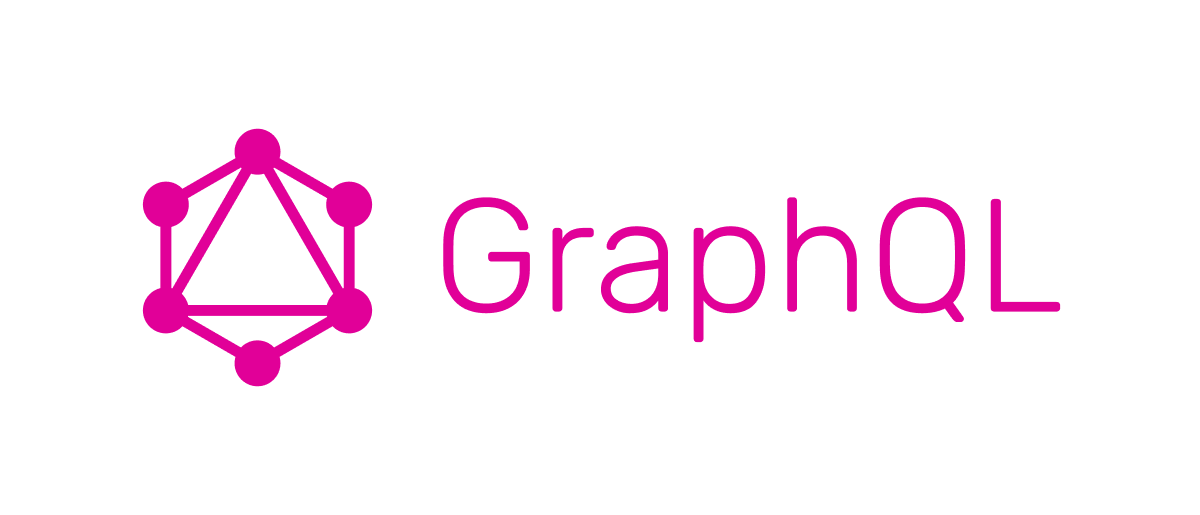
GraphQL has emerged as a powerful tool for building APIs, providing flexibility and efficiency in data fetching. In this guide, we'll delve into what GraphQL is, how it works, and how to implement it in your projects. We'll also provide code examples to illustrate its concepts. Let's begin by understanding the fundamentals of GraphQL.
What is GraphQL?
GraphQL is a query language for your API and a runtime for executing those queries by using a type system you define for your data. Unlike traditional REST APIs, where clients have limited control over the data they receive, GraphQL allows clients to request exactly the data they need and nothing more. This helps in reducing over-fetching and under-fetching of data, leading to improved performance and better client-server communication.
How Does GraphQL Work?
At the core of GraphQL is the schema, which defines the data structure and operations that clients can perform. The schema consists of types representing the available data entities and the operations that can be performed on them. The main components of a GraphQL schema are:
Types: These define the shape of the data available in the API. Each type can have fields representing different attributes of that type.
Queries: These define the read operations that clients can perform to fetch data from the API. Queries are analogous to GET requests in RESTful APIs.
Mutations: These define the write operations that clients can perform to modify data in the API. Mutations are analogous to POST, PUT, PATCH, and DELETE requests in RESTful APIs.
Now, let's see a simple example of a GraphQL schema and how queries and mutations are defined.
type Query {
hello: String
}
type Mutation {
updateHello(newHello: String!): String
}
In this schema, we have a Query type with a single field hello that returns a string. We also have a Mutation type with a single field updateHello that takes a string input and returns a string.
Implementing GraphQL
To implement a GraphQL server, you need a GraphQL execution engine that can interpret and execute GraphQL queries against your schema. There are several libraries and frameworks available in various programming languages for building GraphQL servers. One of the most popular ones is Apollo Server, which we'll use for our example.
Below is a basic example of setting up an Apollo Server with Express.js:
const { ApolloServer, gql } = require('apollo-server-express');
const express = require('express');
const typeDefs = gql`
type Query {
hello: String
}
type Mutation {
updateHello(newHello: String!): String
}
`;
const resolvers = {
Query: {
hello: () => 'Hello World!',
},
Mutation: {
updateHello: (_, { newHello }) => {
// Perform mutation logic here, like updating a database
return newHello;
},
},
};
const server = new ApolloServer({ typeDefs, resolvers });
const app = express();
server.applyMiddleware({ app });
app.listen({ port: 4000 }, () =>
console.log(`Server ready at http://localhost:4000${server.graphqlPath}`)
);
Conclusion
GraphQL offers a modern approach to API development, empowering clients to request precisely the data they need while simplifying the server-side logic. In this guide, we've covered the basics of GraphQL, its core concepts, and how to implement a GraphQL server using Apollo Server with Express.js. By embracing GraphQL, you can build more efficient and flexible APIs to meet the evolving needs of your applications. Happy coding!
255 views