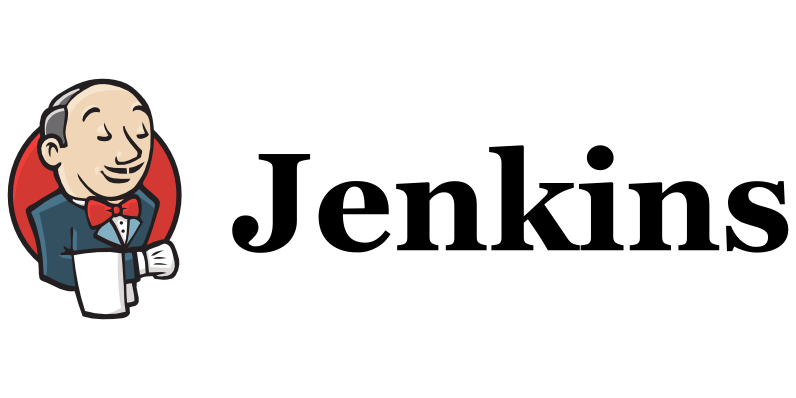
April 16, 2024
Simplifying CI/CD on AWS with Jenkins and Terraform: A Step-by-Step Guide
In today's fast-paced software development landscape, Continuous Integration/Continuous Deployment (CI/CD) has become indispensable for teams striving to deliver high-quality software rapidly. Jenkins, an open-source automation server, plays a vital role in enabling CI/CD pipelines. When combined with the scalability and flexibility of Amazon Web Services (AWS) and the infrastructure-as-code capabilities of Terraform, teams can achieve efficient, automated deployment processes that seamlessly integrate with their AWS environments.
Understanding Jenkins
Jenkins is renowned for its flexibility, extensibility, and vast plugin ecosystem, making it a popular choice for automating various aspects of the software development lifecycle. It facilitates the automation of building, testing, and deploying applications, streamlining the development process and ensuring continuous integration and delivery.
Leveraging AWS for Scalability and Reliability
AWS provides a comprehensive suite of cloud services, offering scalability, reliability, and security to applications and infrastructure. By harnessing AWS services, teams can leverage resources such as EC2 instances, S3 buckets, RDS databases, and more, to build robust, scalable architectures for their applications.
Terraform: Infrastructure as Code for AWS
Terraform simplifies the management of cloud infrastructure by allowing developers to define their infrastructure as code. With Terraform, teams can declaratively define the resources and their configurations using a simple, human-readable language. This approach ensures consistency, repeatability, and version control of infrastructure changes, making it an ideal choice for managing AWS resources.
Integrating Jenkins with AWS Using Terraform
Integrating Jenkins with AWS using Terraform allows teams to automate the provisioning of Jenkins servers, configure them according to their requirements, and seamlessly integrate them with their AWS environments. Here's a high-level overview of the process:
Infrastructure Provisioning: Use Terraform to define the AWS resources required for Jenkins, such as EC2 instances, security groups, IAM roles, and networking components. Terraform's AWS provider simplifies the provisioning process by abstracting away the complexity of interacting with AWS APIs.
Jenkins Installation and Configuration: Utilize Terraform's provisioners or external configuration management tools like Ansible or Chef to automate the installation and initial configuration of Jenkins on the provisioned EC2 instance. This includes installing Java, downloading and configuring Jenkins, and setting up necessary plugins and credentials.
Integration with AWS Services: Leverage Jenkins plugins or AWS SDKs to interact with various AWS services as part of your CI/CD pipelines. For example, you can use the AWS CLI plugin to deploy applications to Elastic Beanstalk or Lambda functions, or integrate with AWS CodeCommit, CodeBuild, and CodeDeploy for end-to-end automation.
Pipeline as Code: Define your CI/CD pipelines using Jenkins Pipeline, which allows you to define your build, test, and deployment stages as code. This approach enables version control, code review, and collaboration on pipeline definitions, ensuring reproducibility and consistency across environments.
Benefits of Jenkins on AWS with Terraform
- Scalability: AWS's elastic infrastructure allows Jenkins to scale up or down based on workload demands, ensuring optimal performance and cost efficiency.
- Reliability: AWS's redundant infrastructure and built-in services like Auto Scaling and Elastic Load Balancing enhance the reliability and availability of Jenkins deployments.
- Automation: Terraform enables the automation of infrastructure provisioning and configuration, reducing manual intervention and ensuring consistency across environments.
- Flexibility: Jenkins' extensibility and Terraform's flexibility allow teams to tailor their CI/CD pipelines and infrastructure to meet specific requirements and integrate with a wide range of tools and services.
Let walk through an example of using Jenkins with AWS and terraform
Prerequisites
Before getting started, ensure you have the following prerequisites:
- An AWS account with appropriate permissions to create resources.
- Terraform installed on your local machine.
- Basic understanding of AWS services and Terraform.
Step 1: Define Your Infrastructure
# main.tf
provider "aws" {
region = "us-east-1"
}
resource "aws_instance" "jenkins" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "JenkinsInstance"
}
}
Step 2: Provision Jenkins Infrastructure
Run the following commands to provision the infrastructure defined in your Terraform configuration:
terraform init
terraform apply
Step 3: Install and Configure Jenkins
Connect to the Jenkins EC2 instance using SSH:
ssh -i <your-key.pem> ec2-user@<instance-public-ip>
Install Java and Jenkins on the instance following the Jenkins installation guide:
sudo yum install java-1.8.0
sudo wget -O /etc/yum.repos.d/jenkins.repo https://pkg.jenkins.io/redhat-stable/jenkins.repo
sudo rpm --import https://pkg.jenkins.io/redhat-stable/jenkins.io.key
sudo yum install jenkins
sudo systemctl start jenkins
sudo systemctl enable jenkins
Step 4: Integrate with AWS Services
For integrating with AWS services, let's assume we want to deploy applications to an S3 bucket. Install the AWS CLI and configure it with credentials:
sudo yum install aws-cli
aws configure
Then, configure a Jenkins job to deploy to S3 using the AWS CLI:
aws s3 sync /path/to/local/files s3://<your-bucket>
Step 5: Define CI/CD Pipelines
Define your CI/CD pipelines using Jenkins Pipeline. Here's an example Jenkinsfile for a simple build and deployment pipeline:
pipeline {
agent any
stages {
stage('Build') {
steps {
// Build your application here
sh 'mvn clean package'
}
}
stage('Test') {
steps {
// Run tests here
sh 'mvn test'
}
}
stage('Deploy') {
steps {
// Deploy to S3 bucket
sh 'aws s3 sync /path/to/local/files s3://<your-bucket>'
}
}
}
}
Step 6: Test and Validate
Trigger the Jenkins pipeline and monitor the build and deployment process. Ensure that the pipeline executes as expected and that the deployment to AWS services is successful.
Step 7: Monitor and Iterate
Monitor the performance of your CI/CD pipelines and Jenkins infrastructure using AWS CloudWatch and Jenkins monitoring plugins. Iterate on your pipelines and infrastructure configurations based on feedback and performance insights.
By following these steps and using the provided code examples, you can set up Jenkins on AWS using Terraform and establish efficient CI/CD pipelines tailored to your development workflow. Automating infrastructure provisioning, configuration, and deployment with Terraform and integrating with AWS services enables teams to accelerate software delivery while ensuring reliability and consistency throughout the process.
266 views