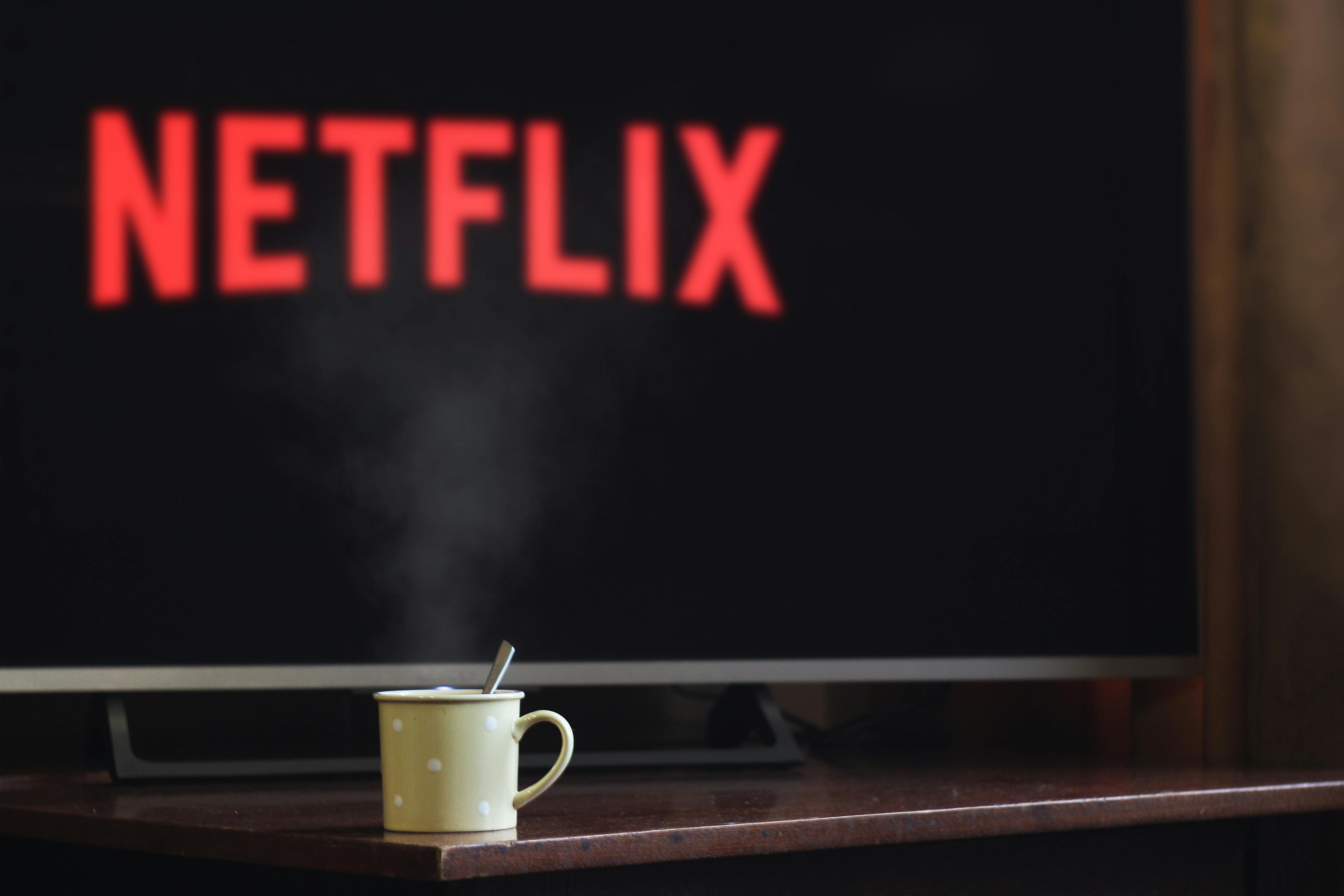
Introduction
In this comprehensive guide, we will walk through the process of building a movie database using Django Admin. We'll cover everything from setting up models to creating views and templates to display and interact with the data.
Setting Up Django Admin
First, let's set up our Django Admin interface. We'll create models for movies, videos, profiles, and custom users. Then, we'll register these models with the Django Admin site to manage them easily.
from django.db import models
from django.contrib.auth.models import AbstractUser
import uuid
AGE_CHOICES=(
('All','All'),
('Kids','Kids')
)
MOVIE_TYPE=(
('single','Single'),
('seasonal','Seasonal')
)
class CustomUser(AbstractUser):
profiles=models.ManyToManyField('Profile')
class Profile(models.Model):
name=models.CharField(max_length=225)
age_limit=models.CharField(max_length=5,choices=AGE_CHOICES)
uuid=models.UUIDField(default=uuid.uuid4,unique=True)
def __str__(self):
return self.name +" "+self.age_limit
class Movie(models.Model):
title:str=models.CharField(max_length=225)
description:str=models.TextField()
created =models.DateTimeField(auto_now_add=True)
uuid=models.UUIDField(default=uuid.uuid4,unique=True)
type=models.CharField(max_length=10,choices=MOVIE_TYPE)
videos=models.ManyToManyField('Video')
flyer=models.ImageField(upload_to='flyers',blank=True,null=True)
age_limit=models.CharField(max_length=5,choices=AGE_CHOICES,blank=True,null=True)
class Video(models.Model):
title:str = models.CharField(max_length=225,blank=True,null=True)
file=models.FileField(upload_to='movies')
Adding Movies to the Admin Panel
Once our models are set up, we'll add them to the Django Admin panel. This step involves creating instances of movies and videos and linking them together.
from django.contrib import admin
from .models import CustomUser,Profile,Movie,Video
# Register your models here.
admin.site.register(CustomUser)
admin.site.register(Profile)
admin.site.register(Movie)
admin.site.register(Video)
Creating Movie Detail Pages
Next, we'll create detailed pages for each movie. These pages will display all the information about a particular movie and allow users to view its details.
Implementing Dynamic Features
We'll enhance our application by adding dynamic features such as playing videos and handling seasonal movies with multiple episodes. This involves writing JavaScript code to interact with the backend and display the appropriate content based on user actions.
Conclusion
Feel free to expand upon it by adding authentication, payment systems, or any other features to suit your needs. Don't forget to subscribe to the channel for more tutorials and updates. Happy coding!
498 views