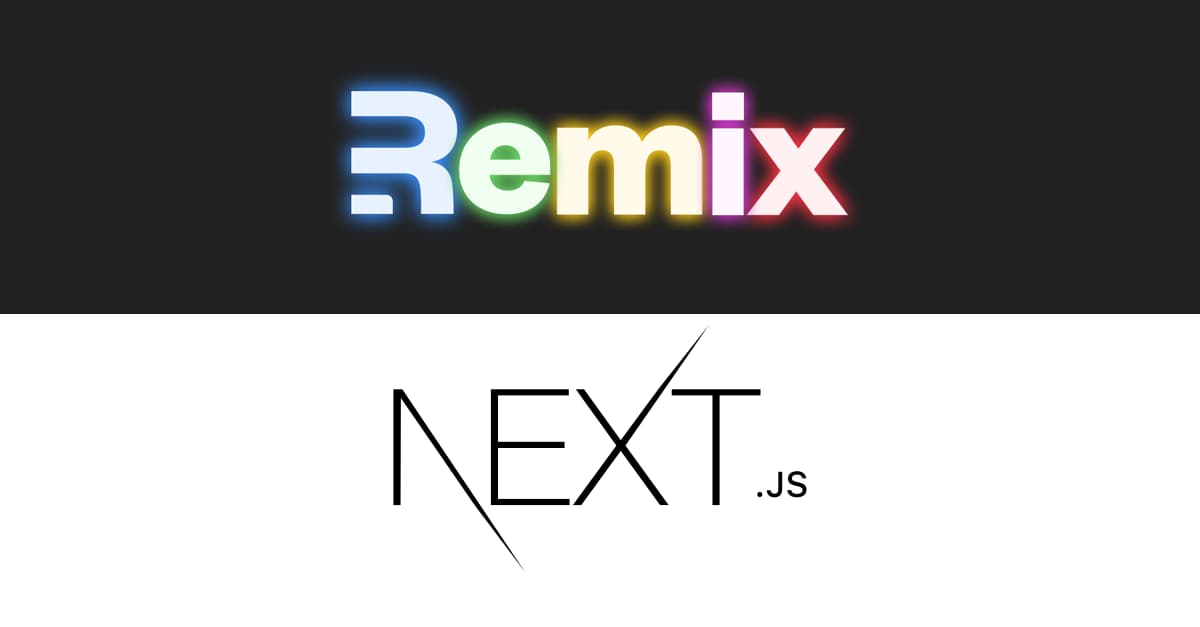
Introduction:
File uploads are a common requirement in web applications, whether you're building a content management system, a social media platform, or an e-commerce site. With frameworks like Remix and Next.js gaining popularity for their performance and developer experience, it's essential to understand how to implement file uploads seamlessly within these frameworks. In this blog post, we'll explore how to handle file uploads in Remix and Next.js, accompanied by practical code examples.
Setting Up Your Project:
Before diving into file uploads, ensure you have a basic understanding of Remix or Next.js. Both frameworks provide excellent documentation to get you started. Once you have a project set up, follow along with the steps below.
File Uploads in Remix: Remix is a powerful framework for building modern web applications. To handle file uploads in Remix, you'll need to utilize the FormData API along with the useForm hook provided by Remix.
import { json, useRouteData } from 'remix';
export async function loader({ request }) {
const formData = await request.formData();
// Handle file upload
const file = formData.get('file');
// Process the file as per your requirements
return json({ success: true });
}
export default function UploadPage() {
const onSubmit = async (event) => {
event.preventDefault();
const formData = new FormData(event.target);
await fetch('/upload', {
method: 'POST',
body: formData,
});
};
return (
<form onSubmit={onSubmit} encType="multipart/form-data">
<input type="file" name="file" />
<button type="submit">Upload</button>
</form>
);
}
File Uploads in Next.js:
Next.js, with its server-side rendering capabilities and excellent developer experience, also makes handling file uploads straightforward. You can utilize middleware like multer to handle multipart/form-data.
import multer from 'multer';
const upload = multer({ dest: 'uploads/' });
export const config = {
api: {
bodyParser: false,
},
};
export default async function handler(req, res) {
upload.single('file')(req, res, (err) => {
if (err instanceof multer.MulterError) {
// Handle multer errors
return res.status(400).json({ error: err.message });
} else if (err) {
// Handle other errors
return res.status(500).json({ error: err.message });
}
// File uploaded successfully
return res.status(200).json({ success: true });
});
}
In your React component, you can create a form similar to the one used in Remix:
export default function UploadPage() {
const onSubmit = async (event) => {
event.preventDefault();
const formData = new FormData();
formData.append('file', event.target.file.files[0]);
await fetch('/api/upload', {
method: 'POST',
body: formData,
});
};
return (
<form onSubmit={onSubmit} encType="multipart/form-data">
<input type="file" name="file" />
<button type="submit">Upload</button>
</form>
);
}
Conclusion:
Handling file uploads in Remix or Next.js is crucial for building robust web applications. Both frameworks offer efficient ways to deal with file uploads, whether you prefer server-side or client-side processing. By following the provided examples, you can seamlessly integrate file upload functionality into your Remix or Next.js projects. Feel free to explore further and tailor the implementations to your specific requirements.
613 views