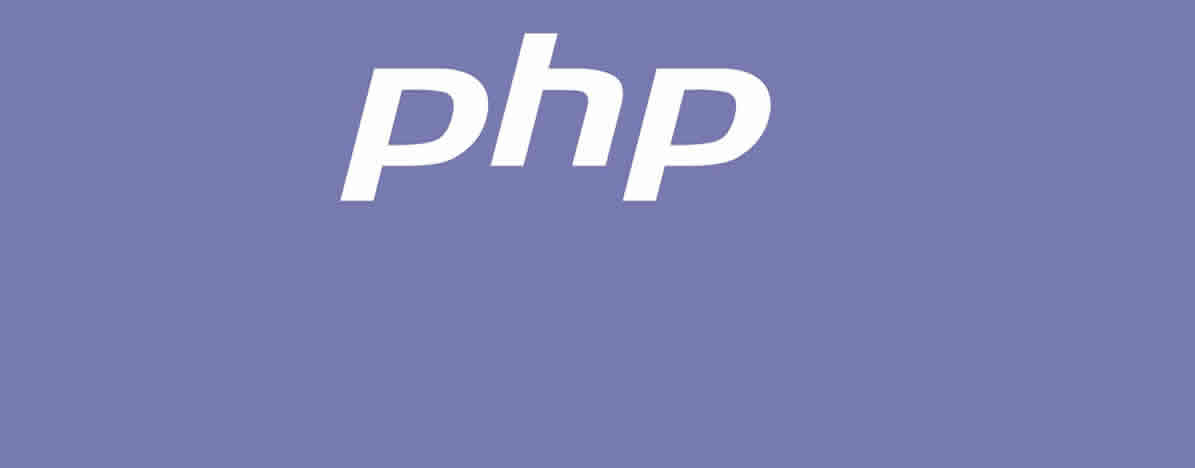
Introduction:
In PHP, interfaces play a crucial role in defining a contract for classes to implement. They provide a way to standardize the behavior of classes without enforcing specific implementations. This tutorial will delve into PHP interfaces, their usage, and how to effectively employ them in your code.
Table of Contents
- -Understanding PHP Interfaces
- -Declaring Interfaces
- -Implementing Interfaces
- -Interface Inheritance
- -Using Interfaces for Type Hinting
- -Handling Different Notification Providers: A Practical Example
- -Benefits of Interfaces
- -Conclusion
Understanding PHP Interfaces
PHP interfaces are like a blueprint for classes. They define a set of methods that a class must implement, but they do not contain any logic themselves. Instead, they specify a contract that implementing classes must follow.
Declaring Interfaces
To declare an interface in PHP, you use the interface keyword followed by the interface name. Inside the interface, you define the methods without providing any implementation details.
<?php
interface LoggerInterface {
public function log($message);
}
?>
Implementing Interfaces
To implement an interface, a class must use the implements keyword followed by the interface name. It then must provide implementations for all the methods defined in the interface.
<?php
class FileLogger implements LoggerInterface {
public function log($message) {
// Implementation to log message to a file
}
}
?>
Interface Inheritance
Interfaces can also inherit from other interfaces using the extends keyword. This allows for the extension of interfaces, providing additional methods that implementing classes must define.
<?php
interface AdvancedLoggerInterface extends LoggerInterface {
public function logError($errorMessage);
}
?>
Using Interfaces for Type Hinting
One of the primary benefits of interfaces is using them for type hinting in function parameters and return types. This ensures that only objects implementing the specified interface can be used with a particular function.
<?php
function logMessage(LoggerInterface $logger, $message) {
$logger->log($message);
}
?>
Handling Different Notification Providers: A Practical Example
Now, let's illustrate how interfaces can be used in a practical scenario. Suppose we have a messaging system that sends notifications to users via different channels, such as email and SMS. We'll define a NotificationInterface with a send method and create classes for email and SMS notifications that implement this interface.
<?php
// Define the NotificationInterface
interface NotificationInterface {
public function send($message);
}
// EmailNotification class implementing NotificationInterface
class EmailNotification implements NotificationInterface {
public function send($message) {
// Implementation to send email notification
echo "Email sent: $message\n";
}
}
// SMSNotification class implementing NotificationInterface
class SMSNotification implements NotificationInterface {
public function send($message) {
// Implementation to send SMS notification
echo "SMS sent: $message\n";
}
}
// Class with a method to send notifications
class NotificationService {
public function sendMessage(NotificationInterface $notification, $message) {
$notification->send($message);
}
}
// Example usage
$notificationService = new NotificationService();
// Sending notification via Email
$emailNotification = new EmailNotification();
$notificationService->sendMessage($emailNotification, "This is an email notification");
// Sending notification via SMS
$smsNotification = new SMSNotification();
$notificationService->sendMessage($smsNotification, "This is an SMS notification");
?>
In this example:
We define the NotificationInterface, specifying the send method.
EmailNotification and SMSNotification classes implement this interface and provide their own implementations of the send method.
The NotificationService class has a method sendMessage that accepts any object implementing the NotificationInterface and calls its send method.
We demonstrate sending notifications via both email and SMS by creating objects of EmailNotification and SMSNotification classes and passing them to the sendMessage method of NotificationService.
This approach allows us to easily switch between different notification providers (e.g., email, SMS) without modifying the sendMessage method. We can simply create a new class that implements NotificationInterface for any new notification channel we want to support, and the sendMessage method will work seamlessly with it.
Benefits of Interfaces
Code Reusability: Interfaces allow for multiple classes to implement the same set of methods, promoting code reusability.
Flexibility: They enable polymorphism, allowing objects of different classes to be treated interchangeably based on the interface they implement.
Maintainability: Interfaces facilitate better code organization and maintenance by enforcing a clear contract between classes.
Conclusion
PHP interfaces are a powerful tool for defining contracts between classes, promoting code reusability, flexibility, and maintainability. By leveraging interfaces, you can write more robust and scalable PHP applications.
This tutorial has provided a comprehensive overview of PHP interfaces, from their declaration to implementation and usage, with a practical example demonstrating their usefulness in handling different notification providers. Incorporating interfaces into your PHP projects will undoubtedly enhance code quality and structure.
555 views