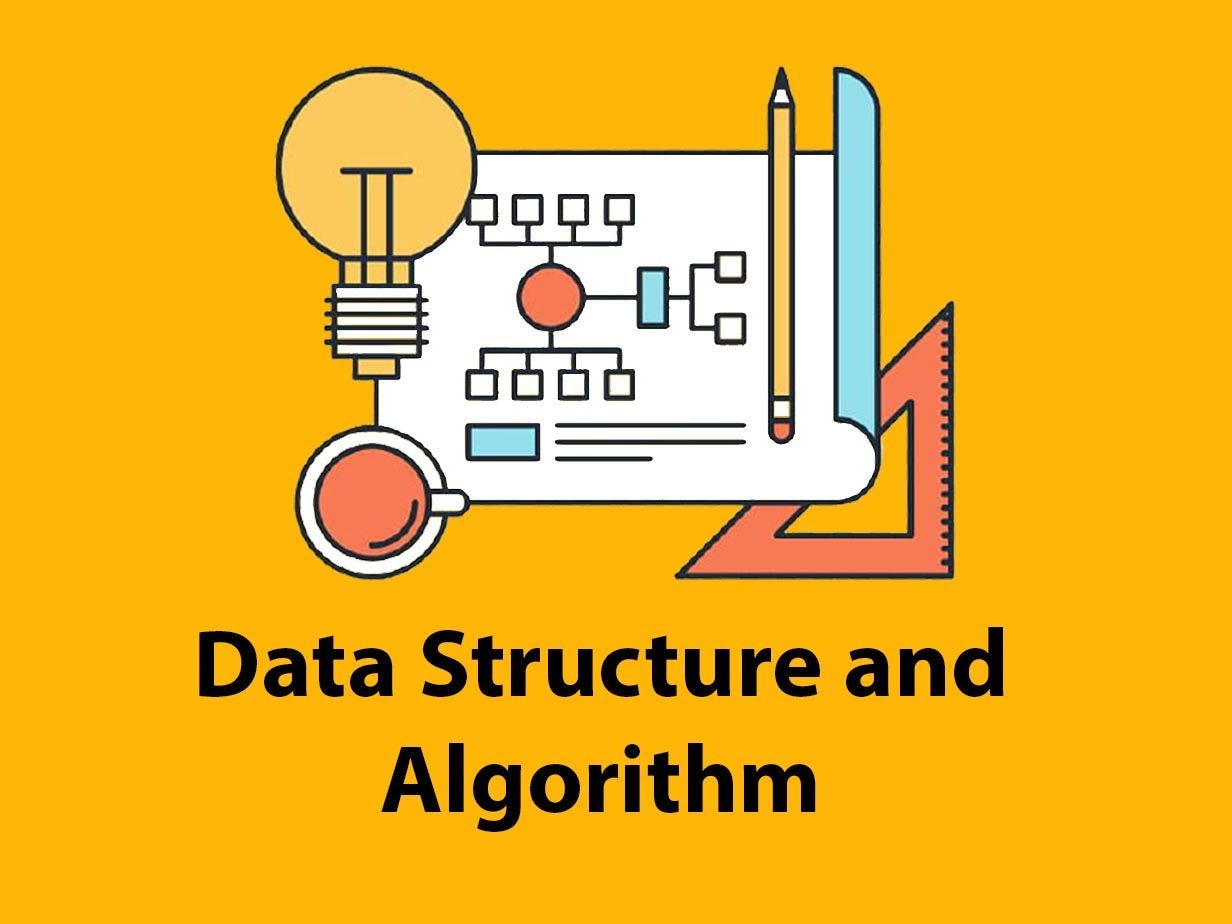
April 11, 2024
Solving Common Array Interview Questions with JavaScript #1
Array manipulation and traversal are common tasks in coding interviews, especially when dealing with algorithms and data structures. In this blog post, we'll explore and solve four popular array-related interview questions using JavaScript. These questions cover a range of topics including manipulation, pattern recognition, and algorithmic complexity.
