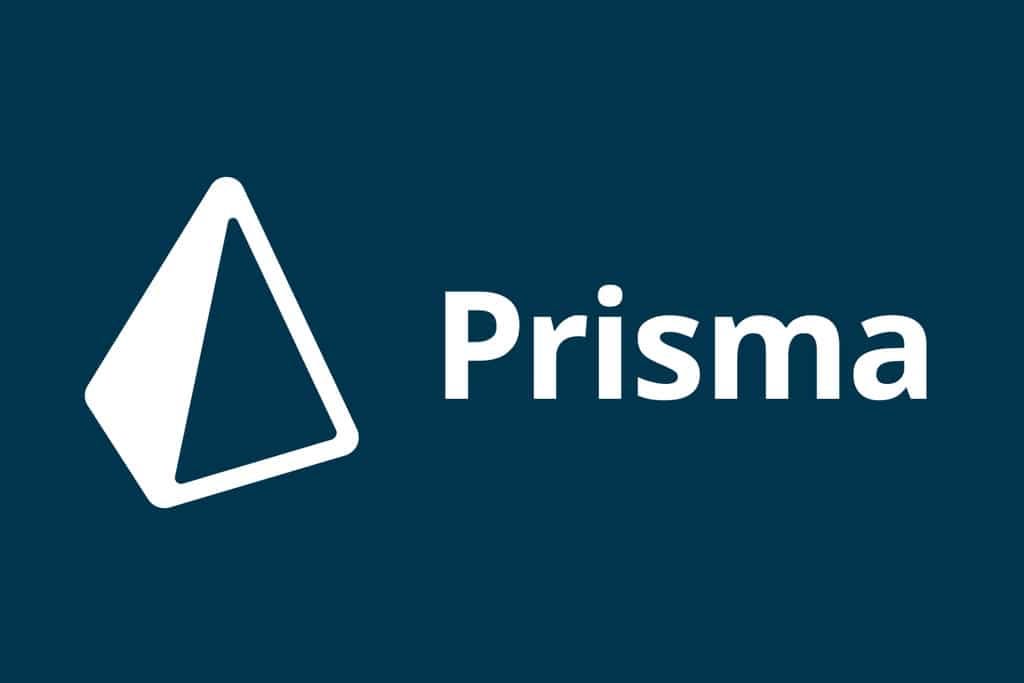
May 01, 2024
Unlocking the Power of Prisma ORM: A Crash Course
In the realm of software development, efficiency and reliability are paramount. Developers are constantly on the lookout for tools and frameworks that streamline their workflow while ensuring robustness and scalability. One such tool that has gained significant traction in recent years is Prisma ORM.
Prisma ORM, short for Object-Relational Mapping, is an open-source database toolkit that simplifies database access for developers. It provides a type-safe and expressive way to interact with databases, allowing developers to focus more on building features rather than writing repetitive SQL queries.
Understanding Prisma ORM
At its core, Prisma ORM serves as a bridge between your application code and the underlying database. It enables you to define your data model using a declarative syntax and then automatically generates the necessary SQL queries to interact with the database. This abstraction layer not only reduces the amount of boilerplate code but also helps prevent common pitfalls such as SQL injection attacks.
One of the key features of Prisma ORM is its strong emphasis on type safety. By leveraging TypeScript or JavaScript's type system, Prisma ensures that your database queries are validated at compile time, catching potential errors before they manifest at runtime. This approach not only enhances code quality but also makes refactoring and code maintenance much easier.
Getting Started with Prisma ORM
To embark on your journey with Prisma ORM, you'll first need to install the Prisma CLI, which provides a set of command-line tools for managing your database schema and generating Prisma client code. Once installed, you can initialize a new Prisma project using the prisma init command, which will scaffold the necessary files and directories for your project.
Next, you'll define your data model using the Prisma Schema Language, a concise and intuitive syntax for describing your database schema. This schema serves as the blueprint for your database tables, specifying the data types, relationships, and constraints.
Here's a simple example of a Prisma schema defining a User model:
// schema.prisma
model User {
id Int @id @default(autoincrement())
name String
email String @unique
createdAt DateTime @default(now())
}
Once you've defined your schema, you can use the Prisma CLI to generate the Prisma client, a type-safe database client tailored to your schema. The Prisma client exposes a set of methods for performing CRUD operations (Create, Read, Update, Delete) on your database tables, abstracting away the underlying SQL queries.
Interacting with the Database
With the Prisma client generated, you can now seamlessly interact with your database from within your application code. Here's a quick example demonstrating how to create a new user and retrieve a list of users:
// app.ts
import { PrismaClient } from '@prisma/client';
const prisma = new PrismaClient();
async function main() {
// Create a new user
const newUser = await prisma.user.create({
data: {
name: 'John Doe',
email: 'john@example.com',
},
});
console.log('Created user:', newUser);
// Retrieve all users
const users = await prisma.user.findMany();
console.log('All users:', users);
}
main()
.catch((e) => console.error(e))
.finally(async () => {
await prisma.$disconnect();
});
In this example, we're using the user.create method to create a new user and the user.findMany method to retrieve all users from the database. The Prisma client takes care of generating the appropriate SQL queries based on our schema definitions.
Conclusion
Prisma ORM offers a modern and developer-friendly approach to database access, empowering developers to build robust and scalable applications with ease. By abstracting away the complexities of database management and providing a type-safe interface, Prisma simplifies the development process while enhancing code quality and maintainability.
This crash course has provided a glimpse into the capabilities of Prisma ORM, but there's much more to explore. Whether you're building a small-scale application or a large-scale enterprise system, Prisma ORM has the tools and features to meet your database needs efficiently and effectively. So why not give it a try and see the difference it can make in your development workflow?
513 views