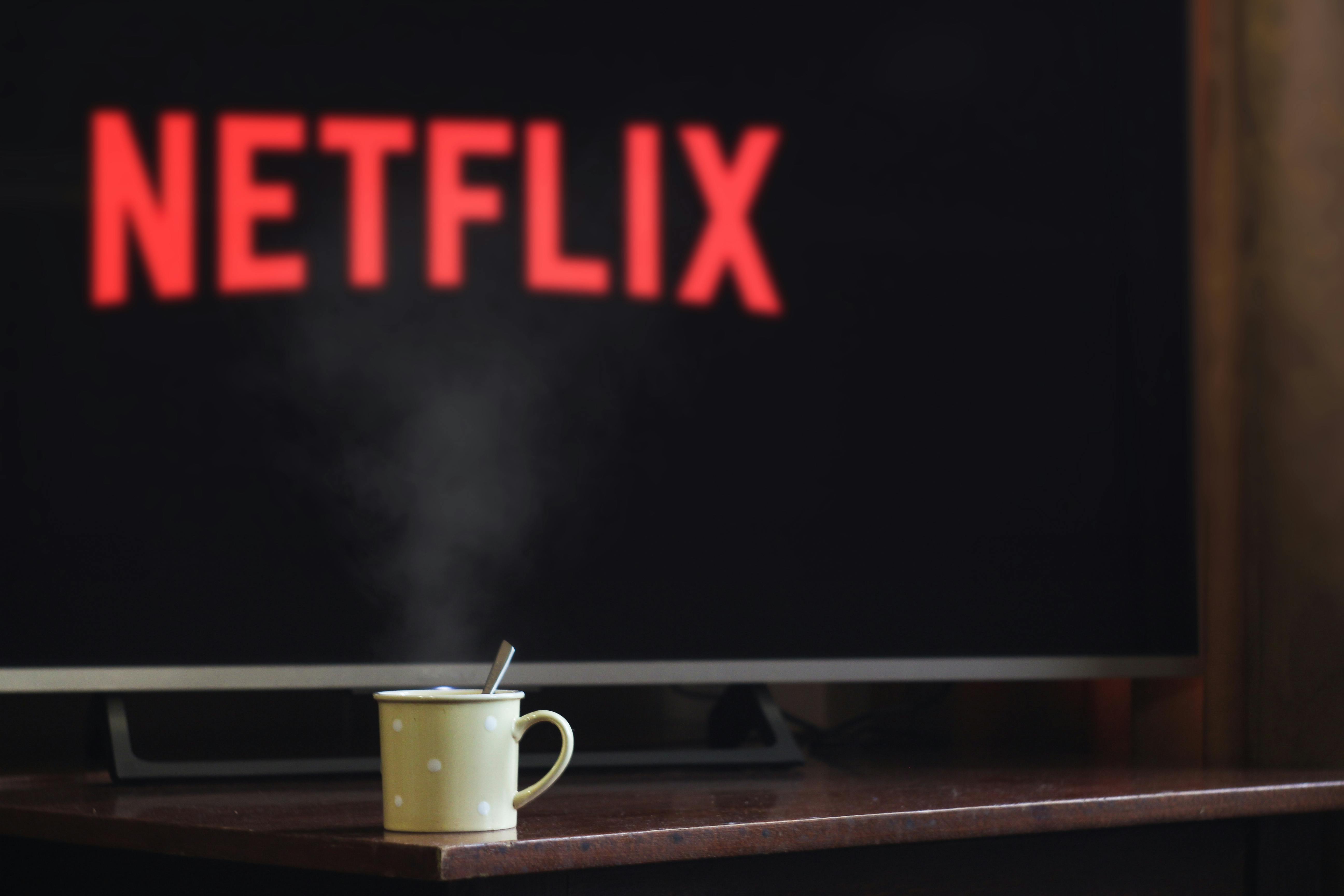
April 15, 2024
Building Your Own Netflix Clone with Django: A Comprehensive Tutorial
In this tutorial, we will embark on a journey to create our very own version of Netflix using Django, one of the most powerful web frameworks out there. Netflix has revolutionized the way we consume entertainment, offering a vast library of movies and TV shows at our fingertips. With Django, we'll be able to build a platform that emulates Netflix's functionality, allowing users to browse, select, and watch their favorite content.
Setting the Stage
Before we dive into the coding part, let's take a moment to understand what we're about to build. Our Netflix clone will feature authentication, user profiles, a selection of movies and TV shows, and the ability to watch them seamlessly. We'll be leveraging Django's capabilities to achieve this, making use of models, views, and templates to craft a functional web application.
Prerequisites
To follow along with this tutorial, you'll need:
- Basic knowledge of Python and Django
- Python installed on your machine
- Django installed in your Python environment
- A text editor or IDE of your choice
Getting Started
### Cloning the Starter Files
To kick things off, we'll clone the starter files from the provided repository. These files contain the initial setup for our Django project, including templates, static files, and some basic configurations.
git clone https://github.com/OtchereDev/django_netflix_clone.git
Once cloned, navigate into the project directory.
### Understanding the Project Structure
The project structure includes:
- Templates: HTML templates for rendering pages
- Static files: CSS, JavaScript, and images
- `settings.py`: Django project settings
- `urls.py`: Main URL configuration
- `models.py`: Database models definition
- `views.py`: View functions or classes
- `forms.py`: Django forms definition
Building the Database Models
Our Netflix clone will require several database models to represent users, profiles, movies, and videos. We'll define these models in the `models.py` file.
#Example model definition
from django.db import models
class CustomUser(models.Model):
Custom user model definition
class Profile(models.Model):
Profile model definition
class Movie(models.Model):
Movie model definition
class Video(models.Model):
Video model definition
Each model will have specific fields to capture relevant data, such as user information, movie details, and video files.
Creating Views
Next, we'll create views to handle user requests and render appropriate responses. We'll use class-based views for simplicity and efficiency.
#Example view definition
from django.views import View
from django.shortcuts import render
class HomeView(View):
def get(self, request, *args, **kwargs):
return render(request, 'index.html')
This view will render the home page template (`index.html`) when a GET request is made to the corresponding URL.
Configuring URLs
We need to map URLs to our views so that Django knows which view to invoke for each request. This is done in the `urls.py` files.
#Main URL configuration
from django.urls import path, include
urlpatterns = [
path('', include('core.urls')),
Other URL patterns
]
Here, we include the URLs defined in the `core` app, where our views are located.
Styling and Assets
To enhance the user experience, we'll add CSS stylesheets and images to our project. These static files will be served by Django's built-in static file server.
Testing and Debugging
Once everything is set up, we'll run the Django development server and test our application in a browser. We'll ensure that all functionalities work as expected and debug any issues that arise.
Conclusion
By following this tutorial, you've learned how to build a Netflix clone using Django. You've gained insights into creating database models, views, URLs, and handling static files. With further customization and enhancements, you can turn this basic clone into a fully functional streaming platform. You can find the full video here Happy coding!
246 views