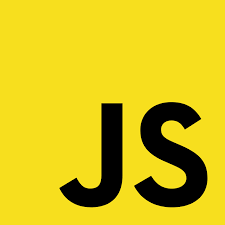
April 14, 2024
Mastering State Management in Vanilla JavaScript: A Comprehensive Guide
In the world of web development, managing the state of your application is crucial for creating dynamic and interactive user experiences. Whether you're building a simple web page or a complex single-page application (SPA), effective state management can make or break your project. While there are many libraries and frameworks available to handle state management, it's essential to understand the fundamentals in pure JavaScript. In this guide, we'll delve into mastering state management in vanilla JavaScript, providing clear explanations and practical code examples along the way.
Understanding State Management
Before diving into the code, let's clarify what we mean by "state" in the context of web development. The state of an application refers to any data that can change over time, influenced by user interaction, network requests, or other factors. State management involves handling and updating this data in a predictable and efficient manner.
Basic Techniques for State Management
1. Using Variables
The most straightforward way to manage state in JavaScript is by using variables. For instance, consider a simple counter application:
let count = 0;
function increment() {
count++;
render();
}
function decrement() {
count--;
render();
}
function render() {
document.getElementById('counter').innerText = count;
}
In this example, the count variable holds the current state of the counter, and the increment and decrement functions modify this state accordingly.
2. Object State
For more complex applications, you can use objects to organize and manage state:
let state = {
count: 0,
message: "Hello, World!"
};
function updateCount(newCount) {
state.count = newCount;
render();
}
function updateMessage(newMessage) {
state.message = newMessage;
render();
}
function render() {
document.getElementById('counter').innerText = state.count;
document.getElementById('message').innerText = state.message;
}
In this example, the state object encapsulates both the counter value and a message. Functions like updateCount and updateMessage modify specific properties of the state object, followed by a render function to update the UI accordingly.
Challenges with Vanilla JavaScript State Management
While these basic techniques suffice for small-scale applications, they become cumbersome and error-prone as your project grows. Some challenges you might encounter include:
- Global Scope Pollution: Managing state with global variables or objects can lead to namespace collisions and unintended side effects.
- Complexity: As your application grows, managing state across multiple components becomes increasingly complex and difficult to maintain.
- Imperative Updates: Directly mutating state can make it challenging to track changes and debug issues, especially in asynchronous scenarios.
Advanced State Management Techniques
To address these challenges, you can employ more advanced techniques or design patterns:
1. Observer Pattern
Implementing the observer pattern allows components to subscribe to state changes and react accordingly. This decouples components from each other and promotes a more modular and maintainable architecture.
2. Functional Programming Principles
Leverage functional programming principles such as immutability and pure functions to ensure predictable state management and facilitate debugging and testing.
3. State Containers
Organize your state into containers or stores, separating concerns and providing a centralized location for state management. This approach is commonly used in Flux and Redux architectures.
Conclusion
Mastering state management in vanilla JavaScript is essential for building robust and maintainable web applications. By understanding the fundamental principles and employing advanced techniques and design patterns, you can create scalable and efficient state management solutions tailored to your project's needs. While libraries and frameworks can provide valuable tools and abstractions, a solid grasp of vanilla JavaScript state management is invaluable for any web developer's toolkit. Experiment with different approaches, iterate on your designs, and strive for simplicity and clarity in your code. Happy coding!
980 views