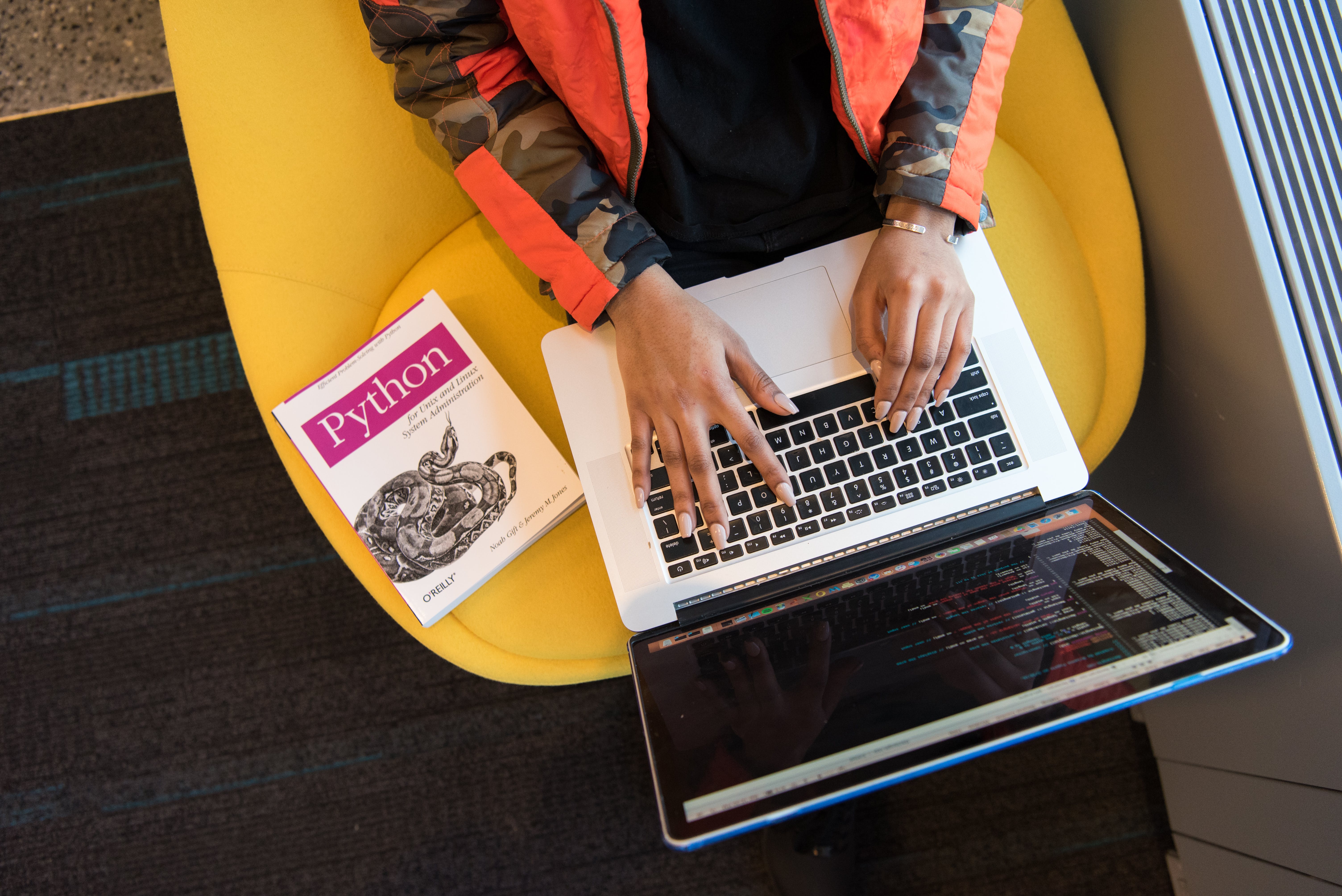
In the world of programming, concurrency plays a crucial role in enhancing the efficiency and performance of applications. Python, with its versatile ecosystem, offers various concurrency models to handle multiple tasks simultaneously. In this blog post, we'll explore different concurrency techniques in Python, including threading, multiprocessing, and asyncio, along with practical code examples to illustrate their usage.
Understanding Concurrency in Python
Concurrency allows programs to execute multiple tasks concurrently, making efficient use of system resources and improving overall performance. In Python, concurrency can be achieved using different approaches, each with its advantages and trade-offs.
1. Threading
Threading in Python enables concurrent execution within the same process. It's suitable for I/O-bound tasks or tasks involving waiting for external resources such as network requests or file I/O.
import threading
def print_numbers():
for i in range(5):
print(i)
def print_letters():
for letter in 'ABCDE':
print(letter)
# Create threads
thread1 = threading.Thread(target=print_numbers)
thread2 = threading.Thread(target=print_letters)
# Start threads
thread1.start()
thread2.start()
# Wait for threads to finish
thread1.join()
thread2.join()
print("Execution completed.")
2. Multiprocessing
Multiprocessing in Python allows running multiple processes simultaneously, leveraging multiple CPU cores. It's suitable for CPU-bound tasks or tasks that can benefit from parallel processing.
from multiprocessing import Process
def square_numbers(numbers):
for number in numbers:
print('Square:', number * number)
if __name__ == '__main__':
numbers = [1, 2, 3, 4, 5]
# Create processes
process = Process(target=square_numbers, args=(numbers,))
# Start process
process.start()
# Wait for process to finish
process.join()
print("Execution completed.")
3. Asynchronous Programming (asyncio)
Asyncio is a built-in library in Python for writing asynchronous code using the async/await syntax. It's suitable for I/O-bound tasks with high concurrency requirements.
import asyncio
async def fetch_data(url):
print("Fetching data from", url)
await asyncio.sleep(2) # Simulate I/O operation
print("Data fetched from", url)
async def main():
tasks = [fetch_data(url) for url in ['url1', 'url2', 'url3']]
await asyncio.gather(*tasks)
if __name__ == "__main__":
asyncio.run(main())
print("Execution completed.")
Conclusion
Concurrency in Python opens up opportunities to write efficient and scalable applications. By understanding and leveraging threading, multiprocessing, and asyncio, developers can create performant solutions catering to diverse requirements. However, it's essential to choose the appropriate concurrency model based on the nature of tasks and system resources available.
492 views