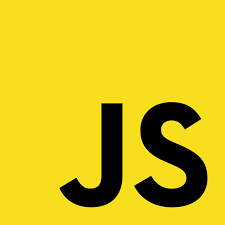
April 19, 2024
Exploring Design Patterns in React: Enhancing Scalability and Maintainability
React has revolutionized the way developers build user interfaces by introducing a component-based architecture. However, as applications grow in complexity, maintaining scalability and ensuring maintainability becomes crucial. This is where design patterns come into play, offering reusable solutions to common problems. In this article, we'll jump into some of the most widely used design patterns in React, accompanied by code examples.
Container/Presenter Pattern
The Container/Presenter pattern, also known as the Smart/Dumb pattern, separates concerns by distinguishing between components responsible for logic (containers) and components focused solely on presentation (presenters). This promotes better code organization and reusability.
// Container Component
import React, { Component } from 'react';
import PresenterComponent from './PresenterComponent';
class ContainerComponent extends Component {
state = {
data: [],
};
componentDidMount() {
// Fetch data and update state
}
render() {
return <PresenterComponent data={this.state.data} />;
}
}
export default ContainerComponent;
// Presenter Component
import React from 'react';
const PresenterComponent = ({ data }) => (
<div>
{data.map(item => (
<div key={item.id}>{item.name}</div>
))}
</div>
);
export default PresenterComponent;
Higher Order Components (HOC)
HOC is a design pattern that allows code reuse through the concept of wrapping components within other components. It's particularly useful for cross-cutting concerns like authentication, logging, or code sharing.
// HOC for adding authentication
import React from 'react';
const withAuth = (WrappedComponent) => {
const isAuthenticated = true; // Check authentication logic here
return class extends React.Component {
render() {
if (isAuthenticated) {
return <WrappedComponent {...this.props} />;
} else {
return <p>Please log in to access this content.</p>;
}
}
};
};
export default withAuth;
// Usage of HOC
import React from 'react';
import withAuth from './withAuth';
const MyComponent = () => (
<div>
<h1>Authenticated Component</h1>
</div>
);
export default withAuth(MyComponent);
Render Props
Render Props is a pattern that involves passing a function as a prop to share code between components. It's highly versatile and allows components to be more flexible by providing rendering control to the parent component.
// Render Props Component
import React from 'react';
class Mouse extends React.Component {
state = { x: 0, y: 0 };
handleMouseMove = (event) => {
this.setState({ x: event.clientX, y: event.clientY });
};
render() {
return (
<div style={{ height: '100%' }} onMouseMove={this.handleMouseMove}>
{this.props.render(this.state)}
</div>
);
}
}
export default Mouse;
// Usage of Render Props Component
import React from 'react';
import Mouse from './Mouse';
const App = () => (
<div>
<h1>Move the mouse around!</h1>
<Mouse render={({ x, y }) => (
<p>The current mouse position is ({x}, {y})</p>
)} />
</div>
);
export default App;
These are just a few examples of design patterns that can greatly enhance the structure and maintainability of React applications. By understanding and implementing these patterns, developers can write more modular, scalable, and maintainable code. Whether it's separating concerns with the Container/Presenter pattern, enhancing reusability with Higher Order Components, or achieving flexibility with Render Props, leveraging these design patterns can lead to more stable React applications.
500 views