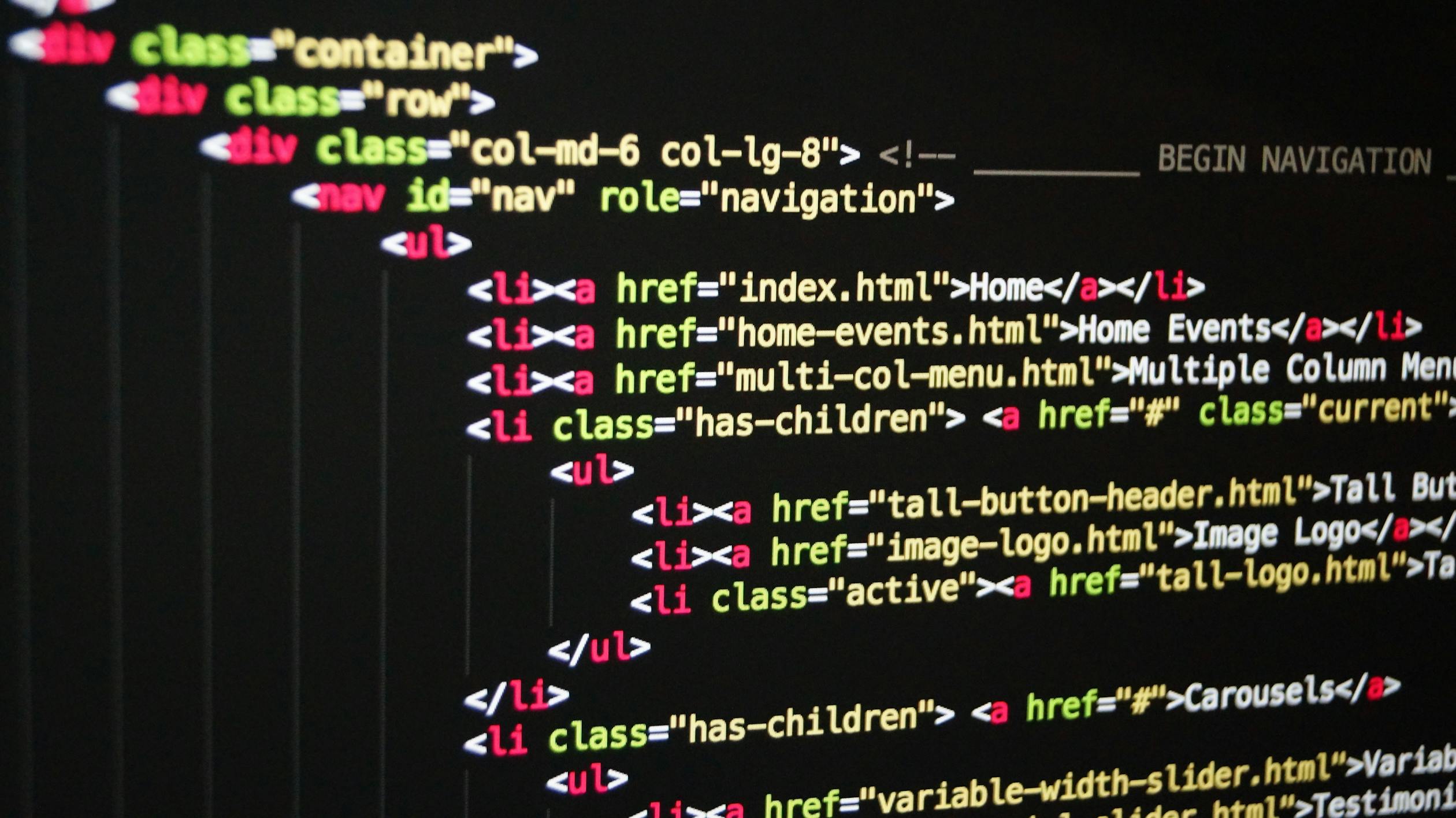
May 14, 2024
Building a Database-driven Web Application with Django Models
Introduction:
Welcome back to our journey of learning Django! In the previous blog post, we set up our Django project and explored its basic structure. Now, we're going to delve deeper into one of the fundamental aspects of Django: models. Models in Django allow us to define the structure of our database and interact with it using Python code. Let's dive in and learn how to build a database-driven web application with Django models.
Understanding Models:
In Django, a model is a Python class that represents a database table. Each attribute of the model class corresponds to a field in the database table. Django provides a wide range of field types such as IntegerField, CharField, DateField, ForeignKey, and many more to define different types of data.
Creating Models for our Web Application:
For our example web application, let's create a simple model to represent a blog post. Open the models.py file inside your Django app directory (myapp/models.py) and define the following model:
from django.db import models
class Blog(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
created_at = models.DateTimeField(auto_now_add=True)
In this model:
title is a CharField representing the title of the blog post.
content is a TextField representing the content of the blog post.
created_at is a DateTimeField representing the date and time when the blog post was created.
Migrations:
After defining our model, we need to create a migration to apply these changes to the database schema. Run the following command in your terminal or command prompt:
python manage.py makemigrations
This command will generate a migration file that contains instructions for creating the corresponding database table. To apply the migration, run:
python manage.py migrate
Interacting with the Database:
Now that our model is defined and the database table is created, we can interact with it using Django's ORM (Object-Relational Mapper). Open up the Django shell by running:
python manage.py shell
Let try this command
>>> from myapp.models import Blog
>>> # Creating a new post
>>> post = Blog.objects.create(title='My First Post', content='Hello, Django!')
>>> # Querying posts
>>> Blog.objects.all()
<QuerySet [<Blog: Blog object (1)>]>
>>> # Retrieving a specific post
>>> post = Blog.objects.get(id=1)
>>> post.title
'My First Post'
>>> # Updating a post
>>> post.title = 'Updated Title'
>>> post.save()
>>> # Deleting a post
>>> post.delete()
Conclusion:
In this tutorial, we learned how to create models in Django to define the structure of our database. We created a simple model for a blog post and explored basic database operations using Django's ORM. Models are a powerful feature of Django that enable us to build database-driven web applications efficiently. In the next part of this series, we'll explore views, templates, and routing in Django to build the user interface for our web application. Stay tuned for more Django goodness!
571 views