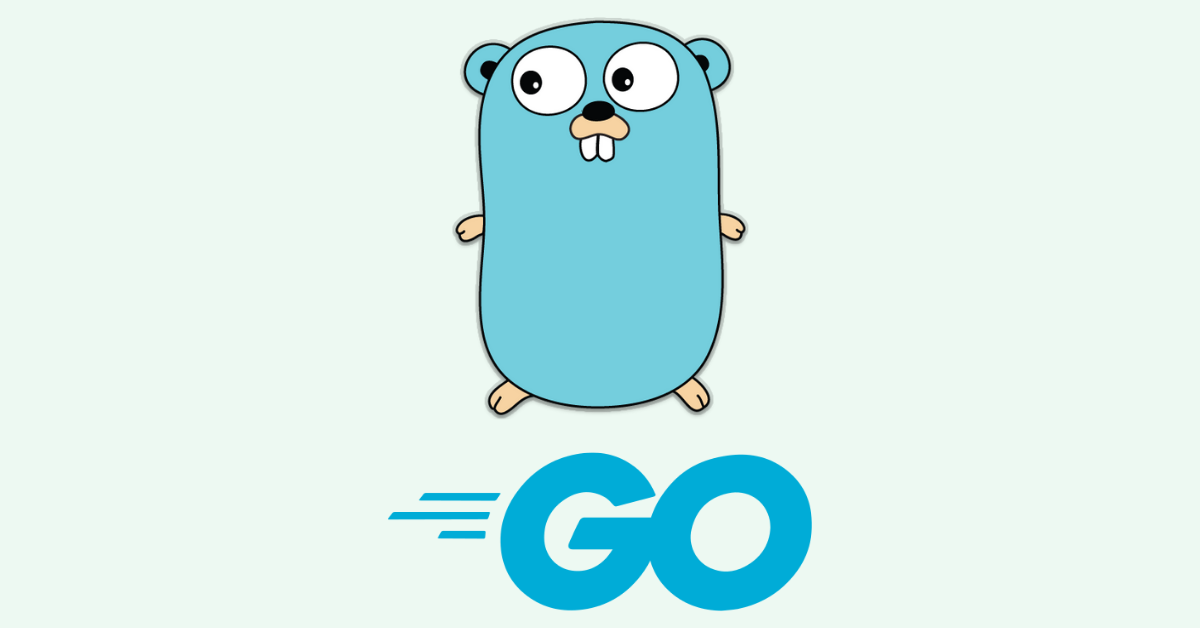
Go, also known as Golang, is a powerful and efficient programming language known for its simplicity and performance. However, like any language, developers can fall into common traps that can lead to bugs, inefficiencies, and headaches. In this article, we'll explore 10 common mistakes in GoLang and provide strategies to avoid them.
Neglecting Error Handling:
One of the most common mistakes in GoLang is neglecting to handle errors properly. Go encourages explicit error handling, using the error type to signal when something goes wrong. Ignoring errors can lead to unexpected behavior or even crashes. Always check and handle errors returned by functions and methods to ensure robustness in your code.
result, err := someFunction()
if err != nil {
// Handle the error
log.Fatal(err)
}
// Continue with the result
Misusing Pointers:
Go provides powerful support for pointers, but misusing them can lead to subtle bugs and memory leaks. Avoid unnecessary pointer indirections and be mindful of the lifetime of objects pointed to by pointers. Ensure proper allocation and deallocation to prevent memory leaks and unintended modifications.
Inefficient String Concatenation:
String concatenation in Go can be inefficient if done incorrectly. Using the + operator to concatenate strings in a loop can result in excessive memory allocations. Instead, use the strings.Builder type or the strings.Join function for efficient string concatenation.
var builder strings.Builder
for _, str := range stringsToConcatenate {
builder.WriteString(str)
}
result := builder.String()
Goroutine Leaks:
Goroutines are lightweight threads in Go, but creating them without proper management can lead to goroutine leaks, where goroutines continue running unnecessarily. Always ensure that goroutines are properly terminated when they're no longer needed, either by using channels for communication or by using synchronization mechanisms like sync.WaitGroup.
Incorrect Use of defer:
The defer statement in Go is used to ensure that a function call is performed before the surrounding function returns. However, deferring functions with closures can lead to unexpected behavior due to the way closures capture variables. Be cautious when deferring functions with closures, as they may not behave as expected.
Sharing Memory by Communicating:
Go's concurrency model relies on communication between goroutines rather than shared memory access. Avoid sharing memory between goroutines directly, as it can lead to race conditions and data races. Instead, use channels to communicate data safely between goroutines, ensuring synchronization and proper access control.
Ignoring Contexts:
The context package in Go provides a way to propagate cancellation signals and deadlines across API boundaries. Ignoring contexts or not passing them along can lead to resource leaks and unresponsive applications. Always pass contexts explicitly and handle cancellation signals appropriately to ensure proper resource management and responsiveness.
Overusing Interfaces:
Interfaces are a powerful feature in Go, but overusing them can lead to unnecessary complexity and performance overhead. Avoid defining interfaces for every type unless necessary. Favor concrete types over interfaces where possible to keep the code simple and efficient.
Not Using go fmt:
Go provides a built-in tool called gofmt to format Go code according to the official style guide. Neglecting to use gofmt can lead to inconsistent code styles across projects and make the code harder to read and maintain. Always use gofmt to format your code consistently and adhere to the official style guide.
Lack of Testing:
Testing is an essential part of software development, but it's often overlooked in Go projects. Neglecting testing can lead to unreliable software and make it difficult to refactor code safely. Write comprehensive tests for your Go code using the built-in testing framework to ensure correctness and maintainability.
By avoiding these common mistakes and following best practices, you can write more reliable, efficient, and maintainable code in GoLang. Remember to always prioritize readability, simplicity, and correctness in your code to ensure long-term success in your projects.
224 views