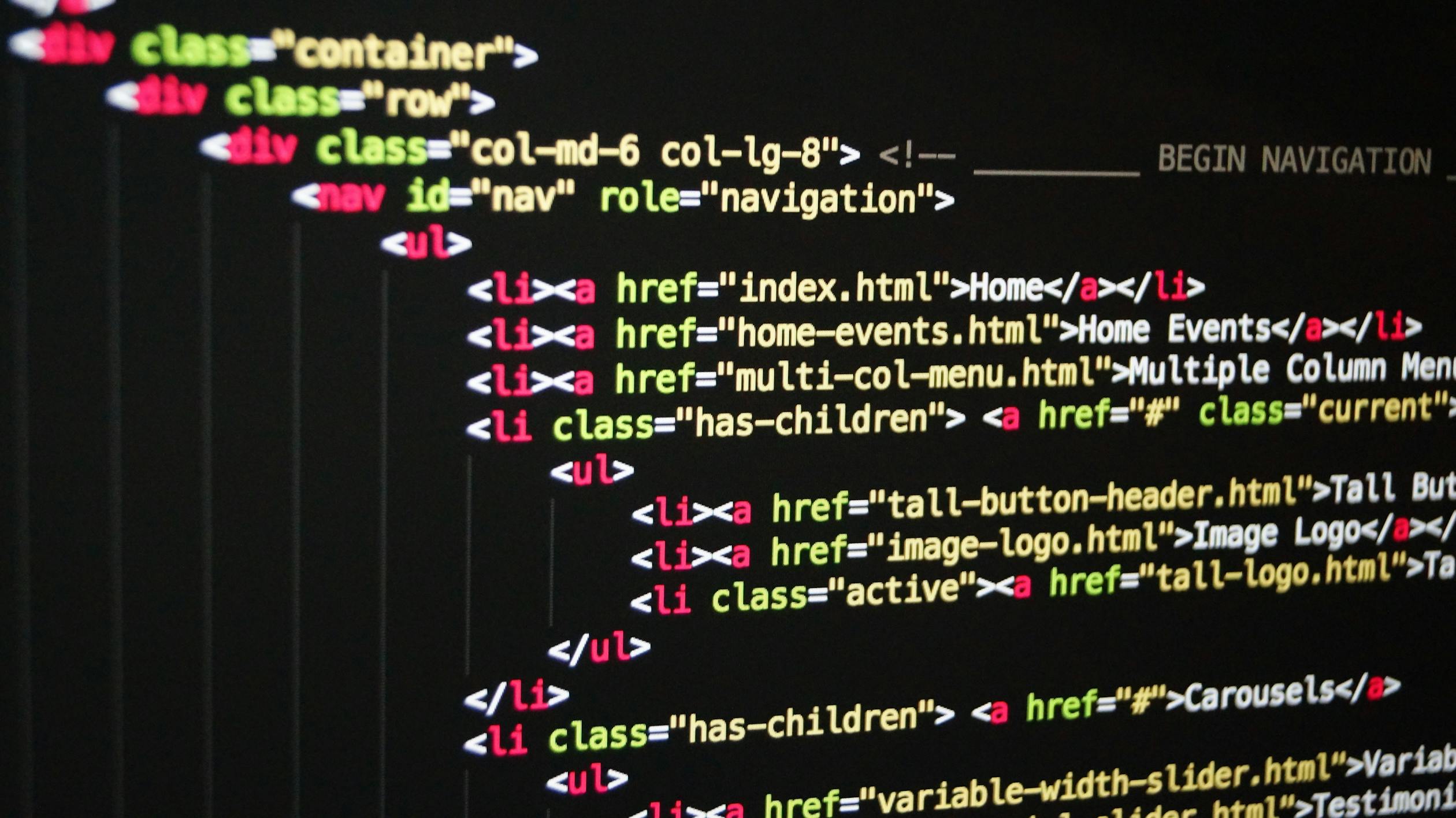
July 09, 2024
Understanding the Essence of the DRY and DIE Principles in JavaScript
In the world of software development, efficiency, readability, and maintainability are paramount. Two principles that guide developers towards achieving these goals are DRY (Don't Repeat Yourself) and DIE (Duplication is Evil). These principles, while simple in concept, carry significant weight in the coding practices of developers, ensuring cleaner, more manageable codebases. In this blog post, we'll explore the essence of these principles and how they apply specifically to JavaScript development.
DRY: Don't Repeat Yourself
The DRY principle advocates for the elimination of redundancy in code. It emphasizes the importance of reusability and encourages developers to abstract repetitive code into reusable components or functions. By adhering to DRY, developers can reduce the risk of errors, improve code readability, and streamline maintenance efforts.
In JavaScript, adhering to the DRY principle involves identifying patterns or blocks of code that occur more than once and refactoring them into reusable functions or modules. Let's consider an example:
// Without DRY
function calculateRectangleArea(width, height) {
return width * height;
}
function calculateCircleArea(radius) {
return Math.PI * radius * radius;
}
function calculateTriangleArea(base, height) {
return (base * height) / 2;
}
In the above code, there's repetition in the calculation of area for different shapes. We can refactor this code to adhere to the DRY principle:
// With DRY
function calculateArea(shape, ...args) {
switch (shape) {
case 'rectangle':
return args[0] * args[1];
case 'circle':
return Math.PI * args[0] * args[0];
case 'triangle':
return (args[0] * args[1]) / 2;
default:
return null;
}
}
By consolidating the area calculation logic into a single function, we eliminate redundancy and make the code more maintainable and flexible.
DIE: Duplication is Evil
While DRY focuses on eliminating redundancy, DIE takes a more aggressive stance against duplication in code. It suggests that any form of duplication, even small instances, should be avoided whenever possible. By minimizing duplication, developers can enhance code clarity, reduce the chance of bugs, and facilitate easier code modifications in the future.
In JavaScript, duplication can manifest in various forms, including repeated logic, similar function implementations, or redundant data structures. Let's look at an example:
// Without DIE
function applyDiscount(price, discount) {
return price * (1 - discount / 100);
}
function applyTax(price, taxRate) {
return price * (1 + taxRate / 100);
}
In the above code, both functions follow a similar pattern of applying a percentage-based calculation to a price. We can refactor this code to adhere to the DIE principle:
// With DIE
function applyPercentage(price, percentage) {
return price * percentage / 100;
}
function applyDiscount(price, discount) {
return price - applyPercentage(price, discount);
}
function applyTax(price, taxRate) {
return price + applyPercentage(price, taxRate);
}
By extracting the common logic into a separate function (applyPercentage), we eliminate duplication and improve code maintainability. Any changes to the percentage calculation logic only need to be made in one place.
Conclusion
In JavaScript development, adhering to the DRY and DIE principles is essential for writing clean, efficient, and maintainable code. By avoiding repetition and eliminating duplication, developers can produce codebases that are easier to understand, modify, and extend. While applying these principles requires careful consideration and discipline, the benefits they bring in terms of code quality and developer productivity make them indispensable guidelines in modern software development.
622 views