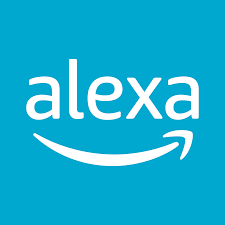
March 08, 2024
Mastering Amazon Alexa Skill Development with JavaScript: A Comprehensive Guide
Introduction:
Amazon Alexa has revolutionized the way we interact with technology, offering a voice-driven platform that enables users to perform a myriad of tasks simply by speaking. Developing skills for Amazon Alexa opens up endless possibilities for creating voice-driven experiences that range from simple utilities to complex interactive applications. In this article, we'll delve into the essential skillset required for mastering Amazon Alexa Skill Development using JavaScript, along with code examples to illustrate key concepts.
Understanding the Basics of Alexa Skills:
Before diving into skill development, it's essential to understand the fundamental components of Alexa skills:
- Invocation Name: The phrase users utter to activate the skill.
- Intents: Actions or commands users can perform within the skill.
- Slots: Variables or parameters associated with intents.
- Utterances: Phrases users speak to trigger intents.
Setting Up Your Development Environment:
To develop Alexa skills with JavaScript, you'll need:
- Node.js: JavaScript runtime for server-side development.
- ASK CLI (Alexa Skills Kit Command Line Interface): A command-line tool for managing Alexa skills.
- AWS Lambda: Serverless compute service to host the skill's backend code.
Creating Your First Alexa Skill: Let's create a simple "Hello World" skill using JavaScript.
const Alexa = require('ask-sdk-core');
const LaunchRequestHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'LaunchRequest';
},
handle(handlerInput) {
const speakOutput = 'Hello, welcome to my Alexa Skill!';
return handlerInput.responseBuilder
.speak(speakOutput)
.getResponse();
}
};
const skillBuilder = Alexa.SkillBuilders.custom();
exports.handler = skillBuilder
.addRequestHandlers(
LaunchRequestHandler
)
.lambda();
4. Handling Intents and Slots:
Let's extend our skill to handle a custom intent with slots.
const HelloWorldIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& Alexa.getIntentName(handlerInput.requestEnvelope) === 'HelloWorldIntent';
},
handle(handlerInput) {
const name = handlerInput.requestEnvelope.request.intent.slots.Name.value;
const speakOutput = `Hello, ${name}, welcome to my Alexa Skill!`;
return handlerInput.responseBuilder
.speak(speakOutput)
.getResponse();
}
};
exports.handler = skillBuilder
.addRequestHandlers(
LaunchRequestHandler,
HelloWorldIntentHandler
)
.lambda();
5. Enhancing Your Skill with DynamoDB:
Integrate DynamoDB to store and retrieve user-specific data within your skill.
const AWS = require('aws-sdk');
const docClient = new AWS.DynamoDB.DocumentClient();
const SaveNameIntentHandler = {
canHandle(handlerInput) { /* ... */ },
async handle(handlerInput) {
const name = handlerInput.requestEnvelope.request.intent.slots.Name.value;
const userId = handlerInput.requestEnvelope.session.user.userId;
await docClient.put({
TableName: 'UserData',
Item: { userId, name }
}).promise();
return handlerInput.responseBuilder
.speak(`Hello, ${name}, your name has been saved.`)
.getResponse();
}
};
Conclusion:
Mastering Amazon Alexa Skill Development with JavaScript opens up a world of possibilities for creating innovative voice-driven experiences. In this article, we've explored the essential skillset required for developing Alexa skills, along with practical code examples demonstrating key concepts. With JavaScript, Node.js, and the Alexa Skills Kit, you have all the tools you need to bring your voice-enabled ideas to life on the Amazon Alexa platform.
520 views