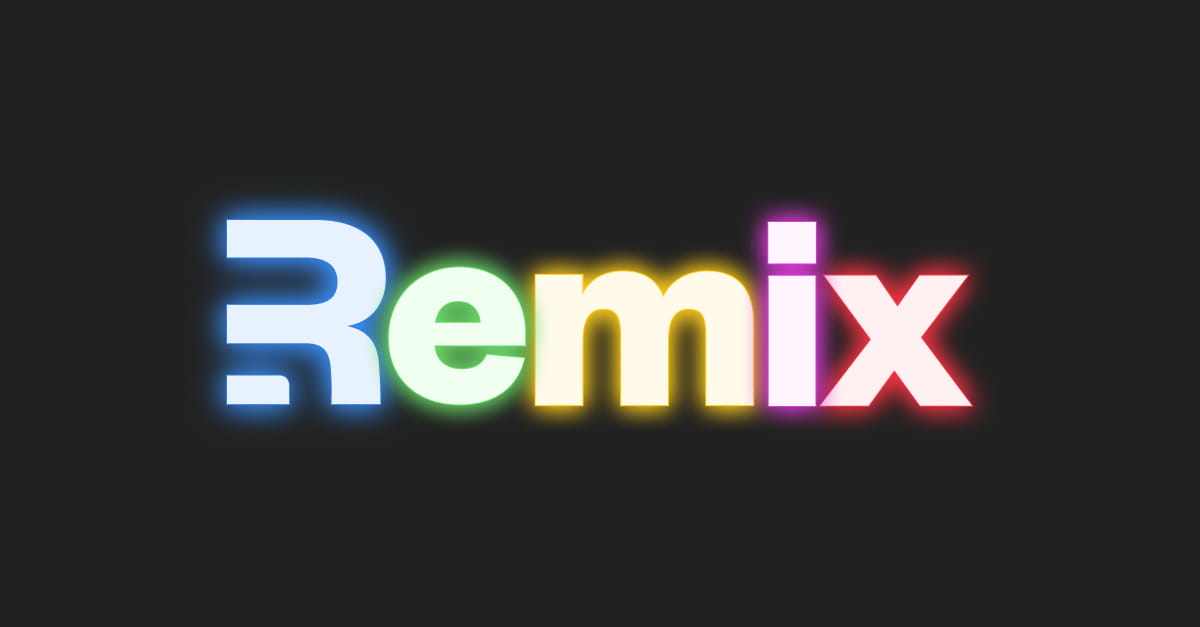
April 12, 2024
Simplify Your Signup Process with Remix-Run
Are you tired of complex signup processes that confuse your users? Do you want to streamline the user onboarding experience on your platform? Look no further than Remix-Run! In this blog post, we'll explore how you can leverage Remix-Run to create a smooth and efficient signup flow for your application.
Remix-Run is a powerful framework for building web applications with React. It offers a robust set of tools and conventions to help you create delightful user experiences. One of its key features is the ability to handle server-side rendering (SSR) and client-side rendering (CSR) seamlessly, providing fast loading times and improved SEO.
Today, we'll focus on implementing a signup page using Remix-Run. We'll cover everything from form handling to server-side validation, ensuring that your signup process is both user-friendly and secure.
Getting Started
Before diving into the code, make sure you have Remix-Run set up in your project. If you haven't already, you can install Remix-Run by following the official documentation.
Building the Signup Page
Our signup page consists of a form where users can enter their personal information, such as first name, last name, email, password, and business name. We'll use Remix-Run's Form component along with input fields to capture this information.
// Signup.tsx
import { Form, Link, useActionData, useNavigation } from '@remix-run/react';
import { useEffect, useRef } from 'react';
import { toast } from 'sonner';
import Input from '~/components/shared/Input';
import FormLabel from '~/components/shared/FormLabel';
import { action } from './signup';
export default function Signup() {
// Hooks for handling form submission and navigation
const actionData = useActionData<typeof action>();
const navigation = useNavigation();
const formRef = useRef<HTMLFormElement | null>(null);
// Effect to display toast messages based on form submission result
useEffect(() => {
if (actionData?.errors?.response) {
toast.error('An error occurred', {
description: actionData?.errors?.response,
});
}
if (actionData?.success?.response) {
if (formRef.current) formRef.current.reset();
toast.success('Successful', {
description: actionData?.success?.response,
});
}
}, [actionData]);
return (
<>
<h1 className="mt-10 text-center text-3xl font-bold lg:text-4xl">
Signup
</h1>
<Form ref={formRef} method="post" className="mx-auto mt-5 lg:mt-8">
{/* Form fields */}
</Form>
</>
);
}
Handling Form Submission
Next, let's implement the form submission logic. We'll define an action function that will be responsible for processing the form data, validating it, and handling any errors.
// signup.ts
import { ActionFunctionArgs, json } from '@remix-run/node';
import { getSession } from '~/sessions';
import { backendUrl } from '~/constants/backendUrls';
import http from '~/lib/http';
import type { ISignupResponse } from '~/types/auth';
export async function action({ request }: ActionFunctionArgs) {
// Form validation and submission logic
}
Server-Side Validation and API Interaction
Inside the action function, we'll validate the form data and make an API request to register the user. We'll also handle any errors that occur during this process.
// signup.ts
export async function action({ request }: ActionFunctionArgs) {
// Extract form data and session information
const formData = await request.formData();
const email = String(formData.get('email'));
const password = String(formData.get('password'));
const accountName = String(formData.get('bussinessName'));
const session = await getSession(request.headers.get('Cookie'));
// Form field validation
const errors: Record<string, string> = {};
const fields = ['firstName', 'lastName', 'email', 'password', 'businessName'];
fields.forEach((field) => {
const value = formData.get(field);
if (!value) {
errors[field] = 'Please provide a valid value';
}
});
// If no validation errors, attempt user registration
if (!Object.keys(errors).length) {
try {
const response = await http.post(backendUrl.signup, {
email,
password,
accountName,
});
const data = response.data as ISignupResponse;
// Store user session information
session.set('accessToken', data.response.accessToken);
session.set('email', data.response.email);
// Success message
const success: Record<string, string> = {};
success['response'] =
'Successfully registered... Redirecting you to the home page';
// Return success response
return json({ success }, {
headers: {
'Set-Cookie': await commitSession(session),
},
});
} catch (error) {
// Error message for invalid credentials
errors['response'] = 'Incorrect credentials';
}
}
// Return error response if validation fails
return json({ errors }, {
headers: {
'Set-Cookie': await commitSession(session),
},
});
}
Conclusion
With Remix-Run, creating a signup page that is both functional and user-friendly is a breeze. By leveraging its powerful features and intuitive API, you can build delightful experiences for your users while maintaining code simplicity and efficiency.
In this blog post, we covered the basics of creating a signup page using Remix-Run, including form handling, server-side validation, and API interaction. We hope this serves as a useful guide to help you simplify your signup process and enhance your application's user experience. Happy coding!
If you have any questions or feedback, feel free to leave a comment below. And don't forget to check out the Remix-Run documentation for more advanced features and best practices. Until next time, happy coding!
490 views