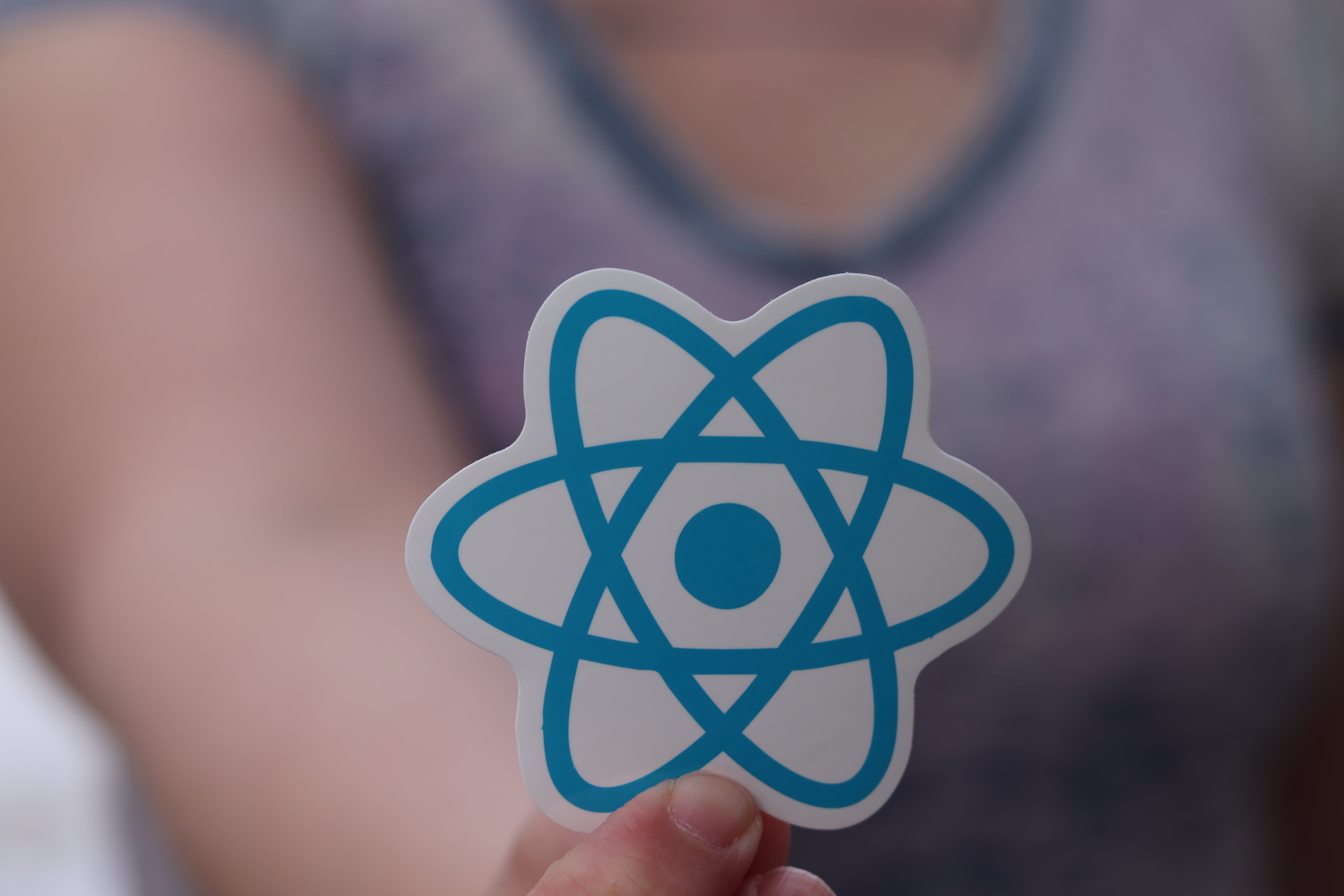
March 11, 2024
Using Tanstack Query with FormData in React
In the realm of modern web development, efficient data management is key to building responsive and scalable applications. Traditionally, managing data in React applications often involved complex state management libraries or manual handling of asynchronous operations. However, with the emergence of libraries like React Query, developers now have a powerful tool at their disposal to streamline data fetching, caching, and synchronization.
One such powerful library is Tanstack Query. Tanstack Query simplifies data fetching, caching, and synchronization in React applications. It provides a concise and intuitive API for fetching and managing data from various sources, including REST APIs, GraphQL endpoints, and more. In this blog post, we'll explore how to use Tanstack Query in conjunction with FormData for handling data in React applications.
Getting Started with Tanstack Query
Before we dive into using Tanstack Query with FormData, let's briefly cover how to set up Tanstack Query in a React project. You can install Tanstack Query along with its dependencies using npm or yarn:
npm install @tanstack/react-query
Once installed, you'll need to set up a QueryClientProvider at the root of your React application to provide the Tanstack Query client to all components:
import { QueryClient, QueryClientProvider } from '@tanstack/react-query';
const queryClient = new QueryClient();
ReactDOM.render(
<QueryClientProvider client={queryClient}>
<App />
</QueryClientProvider>,
document.getElementById('root')
);
With the setup complete, let's move on to integrating Tanstack Query with FormData.
Using Tanstack Query with FormData
FormData is a built-in JavaScript object that provides a simple way to construct and send data in a format that's easily processed by server-side code, particularly for handling form submissions and file uploads. Integrating FormData with Tanstack Query allows us to handle data fetching and submission seamlessly.
Consider a scenario where you have a form in your React application that collects user information such as name, email, and profile picture. You want to send this data to a server endpoint and handle the response using Tanstack Query.
Here's how you can achieve this:
import { useMutation } from '@tanstack/react-query';
const CreateUserForm = () => {
const createUser = useMutation((formData) =>
fetch('/api/users', {
method: 'POST',
body: formData,
}).then((res) => res.json())
);
const handleSubmit = async (event) => {
event.preventDefault();
const formData = new FormData(event.target);
try {
await createUser.mutateAsync(formData);
// Handle success
} catch (error) {
// Handle error
}
};
return (
<form onSubmit={handleSubmit}>
<input type="text" name="name" placeholder="Name" />
<input type="email" name="email" placeholder="Email" />
<input type="file" name="profilePicture" accept="image/*" />
<button type="submit">Submit</button>
</form>
);
};
export default CreateUserForm;
In the above example, we define a component called CreateUserForm, which contains a form with input fields for name, email, and profile picture. When the form is submitted, the handleSubmit function is triggered. Inside this function, we create a new FormData object from the form data and pass it to the createUser mutation function provided by Tanstack Query.
The createUser mutation function takes the FormData object and sends it to the server using the fetch API. Upon successful submission, the response from the server is returned as JSON.
Conclusion
Integrating Tanstack Query with FormData in React applications provides a powerful solution for handling data fetching and submission. By leveraging Tanstack Query's intuitive API for data management and FormData's simplicity for constructing form data, developers can streamline the process of managing data in their React applications.
691 views