Monotonic Array
Problem Statement: Given an array of integers, determine if it's monotonic (either entirely non-increasing or entirely non-decreasing).
Solution Approach:
- Iterate through the array and check if it's either non-increasing or non-decreasing.
- Keep track of whether the array is increasing, decreasing, or neither.
- If it's both increasing and decreasing at any point, it's not monotonic.
function isMonotonic(array) {
// Write your code here.
let isUpward = true;
let isDownward = true;
for (let i = 0; i < array.length; i++) {
if (array[i] > array[i + 1]) {
isDownward = false;
}
if (array[i] < array[i + 1]) {
isUpward = false;
}
if (!isDownward && !isUpward) {
break;
}
}
return isDownward == true || isUpward == true;
}
Longest Peak
Problem Statement: Given an array of integers, find the length of the longest peak. A peak is defined as adjacent integers in the array that are strictly increasing until they reach a peak, at which point they become strictly decreasing.
Solution Approach:
- Iterate through the array and identify peaks.
- For each peak, expand to the left and right to find the length of the peak.
- Keep track of the longest peak found.
function longestPeak(array) {
let longestPeakLength = 0;
let i = 1;
while (i < array.length - 1) {
const isPeak = array[i - 1] < array[i] && array[i] > array[i + 1];
if (!isPeak) {
i++;
continue;
}
let leftIdx = i - 2;
while (leftIdx >= 0 && array[leftIdx] < array[leftIdx + 1]) {
leftIdx--;
}
let rightIdx = i + 2;
while (rightIdx < array.length && array[rightIdx] < array[rightIdx - 1]) {
rightIdx++;
}
const currentPeakLength = rightIdx - leftIdx - 1;
longestPeakLength = Math.max(longestPeakLength, currentPeakLength);
i = rightIdx;
}
return longestPeakLength;
}
Move Element to the End
Problem Statement: Given an array of integers and an integer value, move all instances of that value to the end of the array while maintaining the relative order of the other elements.
Solution Approach:
- Iterate through the array, keeping two pointers: one to track the position to insert the target value, and one to traverse the array.
- If the current element is not the target value, swap it with the pointer position and move the pointer.
- After traversal, all instances of the target value will be at the end of the array.
function moveElementToEnd(array, toMove) {
// Write your code here.
let i = 0;
let j = array.length - 1;
while (i < j) {
if (array[i] == toMove && array[j] != toMove) {
let left = array[i];
let right = array[j];
array[j] = left;
array[i] = right;
i++;
j--;
} else if (array[j] == toMove) {
j--;
} else {
i++;
}
}
return array;
}
Spiral Traverse
Problem Statement: Given a 2D array, traverse it in a spiral order, starting from the top-left corner.
Solution Approach:
- Define four pointers to track the boundaries of the traversal: top, bottom, left, and right.
- Loop through the array while updating these pointers and traversing the array in a spiral manner.
function spiralTraverse(array) {
const spiral = [];
// Write your code here.
let startingRow = 0;
let endingRow = array.length - 1;
let startingColumn = 0;
let endingColumn = array[0].length - 1;
while (startingColumn <= endingColumn && startingRow <= endingRow) {
for (let i = startingColumn; i < endingColumn; i++) {
spiral.push(array[startingRow][i]);
}
for (let i = startingRow; i < endingRow; i++) {
spiral.push(array[i][endingColumn]);
}
for (let i = endingColumn; i > startingColumn; i--) {
spiral.push(array[endingRow][i]);
}
for (let i = endingRow; i > startingRow; i--) {
spiral.push(array[i][startingColumn]);
}
startingColumn++;
endingColumn--;
startingRow++;
endingRow--;
}
return spiral;
}
Conclusion
These array-related interview questions cover a wide range of topics and are commonly encountered in technical interviews. By understanding the problem statement and implementing efficient solutions, you'll be better prepared to tackle similar challenges. JavaScript provides a versatile and powerful platform for solving such problems, and the solutions presented here demonstrate how to approach these questions effectively. Keep practicing and exploring different problem-solving techniques to enhance your skills further.
261 views