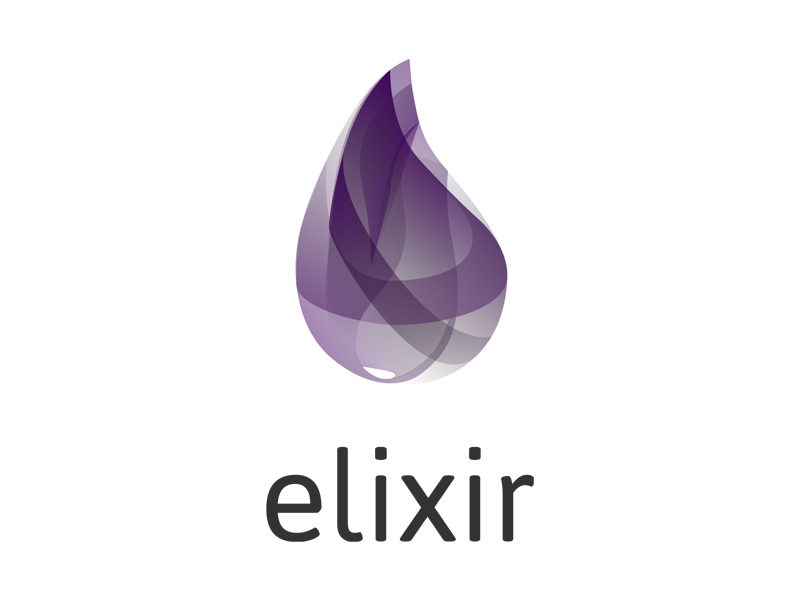
June 20, 2024
Building a RESTful Books API in Elixir: A Comprehensive Guide
In the ever-expanding landscape of web development, creating robust and efficient APIs is crucial for building modern applications. Elixir, with its concurrency model and functional programming paradigm, provides an excellent platform for crafting high-performance backend systems. In this guide, we'll walk through the process of building a RESTful Books API using Elixir, demonstrating how to leverage its unique features to create a scalable and maintainable solution.
Why Elixir for API Development?
Elixir offers a compelling combination of performance, scalability, and fault tolerance, making it an ideal choice for building APIs. Its concurrency model, inspired by Erlang's actor-based approach, allows Elixir applications to handle thousands of simultaneous connections with ease. Additionally, Elixir's functional programming paradigm promotes code clarity, testability, and maintainability, enabling developers to build robust and scalable systems.
Setting Up the Project
Let's kickstart our project by creating a new Elixir application. We'll use Mix, Elixir's build tool, to scaffold the project structure:
mix new books_api --module BooksAPI
Next, navigate to the project directory:
cd books_api
Designing the Data Model
Before diving into API implementation, let's define the data model for our Books API. For simplicity, we'll create a Book module with basic attributes such as title, author, isbn, and published_year. Open lib/books_api/book.ex and define the module as follows:
defmodule BooksAPI.Book do
defstruct [:id, :title, :author, :isbn, :published_year]
end
Implementing CRUD Operations
Now, let's focus on implementing CRUD (Create, Read, Update, Delete) operations for managing books. We'll create a Books module responsible for handling these operations. Create a new file lib/books_api/books.ex and define the module:
defmodule BooksAPI.Books do
@books []
def list_books, do: @books
def get_book(id), do: Enum.find(@books, &(&1.id == id))
def add_book(book) do
book_id = Enum.count(@books) + 1
updated_books = [@books | %{book | id: book_id}]
{:ok, updated_books}
end
def update_book(id, attrs) do
updated_books = Enum.map(@books, fn book ->
if book.id == id, do: %{book | attrs}, else: book
end)
{:ok, updated_books}
end
def delete_book(id) do
updated_books = Enum.filter(@books, fn book -> book.id != id end)
{:ok, updated_books}
end
end
Building the API Endpoints
With the CRUD operations in place, we can now define the API endpoints using Phoenix, a web framework for Elixir. Let's create a new Phoenix project within our existing Elixir application:
mix phx.new books_api --no-ecto --no-html
Phoenix will generate the necessary files and directories for our project structure. Next, let's define the routes and controllers for our Books API.
Conclusion
In this guide, we've explored the process of building a RESTful Books API using Elixir. By leveraging Elixir's concurrency model and functional programming paradigm, we can create high-performance and maintainable backend systems. With Phoenix's web framework, building APIs becomes a streamlined process, allowing developers to focus on delivering robust and scalable solutions. Whether you're building a simple CRUD API or a complex distributed system, Elixir provides the tools and paradigms necessary to meet the demands of modern web development.
518 views