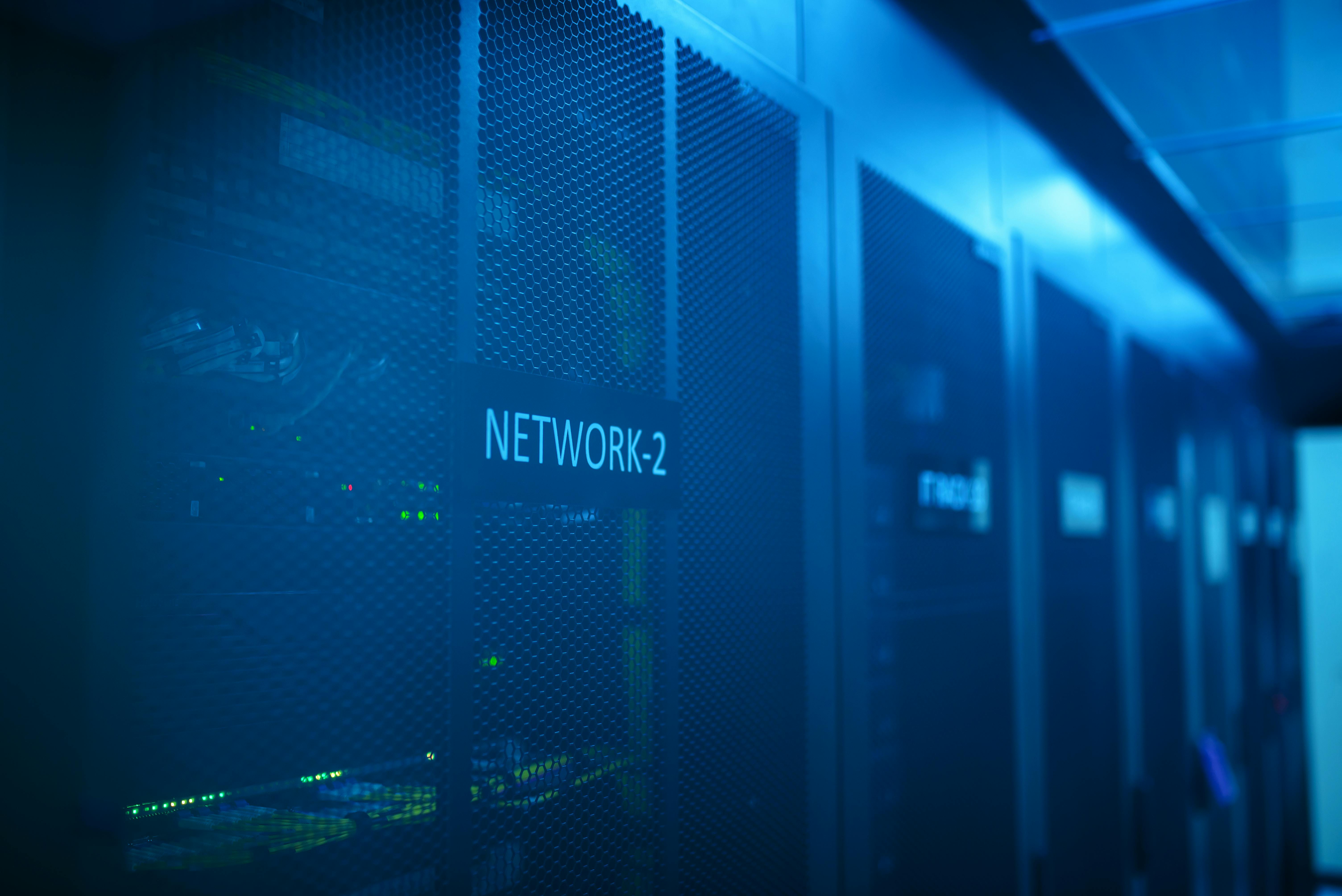
April 09, 2024
Safeguarding Your Database: Preventing SQL Injection in JavaScript
In the vast landscape of web development, securing your applicationââ¬â¢s data is paramount. One of the most prevalent threats developers face is SQL injection. SQL injection attacks occur when malicious actors exploit vulnerabilities in a web application to inject malicious SQL queries into input fields. These queries can manipulate your database, potentially exposing sensitive information or even causing data loss. However, with careful consideration and proper implementation of security measures, you can fortify your application against SQL injection attacks, even when working with JavaScript.
Understanding SQL Injection
Before delving into prevention methods, it's crucial to grasp the mechanics of SQL injection. In a typical scenario, an attacker inserts SQL code into input fields, such as login forms or search bars, aiming to manipulate the SQL query executed by the application's database. For instance, consider a login form where users enter their credentials. If the application concatenates user input directly into an SQL query without proper validation or parameterization, an attacker could input something like ' OR '1'='1'--, which could alter the query's logic to bypass authentication, granting unauthorized access.
Best Practices for SQL Injection Prevention in JavaScript
When developing applications with JavaScript, particularly those employing Node.js for server-side logic, several best practices can help thwart SQL injection attacks:
1. Parameterized Queries
Utilize parameterized queries or prepared statements when interacting with the database. Parameterization separates SQL code from user input, preventing malicious injections. Libraries like mysql or pg in Node.js offer support for parameterized queries.
const query = 'SELECT * FROM users WHERE username = ? AND password = ?';
connection.query(query, [username, password], (error, results) => {
// Handle results
});
2. Input Validation and Sanitization
Validate and sanitize user input rigorously. Employ libraries such as validator.js or frameworks like Express.js to ensure incoming data adheres to expected formats and does not contain malicious code.
const { check, validationResult } = require('express-validator');
app.post('/login', [
check('username').isAlphanumeric().trim(),
check('password').isLength({ min: 8 }).trim()
], (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
// Proceed with authentication
});
3. Principle of Least Privilege
Adhere to the principle of least privilege by restricting database user permissions. Assign only the necessary privileges required for the application to function, reducing the potential impact of a successful SQL injection attack.
4. Escaping Special Characters
Escape special characters in user input to neutralize their significance in SQL queries. Libraries like mysql provide functions for escaping special characters, mitigating the risk of injection.
const username = mysql.escape(req.body.username);
const password = mysql.escape(req.body.password);
const query = `SELECT * FROM users WHERE username = ${username} AND password = ${password}`;
5. Implement Web Application Firewall (WAF)
Deploy a Web Application Firewall (WAF) to intercept and filter out malicious HTTP traffic before it reaches your application. WAFs can detect and block SQL injection attempts in real-time, adding an extra layer of defense.
Conclusion
Securing your database from SQL injection attacks is an ongoing process that demands vigilance and proactive measures. By incorporating the aforementioned best practices into your JavaScript-based applications, you can significantly reduce the risk of SQL injection vulnerabilities. Remember, robust security practices not only protect your data but also enhance the trust and credibility of your application among users. Stay informed, stay vigilant, and stay secure.
250 views