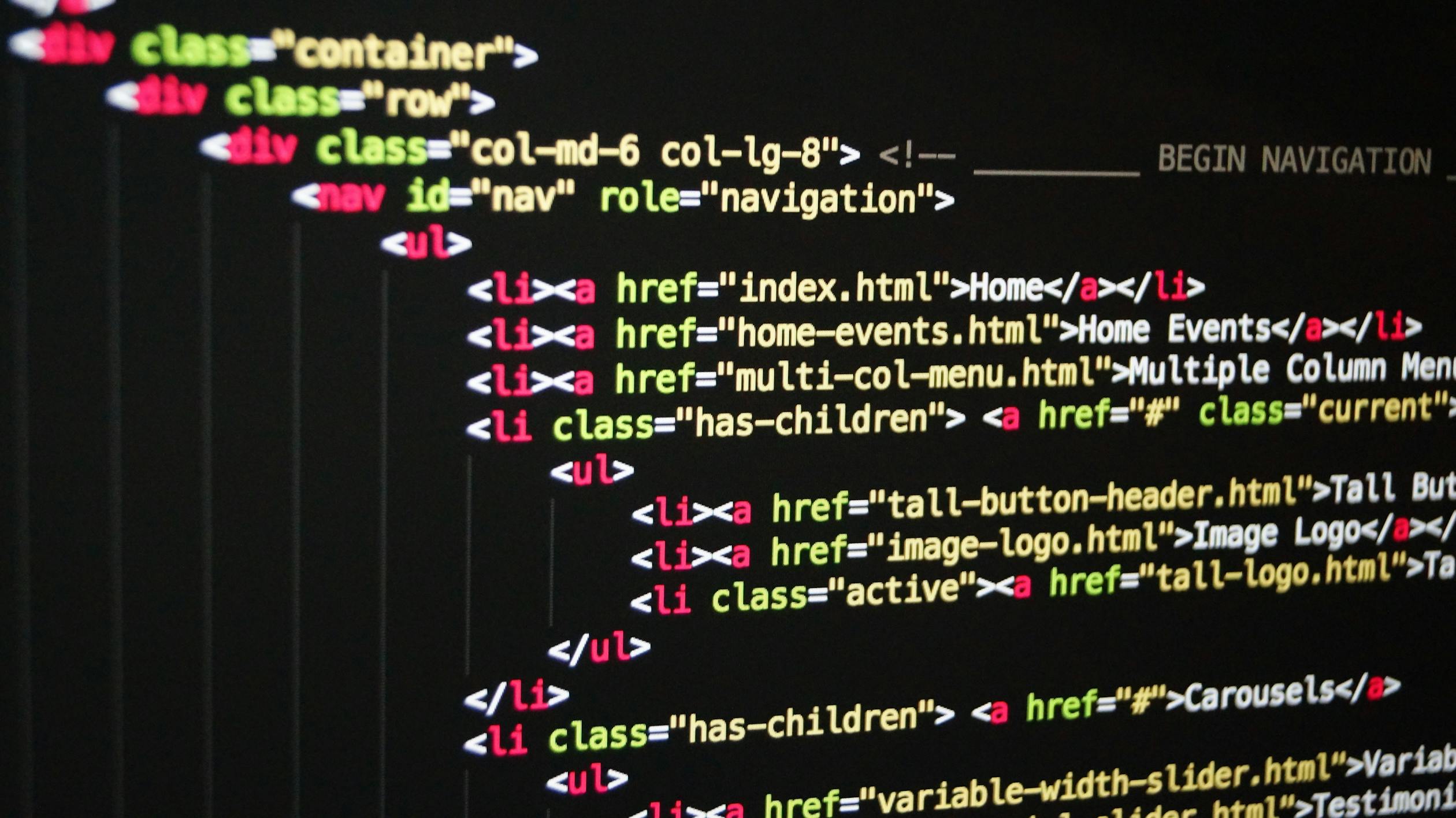
June 11, 2024
Dynamic Web Pages with Django: Views, Templates, and Routing
Introduction:
Welcome back to our Django journey! In the previous blog post, we explored Django models and learned how to define the structure of our database. Now, it's time to focus on the user interface of our web application. In this tutorial, we'll dive into views, templates, and routing in Django to create dynamic web pages that can display data from our database.
Understanding Views:
In Django, a view is a Python function or class that takes a web request and returns a web response. Views are responsible for processing user input, querying the database, and rendering the appropriate HTML content. Django provides two types of views: function-based views and class-based views. For simplicity, we'll start with function-based views in this tutorial.
Creating Views and URL Patterns:
Let's begin by creating a view to display a list of blog posts. Open the views.py file inside your Django app directory (myapp/views.py) and define the following view function:
from django.shortcuts import render
from .models import Blog
def post_list(request):
posts = Blog.objects.all()
return render(request, 'myapp/post_list.html', {'posts': posts})
In this view function:
- We import the render function from django.shortcuts module to render HTML templates.
- We import the Post model from our models file to fetch all blog posts from the database.
- We define a function post_list that takes a request object and queries all posts from the database.
- We pass the list of posts to the render function along with the template name (post_list.html) as a context variable.
Next, we need to define a URL pattern to map this view to a URL. Open the urls.py file inside your Django app directory (myapp/urls.py) and define the following URL pattern:
from django.urls import path
from . import views
urlpatterns = [
path('', views.post_list, name='post_list'),
]
In this URL pattern:
- We import the path function from django.urls module.
- We import the post_list view function from our views file.
- We define a URL pattern that maps the root URL ('/') to the post_list view function.
Creating Templates: Now, let's create an HTML template to display the list of blog posts. Create a new directory named templates inside your Django app directory (myapp/templates) if it doesn't already exist. Inside the templates directory, create a new file named post_list.html and add the following HTML code:
<!DOCTYPE html>
<html>
<head>
<title>Blog Post List</title>
</head>
<body>
<h1>Blog Posts</h1>
<ul>
{% for post in posts %}
<li>{{ post.title }}</li>
{% endfor %}
</ul>
</body>
</html>
In this template:
- We use Django's template language to loop through the list of posts and display the title of each post within an unordered list (<ul>).
Testing the Web Page:
Start the Django development server if it's not already running and navigate to http://127.0.0.1:8000/ in your web browser. You should see a list of blog posts displayed on the page, fetched from the database and rendered using the template we created.
Conclusion:
In this tutorial, we learned how to create views, templates, and URL patterns in Django to build dynamic web pages that can display data from our database. We created a view to fetch blog posts from the database, defined a URL pattern to map the view to a URL, and created an HTML template to render the list of blog posts. Views, templates, and routing are essential components of any Django web application, enabling us to create dynamic and interactive user interfaces. In the next part of this series, we'll explore more advanced features of Django views and templates. Stay tuned for more Django goodness!
423 views