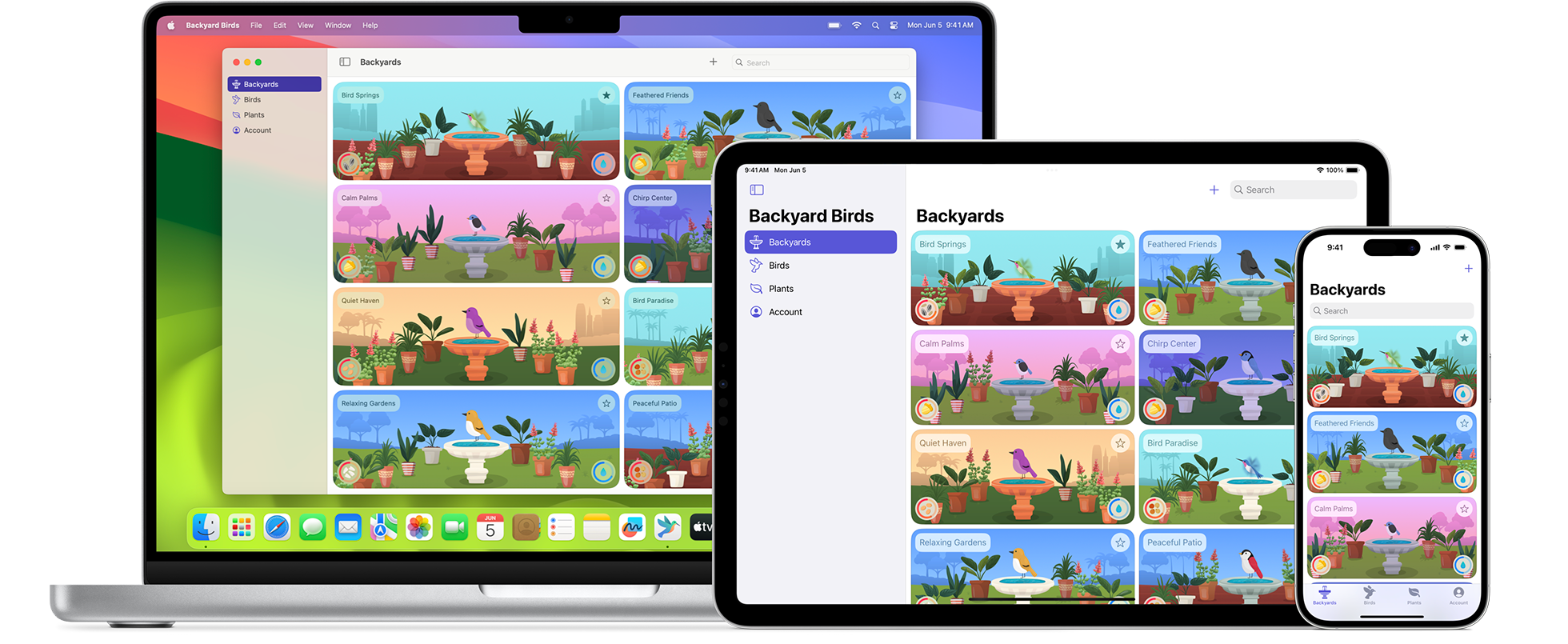
March 28, 2024
Getting Started with SwiftUI Programming: A Beginner's Guide
Are you a beginner looking to dive into app development for iOS, macOS, watchOS, or even tvOS? If so, you've probably heard about SwiftUI, Apple's latest framework for building user interfaces across all Apple platforms. SwiftUI offers a modern and declarative approach to creating interfaces, making it easier than ever for beginners to get started with app development. In this beginner's guide, we'll walk you through the basics of SwiftUI programming, so you can start building your own apps in no time.
What is SwiftUI?
SwiftUI is a user interface toolkit introduced by Apple in 2019, designed to simplify the process of building user interfaces for Apple platforms. It's built using Swift, Apple's programming language, and provides a declarative syntax for defining UI elements and their behavior. With SwiftUI, developers can create highly interactive and visually stunning apps with less code than ever before.
Setting Up Your Development Environment
Before diving into SwiftUI programming, you'll need to set up your development environment. Here's what you'll need:
Xcode: Xcode is Apple's integrated development environment (IDE) for macOS. It includes everything you need to create apps for all Apple platforms, including SwiftUI.
macOS: You'll need a Mac computer to run Xcode and build SwiftUI apps.
SwiftUI Playground: If you're just getting started and want to experiment with SwiftUI without creating a full-fledged Xcode project, you can use SwiftUI Playgrounds, a feature of Xcode that allows you to write SwiftUI code and see live previews of your UI.
Once you have Xcode installed on your Mac, you're ready to start coding with SwiftUI.
Creating Your First SwiftUI Project
To create a new SwiftUI project in Xcode, follow these steps:
Open Xcode and select "Create a new Xcode project" from the welcome window.
Choose a template for your project. For SwiftUI development, select "App" under the iOS, macOS, watchOS, or tvOS category, depending on the platform you want to target.
Enter a name for your project and choose additional options such as organization identifier, language (Swift), and user interface (SwiftUI).
Click "Next" and choose a location to save your project.
Click "Create" to create your project.
Once your project is created, you'll see a default SwiftUI view representing the initial user interface of your app. You can now start customizing this view and adding additional views and functionality to build your app.
Understanding SwiftUI Syntax
SwiftUI uses a declarative syntax, which means you describe the desired outcome of your UI and let SwiftUI handle the underlying implementation details. Here's a simple example of SwiftUI code that displays a text label:
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, SwiftUI!")
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
In this code:
- We define a new struct called ContentView that conforms to the View protocol, which indicates that it represents a view in SwiftUI.
- Inside the ContentView struct, we define a computed property called body, which returns the main content of the view. In this case, it returns a Text view displaying the text "Hello, SwiftUI!".
- We also provide a ContentView_Previews struct that conforms to PreviewProvider, which allows us to preview the view in Xcode's canvas.
Building Your UI
With SwiftUI, building user interfaces is intuitive and straightforward. You can use a variety of built-in views and modifiers to create the desired layout and appearance for your app. Here are some common UI elements you'll use:
- Text: Displays static or dynamic text.
- Image: Displays images from your app's asset catalog or remote URLs.
- Button: A tappable button that performs an action when pressed.
- TextField: Allows users to enter text input.
- List: Displays a scrollable list of items.
- NavigationView: Provides navigation functionality, such as navigation bars and navigation links.
You can combine these views and modifiers to create complex layouts and interactions for your app.
Previewing Your UI
One of the advantages of SwiftUI is the live preview feature in Xcode, which allows you to see how your UI looks and behaves in real-time as you write code. You can use the canvas to interact with your UI and test different device configurations without having to run your app on a simulator or device.
Conclusion
SwiftUI offers an accessible and powerful framework for building user interfaces for Apple platforms. With its declarative syntax and live preview feature in Xcode, beginners can quickly get up to speed with app development and create visually stunning and interactive apps with ease. Whether you're building your first app or exploring new ways to enhance your existing projects, SwiftUI is definitely worth learning for any aspiring iOS or macOS developer. So why wait? Start coding with SwiftUI today and bring your app ideas to life!
545 views