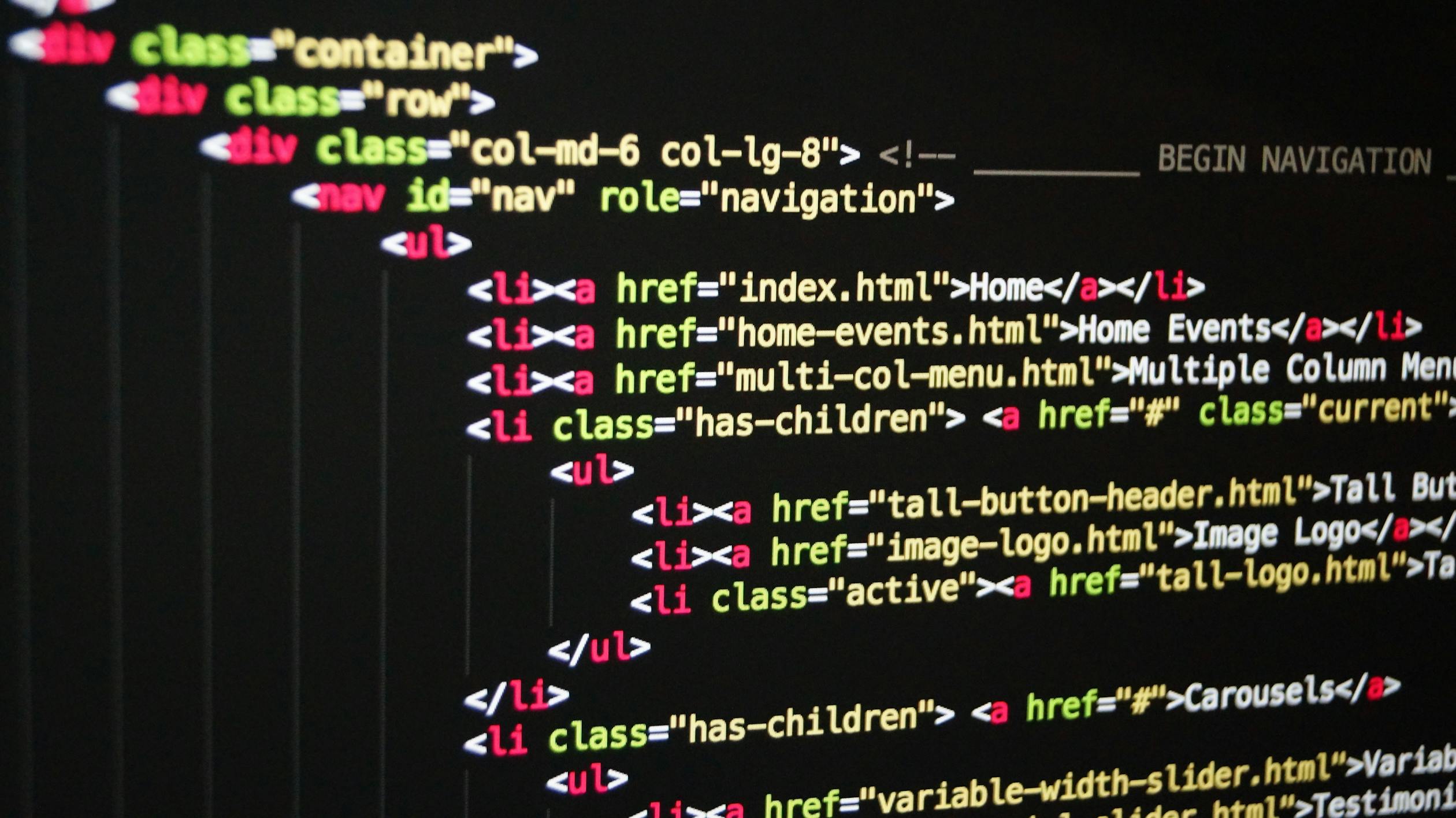
In Laravel, Eloquent is the built-in ORM (Object-Relational Mapping) that makes working with databases and models a breeze. Mutators and casting are two powerful features of Eloquent that help you manipulate and format attributes of your model instances.
Mutators are methods that allow you to modify the attribute values before they are saved to the database or retrieved from it. This can be useful for formatting, sanitizing, or transforming data.
Casting, on the other hand, allows you to specify the data type of an attribute. It automatically converts the attribute's value to the specified data type when retrieving it from the database and back to a suitable format when saving it.
Creating a Model
Let's start by creating a simple model to work with. We'll assume you have a table named users with columns id, name, email, and age. We'll focus on the age column for this tutorial.
Create the Model: Generate a new model named User using Artisan's make:model command.
php artisan make:model User
Defining Attributes & Casting: Open the User model (located in the app directory) and define the attributes and casts array.
namespace App;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $fillable = ['name', 'email', 'age'];
protected $casts = [
'age' => 'integer', // Cast the 'age' attribute to an integer
];
}
Using Mutators
Now, let's create a mutator to automatically capitalize the first letter of the user's name before it's saved to the database.
Create a Mutator Method: Add a mutator method to the User model that will modify the name attribute.
public function setNameAttribute($value)
{
$this->attributes['name'] = ucfirst($value);
}
Using Casting
Casting allows you to specify the data type of an attribute, making it easier to work with data in the expected format.
Using Casting in Model: We've already defined casting in the User model for the age attribute. This means that whenever you access the age attribute, Laravel will automatically cast it to an integer.
Mutators and casting are powerful features in Laravel's Eloquent ORM that help you manipulate and format attribute values seamlessly. Mutators allow you to modify attribute values before they are saved or retrieved, while casting helps you work with attributes in specific data types.
By understanding and utilizing mutators and casting, you can ensure that your data is always in the right format and perform any necessary transformations without much hassle.
661 views