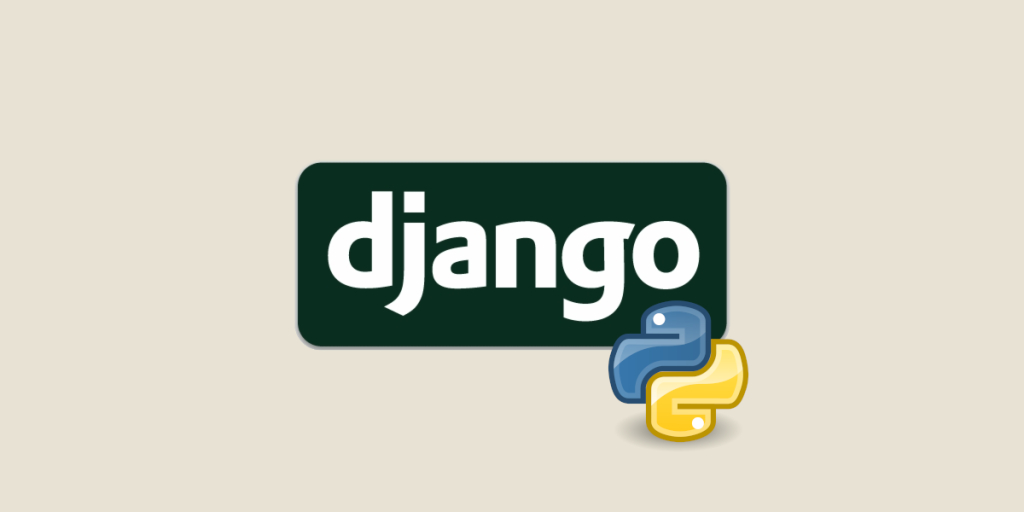
Introduction
Welcome back to another tutorial on my YouTube channel! In this session, we'll be delving into the creation of a Django Weather App. I'm Autry Dev, and I'll guide you through the process of building this application step by step.
Getting Started
Before we dive into the coding part, let's have a quick preview of what the app will look like and its functionalities. The app will display the current weather details of a city, including the city name, weather conditions, time of day, and temperature in Celsius. Users can input the city of their choice to retrieve weather information.
Setting Up
To kickstart our project, I've prepared some starter files which you can find in the repository linked in the description below. These files include the necessary setup for our Django project.
Integrating Weather API
For this tutorial, we'll be using the AccuWeather API, which provides free weather services. However, you'll need to sign up and obtain an API key to access the weather data. The API allows a limited number of calls per day, so make sure to create an account and obtain the API key.
Creating the Weather App
Let's begin by creating a Django app named \"weather\" using the command `python manage.py startapp weather`. Once created, we'll integrate this app into our project by adding it to the installed apps list in the settings.
Routing URLs
Next, we need to configure the URLs. We'll route requests to the weather app by including its URLs in the main `urls.py` file.
from django.urls import path
from .views import home
app_name='weather'
urlpatterns = [
path('',home, name='home')
]
Views and Templates
We'll create a simple view function to render the weather details template. This template will display the weather information fetched from the AccuWeather API.
from django.shortcuts import render
import requests
import os
from .form import LocationForm
def getCityDet(api_key,city):
city_url=f'http://dataservice.accuweather.com/locations/v1/cities/search?apikey={api_key}&q={city}'
city_response= requests.get(city_url)
return city_response.json()[0]
def getTemp(api_key,loc_key):
weather_url=f'http://dataservice.accuweather.com/currentconditions/v1/{loc_key}?apikey={api_key}'
temp_response=requests.get(weather_url)
return temp_response.json()
def home(request):
form=LocationForm(request.POST or None)
if form.is_valid():
city=form.cleaned_data['location']
api_key=os.environ.get('API_KEY')
city_data=getCityDet(api_key,city)
loc_key=city_data['Key']
city_name=city_data['EnglishName']
temp_api_res=getTemp(api_key,loc_key)
temp_value=temp_api_res[0]['Temperature']['Metric']['Value']
is_Day=temp_api_res[0]['IsDayTime']
context={
'form':form,
'city_name':city_name,
'is_Day':is_Day,
'temperature':temp_value
}
return render(request,'weather_app/index.html',context)
return render(request,'weather_app/index.html',context={
'form':form
})
//Base.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Django Weather App | By OtchereDev</title>
</head>
<body>
{% comment %} <form method='POST'>
{{form.as_p}}
<button type='submit'>click me</button>
</form> {% endcomment %}
{% block content %}
{% endblock content %}
</body>
</html>
//weather_api/index.html
{% load static %}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script type="module" src="https://unpkg.com/ionicons@5.4.0/dist/ionicons/ionicons.esm.js"></script>
<script nomodule="" src="https://unpkg.com/ionicons@5.4.0/dist/ionicons/ionicons.js"></script>
<link rel="preconnect" href="https://fonts.gstatic.com">
<link href="https://fonts.googleapis.com/css2?family=Montserrat&family=Roboto:wght@400;500;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="{% static 'css/style.css' %}">
<title>Weather App</title>
</head>
<body>
<div class="popup closed">
<div class="popup_form">
<div class="change">
<ion-icon name="close-outline"></ion-icon>
</div>
<form action="" method="POST">
{% csrf_token %}
<input type="text" name="location" id="id_location" placeholder="Please enter your city">
<button type="submit">Get weather details</button>
</form>
</div>
</div>
<div class="container {% if is_Day %}day{% endif %}">
<div class="weather_content">
<div class="change">
<ion-icon name="cog-outline"></ion-icon>
</div>
<div class="city_name">
<h2>
{% if city_name %}
{{city_name}}
{% else %}
City Name
{% endif %}
</h2>
</div>
<div class="weather_icon">
<ion-icon name="sunny-outline" size="large" class="sun"></ion-icon>
<ion-icon name="moon-outline" size="large" class="moon"></ion-icon>
</div>
<div class="weather_temp">
<h1>
{% if temperature %}
{{temperature}}
{% else %}
16
{% endif %}
°C
</h1>
</div>
</div>
</div>
<script src="{% static 'js/app.js' %}"></script>
</body>
</html>
Handling Form Input
To allow users to input the city of their choice, we'll create a form using Django forms. This form will have a single field for the location. Users can enter the city name, which will be sent to the backend for processing.
from django import forms
class LocationForm(forms.Form):
location=forms.CharField(max_length=500)
Making API Requests
Using the AccuWeather API, we'll make requests to retrieve the location key of the specified city. Then, we'll use this key to fetch the current weather details, including temperature and time of day.
Displaying Weather Details
Once we have the weather data, we'll pass it to the frontend and render it in the template. We'll display the city name, weather conditions, and temperature. Additionally, we'll modify the UI based on the time of day, showing appropriate icons for daytime and nighttime.
Conclusion
Congratulations! You've successfully built a Jungle Weather App that fetches and displays real-time weather information using the AccuWeather API. Feel free to further enhance the app by adding features like weather forecasts for multiple days. Don't forget to share your project and consider subscribing to my channel for more tutorials on various programming projects. Happy coding!
Note
Remember to handle errors and edge cases appropriately, and always adhere to best practices while coding.
283 views