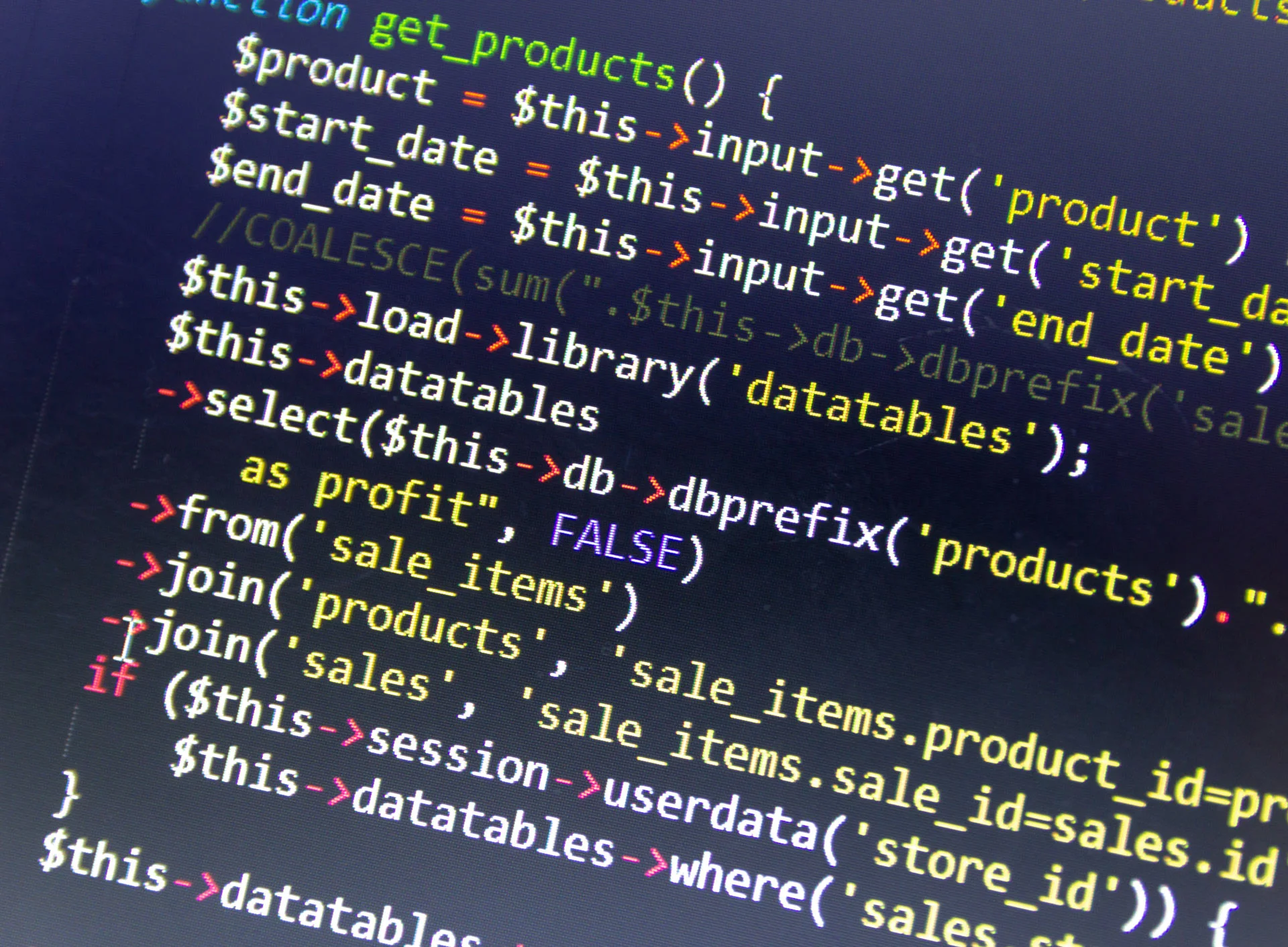
PHP (Hypertext Preprocessor) is a widely-used open-source scripting language that is especially suited for web development and can be embedded into HTML. It powers a significant portion of the internet, from small websites to large-scale applications. However, like any programming language, PHP performance can be optimized to enhance the speed and efficiency of your web applications. In this article, we will explore some tips and best practices for optimizing PHP performance, along with code examples.
1. Use Opcode Caching:
One of the most effective ways to improve PHP performance is by enabling opcode caching. Opcode caching stores compiled PHP code in memory, reducing the overhead of compiling the code on every request. Popular opcode caching solutions include APC (Alternative PHP Cache), OPcache, and XCache. Here's an example of enabling OPcache in your PHP configuration file:
opcache.enable=1
opcache.enable_cli=1
2. Minimize File Includes:
Minimize the number of file includes in your PHP code, as each include statement incurs a performance overhead. Instead, consider using autoloading for classes and consolidating code into fewer files where possible. Here's an example of autoloading classes using Composer's PSR-4 autoloader:
// In your composer.json file
{
"autoload": {
"psr-4": {"App\\": "src/"}
}
}
// In your PHP script
require 'vendor/autoload.php';
3. Optimize Database Queries:
Efficient database queries are crucial for PHP performance. Avoid using SELECT * and fetch only the columns you need. Additionally, utilize indexes appropriately to speed up query execution. Here's an example of optimizing a database query using PDO:
$stmt = $pdo->prepare("SELECT id, name FROM users WHERE status = ?");
$stmt->execute([$status]);
$users = $stmt->fetchAll();
4. Cache Data:
Cache frequently accessed data to reduce database and file system load. Use caching mechanisms like Memcached or Redis to store query results, rendered HTML, or any other data that can be reused across requests. Here's an example of caching query results using Memcached:
$memcached = new Memcached();
$memcached->addServer('localhost', 11211);
$key = 'users_' . $status;
$users = $memcached->get($key);
if (!$users) {
$stmt = $pdo->prepare("SELECT id, name FROM users WHERE status = ?");
$stmt->execute([$status]);
$users = $stmt->fetchAll();
$memcached->set($key, $users, 60); // Cache for 60 seconds
}
5. Optimize Loops and Functions:
Optimize loops and functions to reduce execution time. Avoid nested loops whenever possible and use built-in array functions like array_map, array_filter, and array_reduce for array manipulation. Here's an example of optimizing a loop using array_map:
// Without optimization
$modifiedArray = [];
foreach ($originalArray as $item) {
$modifiedArray[] = processItem($item);
}
// With optimization
$modifiedArray = array_map('processItem', $originalArray);
Conclusion:
Optimizing PHP performance is essential for ensuring fast and efficient web applications. By following these tips and best practices, you can significantly improve the speed and responsiveness of your PHP code. Remember to measure the impact of optimizations using profiling tools and continuously refine your code for optimal performance.
278 views