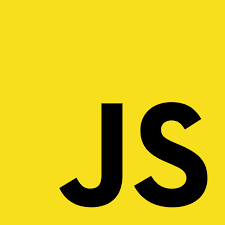
August 16, 2024
Mastering JavaScript Performance Optimization: Proven Tips and Best Practices
In today's fast-paced web development landscape, optimizing JavaScript performance is paramount for delivering seamless user experiences. As websites become increasingly complex, ensuring that your JavaScript code runs efficiently is crucial. In this blog post, we'll explore some essential tips and best practices for optimizing JavaScript performance, along with code examples to illustrate each concept.
Minimize DOM Manipulation:
One of the most significant factors affecting JavaScript performance is DOM manipulation. Excessive DOM manipulation can lead to rendering bottlenecks and decreased performance. To optimize performance, minimize DOM manipulation by:
// Inefficient DOM manipulation
for (let i = 0; i < 1000; i++) {
document.getElementById('container').innerHTML += '<div>' + i + '</div>';
}
// Efficient DOM manipulation
const container = document.getElementById('container');
const fragment = document.createDocumentFragment();
for (let i = 0; i < 1000; i++) {
const div = document.createElement('div');
div.textContent = i;
fragment.appendChild(div);
}
container.appendChild(fragment);
Use Event Delegation:
Event delegation involves attaching a single event listener to a parent element rather than individual child elements. This technique reduces the number of event listeners, resulting in better performance, especially for dynamically generated content.
// Without event delegation
const buttons = document.querySelectorAll('.button');
buttons.forEach(button => {
button.addEventListener('click', () => {
// Handle click event
});
});
// With event delegation
const container = document.getElementById('container');
container.addEventListener('click', event => {
if (event.target.classList.contains('button')) {
// Handle click event
}
});
Optimize Loops:
Loop optimization is crucial for improving JavaScript performance, especially when dealing with large datasets. Consider using methods like forEach, map, filter, and reduce instead of traditional loops.
// Traditional loop
const numbers = [1, 2, 3, 4, 5];
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
// Using Array.reduce
const sum = numbers.reduce((acc, curr) => acc + curr, 0);
Implement Debouncing and Throttling:
Debouncing and throttling are techniques used to optimize event handling functions, especially for events like scrolling and resizing. Debouncing ensures that a function is only called after a specified delay, while throttling limits the number of times a function can be called over a certain period.
// Debouncing
function debounce(func, delay) {
let timeoutId;
return function(...args) {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => {
func.apply(this, args);
}, delay);
};
}
const debouncedFn = debounce(() => {
// Do something after delay
}, 300);
window.addEventListener('scroll', debouncedFn);
// Throttling
function throttle(func, limit) {
let throttled = false;
return function(...args) {
if (!throttled) {
func.apply(this, args);
throttled = true;
setTimeout(() => {
throttled = false;
}, limit);
}
};
}
const throttledFn = throttle(() => {
// Do something at most once every 300ms
}, 300);
window.addEventListener('scroll', throttledFn);
Optimize Network Requests:
Reducing the number of HTTP requests and optimizing the size of resources can significantly improve JavaScript performance. Techniques like code splitting, lazy loading, and caching can help optimize network requests.
// Code splitting
import('./module').then(module => {
// Use module
});
// Lazy loading
const button = document.getElementById('button');
button.addEventListener('click', () => {
import('./module').then(module => {
// Use module
});
});
// Caching
const cache = {};
function fetchData(url) {
if (cache[url]) {
return Promise.resolve(cache[url]);
}
return fetch(url)
.then(response => response.json())
.then(data => {
cache[url] = data;
return data;
});
}
Conclusion:
Optimizing JavaScript performance is essential for delivering fast and responsive web experiences. By following these tips and best practices, you can significantly improve the performance of your JavaScript code. Remember to always profile and measure the performance impact of your optimizations to ensure effectiveness. With a well-optimized JavaScript codebase, you can provide users with a smoother and more enjoyable browsing experience.
607 views