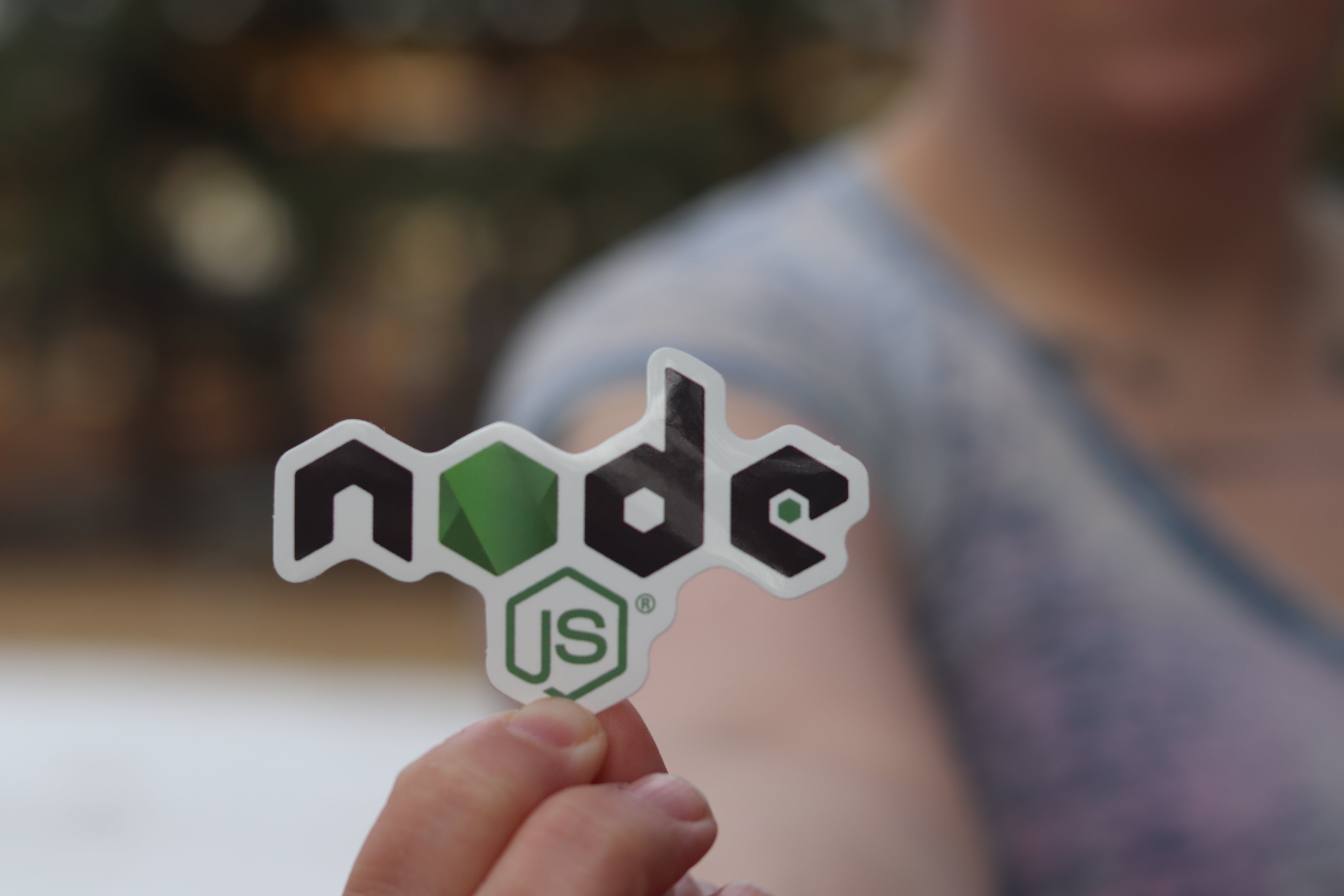
February 09, 2024
Building a RESTful API with Node.js and Express
Share what you learn in this blog to prepare for your interview, create your forever-free profile now, and explore how to monetize your valuable knowledge.
In this tutorial, we'll walk through the process of building a RESTful API using Node.js and Express, two popular tools in the JavaScript ecosystem. We'll cover the basics of setting up a server, defining routes, handling requests, and interacting with a MongoDB database.
First, let's set up our project and install the necessary dependencies
mkdir restful-api
cd restful-api
npm init -y
npm install express mongoose body-parser
Now, let's create our server and define our routes in index.js:
const express = require('express');
const bodyParser = require('body-parser');
const mongoose = require('mongoose');
const app = express();
const PORT = process.env.PORT || 3000;
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/restful-api', { useNewUrlParser: true, useUnifiedTopology: true });
const db = mongoose.connection;
db.on('error', console.error.bind(console, 'MongoDB connection error:'));
// Define schema and model
const Schema = mongoose.Schema;
const ItemSchema = new Schema({
name: String,
description: String,
});
const Item = mongoose.model('Item', ItemSchema);
// Middleware
app.use(bodyParser.json());
// Routes
app.get('/items', async (req, res) => {
try {
const items = await Item.find();
res.json(items);
} catch (err) {
res.status(500).json({ message: err.message });
}
});
app.post('/items', async (req, res) => {
const item = new Item({
name: req.body.name,
description: req.body.description,
});
try {
const newItem = await item.save();
res.status(201).json(newItem);
} catch (err) {
res.status(400).json({ message: err.message });
}
});
// Start server
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
With this setup, we have created a basic RESTful API with two endpoints: GET /items to retrieve all items and POST /items to create a new item.
To test our API, we can use tools like Postman or curl to make requests to our server.
This is just a simple example, but you can extend it by adding more routes, implementing authentication, validation, error handling, and other features to suit your needs.
513 views
Please Login to create a Question