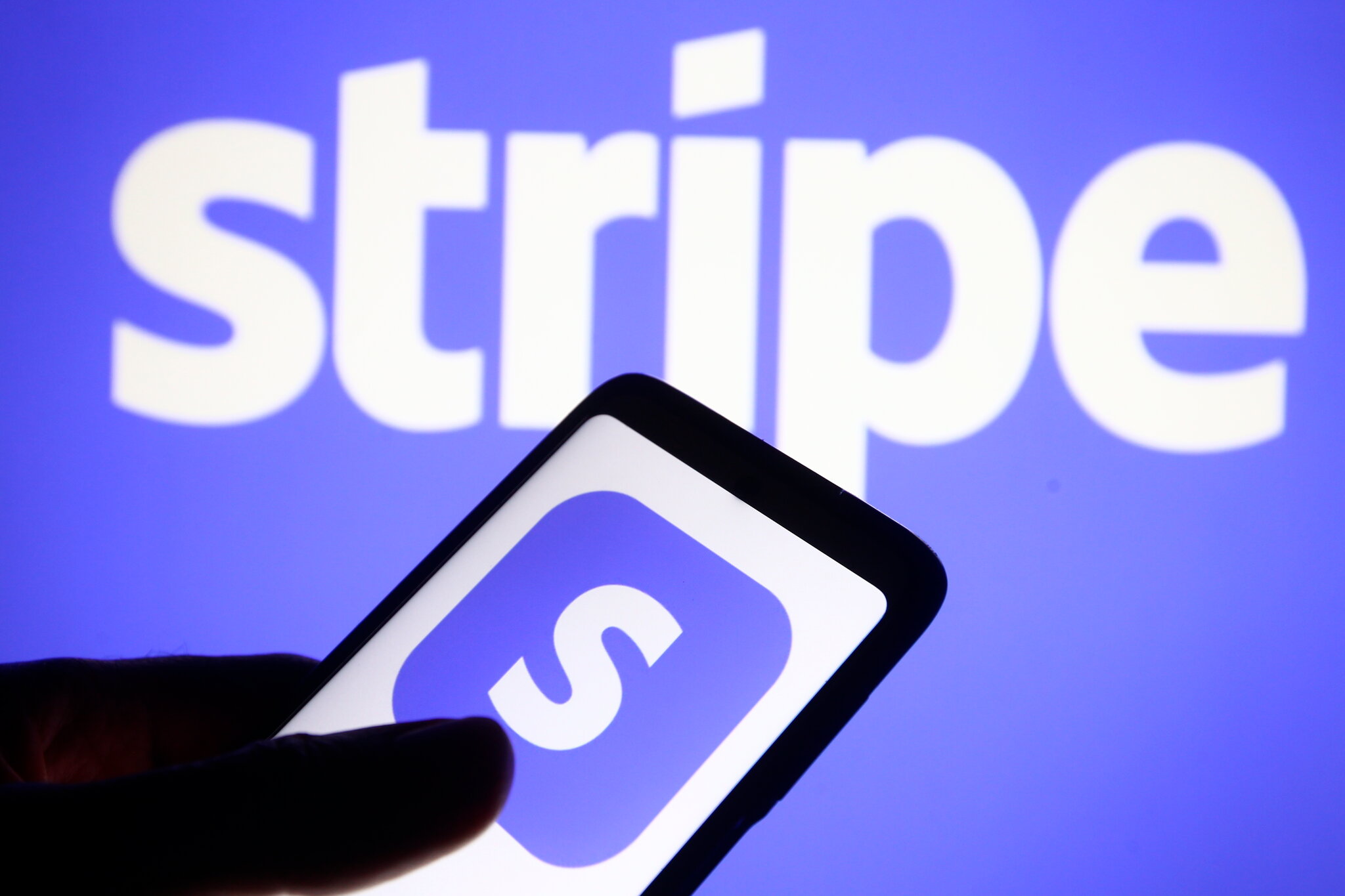
April 16, 2024
Simplifying Payment Processing with Stripe and Node.js
In today's digital age, businesses of all sizes are constantly seeking ways to streamline their payment processes while ensuring security and reliability. One solution that has gained significant popularity is Stripeââ¬âa powerful platform that simplifies online transactions. Coupled with the flexibility and efficiency of Node.js, developers can create seamless payment experiences for their users. In this blog post, we'll explore how to integrate Stripe with Node.js to accept payments effortlessly.
Understanding Stripe:
Stripe is a leading payment gateway that enables businesses to accept online payments securely. It provides a comprehensive set of APIs and tools that handle payment processing, subscription management, fraud prevention, and more. With Stripe, businesses can accept various payment methods, including credit cards, digital wallets, and bank transfers, making it versatile for a wide range of industries and use cases.
Setting Up Your Stripe Account:
Before integrating Stripe into your Node.js application, you'll need to sign up for a Stripe account if you haven't already done so. The process is straightforward and typically involves providing some basic information about your business, such as your company name, website URL, and banking details. Once your account is set up, you'll gain access to the Stripe Dashboard, where you can manage your payment settings, view transactions, and generate API keys.
Installing the Stripe Node.js Library:
To start accepting payments with Stripe in your Node.js application, you'll need to install the Stripe Node.js library. You can do this using npm, the package manager for Node.js, by running the following command in your terminal:
npm install stripe
This command will download and install the latest version of the Stripe library, allowing you to interact with the Stripe API seamlessly from your Node.js code.
Integrating Stripe into Your Node.js Application:
Once you have the Stripe library installed, you can begin integrating Stripe into your Node.js application. Here's a simple example of how to set up a basic payment flow using Node.js and Express:
Require the Stripe library and initialize it with your API keys:
const stripe = require('stripe')('YOUR_STRIPE_SECRET_KEY');
Set up an Express route to handle payment requests:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
app.post('/charge', async (req, res) => {
try {
const { amount, currency, source, description } = req.body;
const paymentIntent = await stripe.paymentIntents.create({
amount,
currency,
source,
description,
});
res.json({ client_secret: paymentIntent.client_secret });
} catch (error) {
res.status(500).json({ error: error.message });
}
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Make a POST request to this route from your client-side code with the required payment details:
fetch('/charge', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
amount: 1000, // amount in cents
currency: 'usd',
source: 'tok_visa', // replace with your test card token
description: 'Test payment',
}),
})
.then(response => response.json())
.then(data => {
// Use data.client_secret to complete the payment on the client side
console.log('Payment successful');
})
.catch(error => {
console.error('Error:', error);
});
Handle the payment confirmation on the client side using Stripe.js:
<script src="https://js.stripe.com/v3/"></script>
<script>
var stripe = Stripe('YOUR_STRIPE_PUBLIC_KEY');
// Complete the payment using the client_secret returned from the server
stripe.confirmCardPayment(clientSecret, {
payment_method: {
card: cardElement,
billing_details: {
name: 'John Doe',
},
},
}).then(function(result) {
if (result.error) {
// Handle errors
console.error(result.error.message);
} else {
// Payment successful
console.log(result.paymentIntent);
}
});
</script>
Conclusion:
Integrating Stripe with Node.js opens up a world of possibilities for businesses looking to streamline their payment processes. With its powerful features and robust API, Stripe makes it easy to accept payments securely across various channels. By following the steps outlined in this blog post, you can quickly set up a seamless payment flow in your Node.js application, empowering your business to succeed in the digital marketplace.
Whether you're selling products online, managing subscriptions, or collecting donations, Stripe's versatility and reliability make it an ideal choice for handling your payment needs. So why wait? Start accepting payments with Stripe and Node.js today and take your business to new heights of success.
564 views