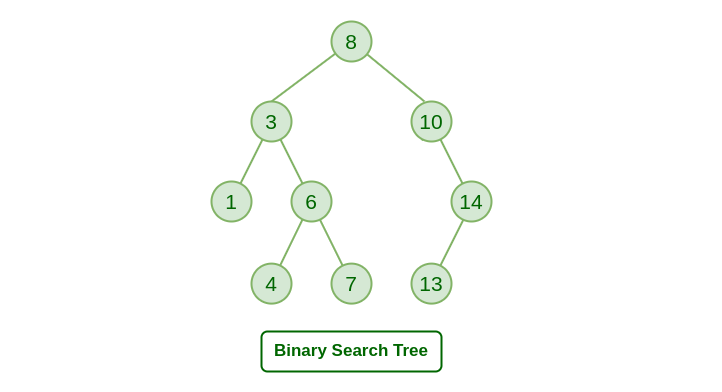
April 25, 2024
Exploring Binary Search Trees: Implementation in JavaScript
Binary search trees (BSTs) are a fundamental data structure in computer science, providing efficient insertion, deletion, and search operations. In this blog, we'll delve into the concept of binary search trees and walk through their implementation in JavaScript.
Understanding Binary Search Trees (BSTs)
A binary search tree is a hierarchical data structure consisting of nodes, where each node has at most two children: a left child and a right child. The key property of a BST is that for any given node, all nodes in its left subtree have values less than its own value, and all nodes in its right subtree have values greater than its own value. This ordering property enables efficient searching, insertion, and deletion operations.
Implementing Binary Search Trees in JavaScript
Let's start by defining a Node class to represent individual nodes in the binary search tree:
class Node {
constructor(value) {
this.value = value;
this.left = null;
this.right = null;
}
}
Next, we'll create a BST class to encapsulate the tree structure and provide methods for insertion, searching, and traversal:
class BinarySearchTree {
constructor() {
this.root = null;
}
// Insert a value into the BST
insert(value) {
const newNode = new Node(value);
if (!this.root) {
this.root = newNode;
} else {
this._insertNode(this.root, newNode);
}
}
_insertNode(node, newNode) {
if (newNode.value < node.value) {
if (!node.left) {
node.left = newNode;
} else {
this._insertNode(node.left, newNode);
}
} else {
if (!node.right) {
node.right = newNode;
} else {
this._insertNode(node.right, newNode);
}
}
}
// Search for a value in the BST
search(value) {
return this._searchNode(this.root, value);
}
_searchNode(node, value) {
if (!node) {
return false;
}
if (value === node.value) {
return true;
} else if (value < node.value) {
return this._searchNode(node.left, value);
} else {
return this._searchNode(node.right, value);
}
}
// In-order traversal of the BST
inOrderTraversal(callback) {
this._inOrderTraversal(this.root, callback);
}
_inOrderTraversal(node, callback) {
if (node) {
this._inOrderTraversal(node.left, callback);
callback(node.value);
this._inOrderTraversal(node.right, callback);
}
}
}
Putting it into Practice
Now that we have our BinarySearchTree class implemented, let's use it to create a BST, insert some values, and perform searches:
const bst = new BinarySearchTree();
bst.insert(10);
bst.insert(5);
bst.insert(15);
bst.insert(3);
bst.insert(7);
console.log(bst.search(5)); // Output: true
console.log(bst.search(12)); // Output: false
bst.inOrderTraversal(value => console.log(value)); // Output: 3, 5, 7, 10, 15
Conclusion
Binary search trees are powerful data structures that enable efficient searching, insertion, and deletion operations. By implementing a binary search tree in JavaScript, we've gained a deeper understanding of how they work and how they can be used in real-world scenarios. Experiment with different operations and explore further optimizations to deepen your understanding of this fundamental data structure. Happy coding!
385 views