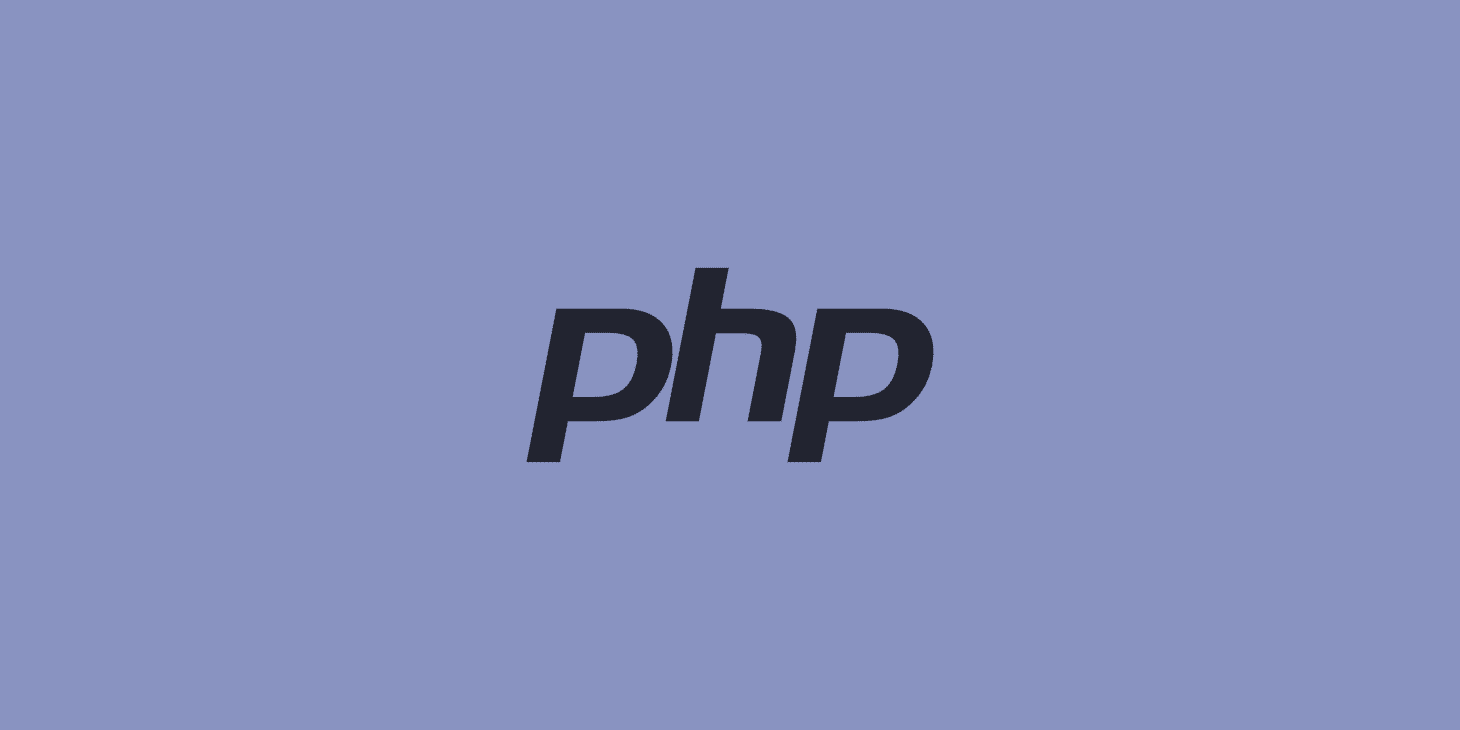
In object-oriented programming (OOP), inheritance is a powerful concept that allows classes to inherit properties and methods from other classes. PHP, being an object-oriented language, supports inheritance extensively. Constructors, which are special methods used for initializing objects, play a crucial role in inheritance. In this article, we'll delve into how constructors work in the context of inheritance in PHP.
What are Constructors?
Before diving into constructors in inheritance, let's first understand what constructors are. In PHP, a constructor is a special method within a class that gets called automatically when an object of that class is instantiated. It typically initializes the object's properties or performs any necessary setup tasks.
Here's a basic example of a constructor in PHP:
class MyClass {
public function __construct() {
echo "Constructor called!";
}
}
$obj = new MyClass(); // Output: Constructor called!
In this example, the __construct() method is the constructor, and it echoes "Constructor called!" when an object of the MyClass class is created.
Constructors in Inheritance
When dealing with inheritance in PHP, constructors behave differently compared to regular methods. Inheritance allows a subclass (child class) to inherit properties and methods from a superclass (parent class). But what about constructors?
Implicit Constructor Inheritance
In PHP, constructors are not inherited by default. However, if a subclass does not define its own constructor, it will implicitly inherit the constructor of its parent class. This means that when an object of a subclass is instantiated, PHP will look for a constructor in the subclass first. If it's not found, PHP will search for and execute the constructor of the parent class.
Here's an example to illustrate this:
class ParentClass {
public function __construct() {
echo "Parent constructor called!";
}
}
class ChildClass extends ParentClass {
}
$obj = new ChildClass(); // Output: Parent constructor called!
In this example, the ChildClass does not have its own constructor. Therefore, when an object of ChildClass is created, PHP implicitly calls the constructor of the ParentClass.
Overriding Constructors
Subclasses can override constructors defined in their parent classes by providing their own constructor with the same name. This allows subclasses to customize the initialization process while still benefiting from the inheritance mechanism.
class ParentClass {
public function __construct() {
echo "Parent constructor called!";
}
}
class ChildClass extends ParentClass {
public function __construct() {
echo "Child constructor called!";
}
}
$obj = new ChildClass(); // Output: Child constructor called!
In this example, the ChildClass defines its own constructor, which overrides the constructor of the ParentClass. When an object of ChildClass is instantiated, PHP calls the constructor defined in ChildClass.
Calling Parent Constructors
Sometimes, when overriding constructors in subclasses, you might want to call the constructor of the parent class to ensure that any initialization tasks defined in the parent constructor are executed. In PHP, you can do this using the parent::__construct() syntax within the subclass constructor.
class ParentClass {
public function __construct() {
echo "Parent constructor called!";
}
}
class ChildClass extends ParentClass {
public function __construct() {
parent::__construct(); // Calling parent constructor
echo "Child constructor called!";
}
}
$obj = new ChildClass(); // Output: Parent constructor called! Child constructor called!
In this example, the ChildClass constructor calls the constructor of the ParentClass using parent::__construct(). This ensures that both the parent and child initialization tasks are performed.
Conclusion
Constructors are essential in object-oriented programming for initializing objects. In PHP, constructors play a crucial role in inheritance, allowing subclasses to inherit and customize the initialization process defined in their parent classes. Understanding how constructors behave in the context of inheritance is fundamental for building robust and maintainable object-oriented PHP applications.
616 views