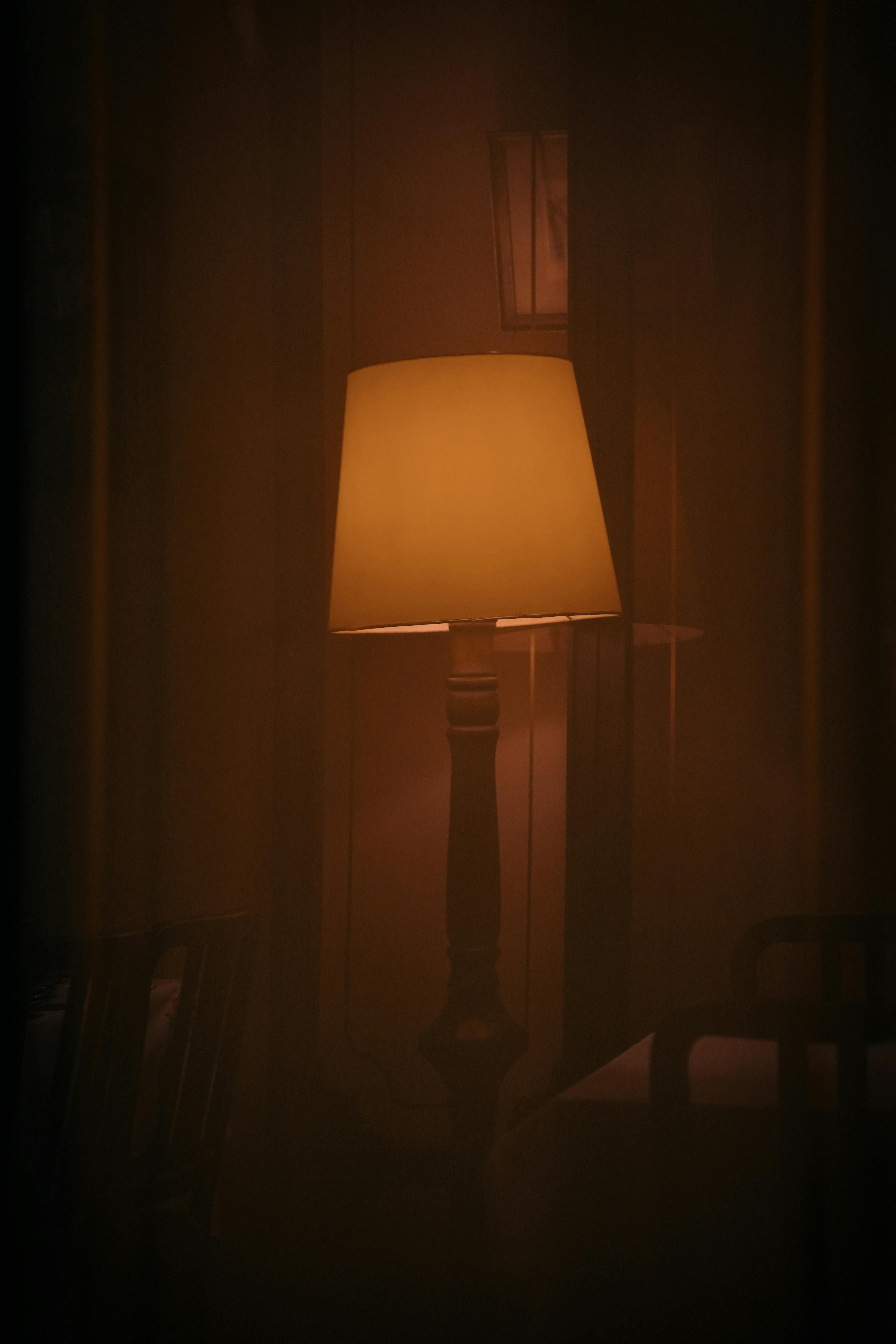
March 07, 2024
Implementing theme mode in any javascript application
Share what you learn in this blog to prepare for your interview, create your forever-free profile now, and explore how to monetize your valuable knowledge.
Implementing a theme mode in a JavaScript application involves creating a mechanism to toggle between different visual themes, such as light mode and dark mode. Here's a basic outline of how you can implement theme mode in a JavaScript application:
- Define Your Themes: Decide on the themes you want to support. Typically, this includes a light theme and a dark theme, but you can add more if needed.
- CSS Styling: Create CSS styles for each theme. These styles will define the colors, fonts, and other visual properties of your application for each theme.
- Toggle Mechanism: Implement a mechanism to toggle between themes. This could be a button, a switch, or any user interface element that triggers the theme change.
- Storage: Optionally, you might want to store the user's theme preference so that it persists across sessions.
Here's a basic example to demonstrate how you can implement theme mode using JavaScript:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Theme Switcher</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="app">
<h1>Welcome to My App</h1>
<button id="theme-toggle">Toggle Theme</button>
</div>
<script src="script.js"></script>
</body>
</html>
/* Light Theme */
body.light {
background-color: #f5f5f5;
color: #333;
}
/* Dark Theme */
body.dark {
background-color: #333;
color: #f5f5f5;
}
document.addEventListener("DOMContentLoaded", function() {
const themeToggle = document.getElementById("theme-toggle");
const body = document.body;
// Check if theme preference is stored
const currentTheme = localStorage.getItem("theme");
if (currentTheme) {
body.classList.add(currentTheme);
}
// Toggle theme when button is clicked
themeToggle.addEventListener("click", function() {
if (body.classList.contains("light")) {
body.classList.remove("light");
body.classList.add("dark");
localStorage.setItem("theme", "dark");
} else {
body.classList.remove("dark");
body.classList.add("light");
localStorage.setItem("theme", "light");
}
});
});
This example sets up a simple theme switcher where clicking the "Toggle Theme" button changes the theme between light and dark. It uses localStorage to persist the user's theme preference. You can expand upon this example by adding more themes, improving the styling, and enhancing the user experience.
260 views
Please Login to create a Question