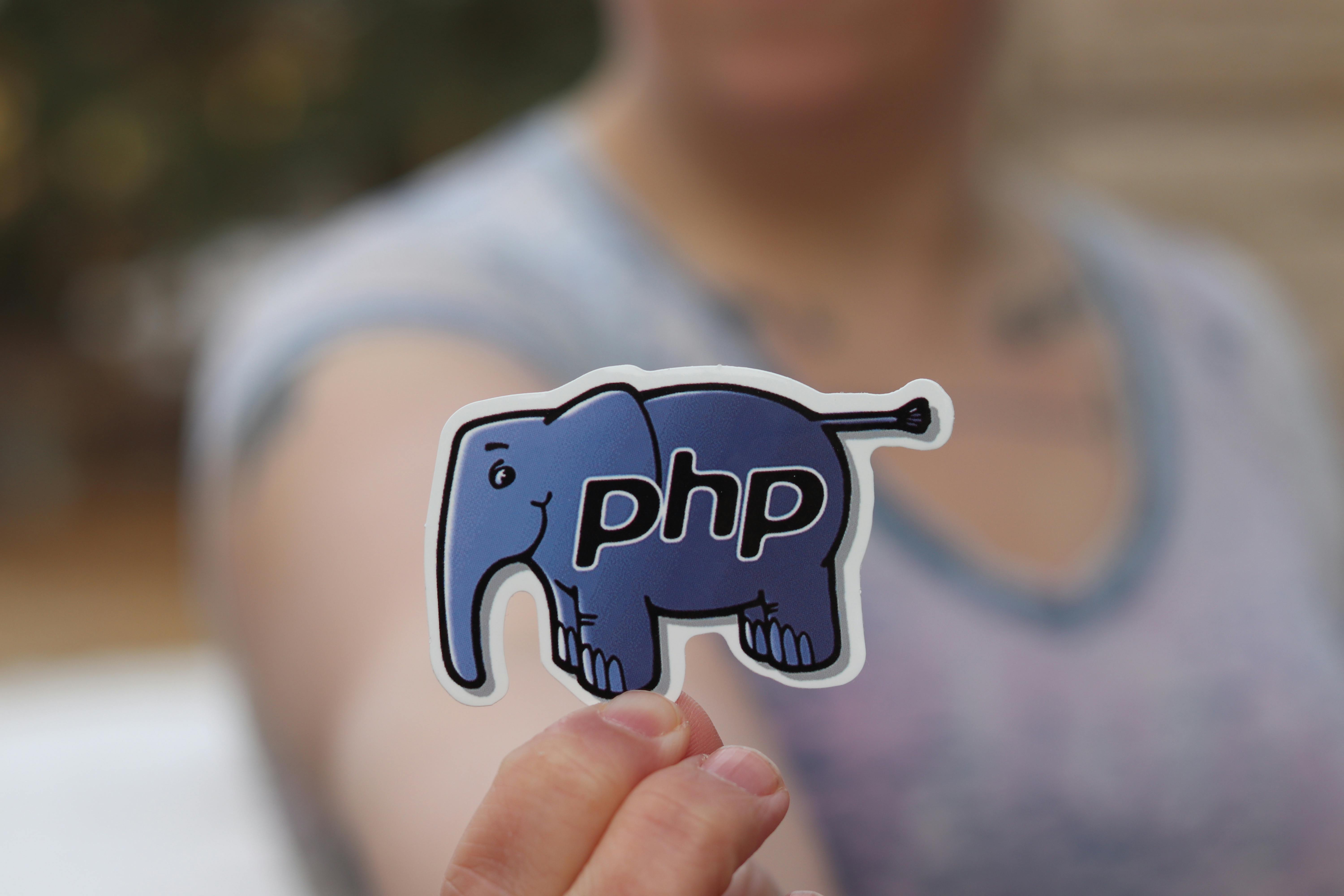
In the realm of programming, particularly in PHP, understanding coercion is crucial for writing efficient and bug-free code. Coercion, sometimes referred to as type juggling, is the process of converting a value from one data type to another implicitly by the PHP engine. While coercion can be convenient, it can also lead to unexpected behaviors and bugs if not handled carefully. In this blog post, we'll delve into coercion in PHP, explore its mechanisms, potential pitfalls, and best practices for managing it effectively.
What is Coercion?
Coercion occurs when PHP automatically converts a value from one data type to another in contexts where it's required. This conversion happens implicitly, without explicit instructions from the programmer. For instance, consider the following example:
$num = "10"; // $num is a string
$sum = $num + 5; // $sum will be 15, with $num being coerced to an integer
In this example, even though $num is a string, PHP coerces it into an integer when performing the addition operation.
Implicit Type Conversion Rules
PHP has a set of rules for determining how data types are coerced in various contexts. Some common coercion rules include:
- Strings containing numeric characters are coerced to numbers.
- Strings not containing numeric characters are coerced to zero.
- Boolean values are coerced to integers (true becomes 1, false becomes 0).
- Arrays and objects are coerced to integers (0 for empty, 1 otherwise).
- Null values are coerced to an empty string or zero depending on the context.
Understanding these rules is essential for predicting how PHP will coerce values in different scenarios.
Pitfalls of Coercion
While coercion can be convenient, it can also lead to subtle bugs and unexpected behavior if not understood properly. Consider the following example:
$value = "10 apples";
$result = $value + 5;
echo $result; // Output: 15
In this example, PHP coerces the string "10 apples" to the integer 10 and performs the addition. However, if the string had been "apples 10", PHP would coerce it to 0, leading to potentially unexpected results.
Best Practices for Handling Coercion
To avoid pitfalls associated with coercion, consider the following best practices:
Explicit Type Conversion: Instead of relying on implicit coercion, explicitly convert values to the desired data type using functions like intval(), floatval(), or strval().
Type Checking: Perform type checking using functions like is_numeric(), is_string(), or is_int() to ensure that variables are of the expected data type before performing operations.
Strict Comparison: Use strict comparison operators (=== and !==) to compare variables, which not only compare values but also their data types, thus minimizing the chance of unintended coercion.
Error Reporting: Enable error reporting in PHP to catch coercion-related warnings and notices early in the development process. This can be achieved by setting error_reporting(E_ALL) in your code or via php.ini configuration.
Conclusion
Coercion in PHP is a powerful feature that simplifies coding in many situations, but it comes with its own set of challenges. By understanding how coercion works, being aware of its pitfalls, and adhering to best practices, developers can write more robust and predictable code. Whether it's through explicit type conversion, type checking, or error reporting, taking a proactive approach to managing coercion can lead to cleaner and more maintainable PHP applications.
610 views