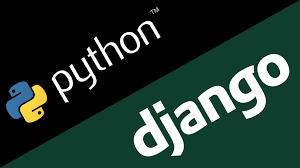
Introduction
In this tutorial, we'll delve into creating a clone of the OMDB API using Django, a high-level Python web framework. The OMDB API, short for Open Movie Database API, is a RESTful web service that provides information about movies. By following along, you'll learn how to set up the project, create endpoints, model the database, and implement various views to handle movie data.
Understanding OMDB API
Before we begin, let's briefly understand the OMDB API. It serves as a repository for movie-related information, including details like titles, descriptions, ratings, actors, directors, and more. Our goal is to replicate its functionality and structure within our Django project.
Setting Up the Project
To get started, you'll need to clone the starter files provided in the repository. These files include the necessary configurations and templates to kickstart our project without the hassle of manual setup.
1. Clone the repository.
2. Modify the `.env` file to configure your database settings, particularly if you're using PostgreSQL.
3. Create a new Django app named `api` using `python manage.py startapp api`.
4. Add the `api` app to your project settings.
5. Set up URL patterns to route requests to the appropriate views within the `api` app.
from api.views import GenreCreateListView, MovieCreateView, MovieDetailView, MovieListView
from django.urls import path
from rest_framework import permissions
from drf_yasg.views import get_schema_view
from drf_yasg import openapi
app_name='own_api'
schema_view = get_schema_view(
openapi.Info(
title="Omdb API Clone",
default_version='v1',
description="The clone of the omdb api",
terms_of_service="https://www.google.com/policies/terms/",
contact=openapi.Contact(email="contact@snippets.local"),
license=openapi.License(name="BSD License"),
),
public=True,
permission_classes=(permissions.AllowAny,),
)
urlpatterns = [
path('all_movies/',MovieListView.as_view()),
path('add_movie/',MovieCreateView.as_view()),
path('movie/<str:title>/',MovieDetailView.as_view()),
path('genre/',GenreCreateListView.as_view()),
path('swagger/', schema_view.with_ui('swagger', cache_timeout=0), name='schema-swagger-ui'),
path('redoc/', schema_view.with_ui('redoc', cache_timeout=0), name='schema-redoc')
]
Modeling the Database
Our database will consist of two main models: `Movie` and `Genre`. The `Movie` model represents individual movies, while the `Genre` model categorizes them based on their genre.
1. Define the `Genre` model with a name field.
2. Define the `Movie` model with fields for title, description, rating, duration, genre (linked via ForeignKey), actors, country, type, poster, director, and language.
from django.db import models
RATINGS=(
('5','5 Star'),
('4', '4 Star'),
('3', '3 Star'),
('2', '2 Star'),
('1', '1 Star'),
)
TYPE=(
('movie','movie'),
('series','series'),
('episode','episode'),
)
class Genre(models.Model):
name=models.CharField(max_length=255)
def __str__(self) -> str:
return self.name
class Movie(models.Model):
title=models.CharField(max_length=400)
description=models.TextField()
created=models.DateField()
rated=models.CharField(choices=RATINGS,max_length=1)
duration=models.CharField(max_length=10)
genre=models.ForeignKey(Genre,on_delete=models.SET_NULL,null=True,blank=True)
actors=models.CharField(max_length=400)
country=models.CharField(max_length=100)
type=models.CharField(choices=TYPE,max_length=15)
poster=models.ImageField()
director=models.CharField(max_length=200)
language=models.CharField(max_length=30)
Implementing Views
With the database models in place, we'll create views to interact with our data.
1. Movie List View: Display a list of all movies.
2. Movie Detail View: Show detailed information about a specific movie.
3. Movie Create View: Allow users to add new movies to the database.
4. Genre List/Create View: Provide functionality to view and create genres.
from django.db.models import query
from api.models import Genre, Movie
from django.shortcuts import render
from rest_framework.generics import CreateAPIView, ListAPIView, ListCreateAPIView, RetrieveAPIView
from .serializers import MovieSerializer,GenreSerializer
from rest_framework.pagination import PageNumberPagination
from django_filters.rest_framework import DjangoFilterBackend
class MovieListView(ListAPIView):
queryset=Movie.objects.all()
serializer_class=MovieSerializer
pagination_class=PageNumberPagination
filter_backends=[DjangoFilterBackend,]
filterset_fields=['title','genre__name','language','type']
class MovieCreateView(CreateAPIView):
serializer_class=MovieSerializer
class MovieDetailView(RetrieveAPIView):
queryset=Movie.objects.all()
lookup_field='title'
serializer_class=MovieSerializer
class GenreCreateListView(ListCreateAPIView):
queryset=Genre.objects.all()
serializer_class=GenreSerializer
pagination_class=PageNumberPagination
filter_backends=[DjangoFilterBackend,]
filterset_fields=['name',]
Serializing Data
To handle data serialization between our models and views, we'll create serializers.
1. Create a serializer for the `Movie` model to convert movie objects into JSON representations and vice versa.
2. Similarly, create a serializer for the `Genre` model.
from rest_framework.serializers import ModelSerializer
from .models import Genre, Movie
class GenreSerializer(ModelSerializer):
class Meta:
model=Genre
fields=['name',]
class MovieSerializer(ModelSerializer):
class Meta:
model=Movie
fields='__all__'
Testing Endpoints
Once everything is set up, run your Django server and navigate to the specified endpoints to test your API.
1. Access the movie list view to see all available movies.
2. Use the movie detail view by providing a specific movie title to get detailed information.
3. Add new movies using the movie create view.
4. Explore genre-related functionalities, such as listing all genres and creating new ones.
Conclusion
By following this tutorial, you've learned how to build a clone of the OMDB API using Django. You've set up the project, modeled the database, implemented views, serialized data, and tested your endpoints. This project serves as a great learning experience for Django beginners and provides a foundation for building more complex web applications in the future.
464 views