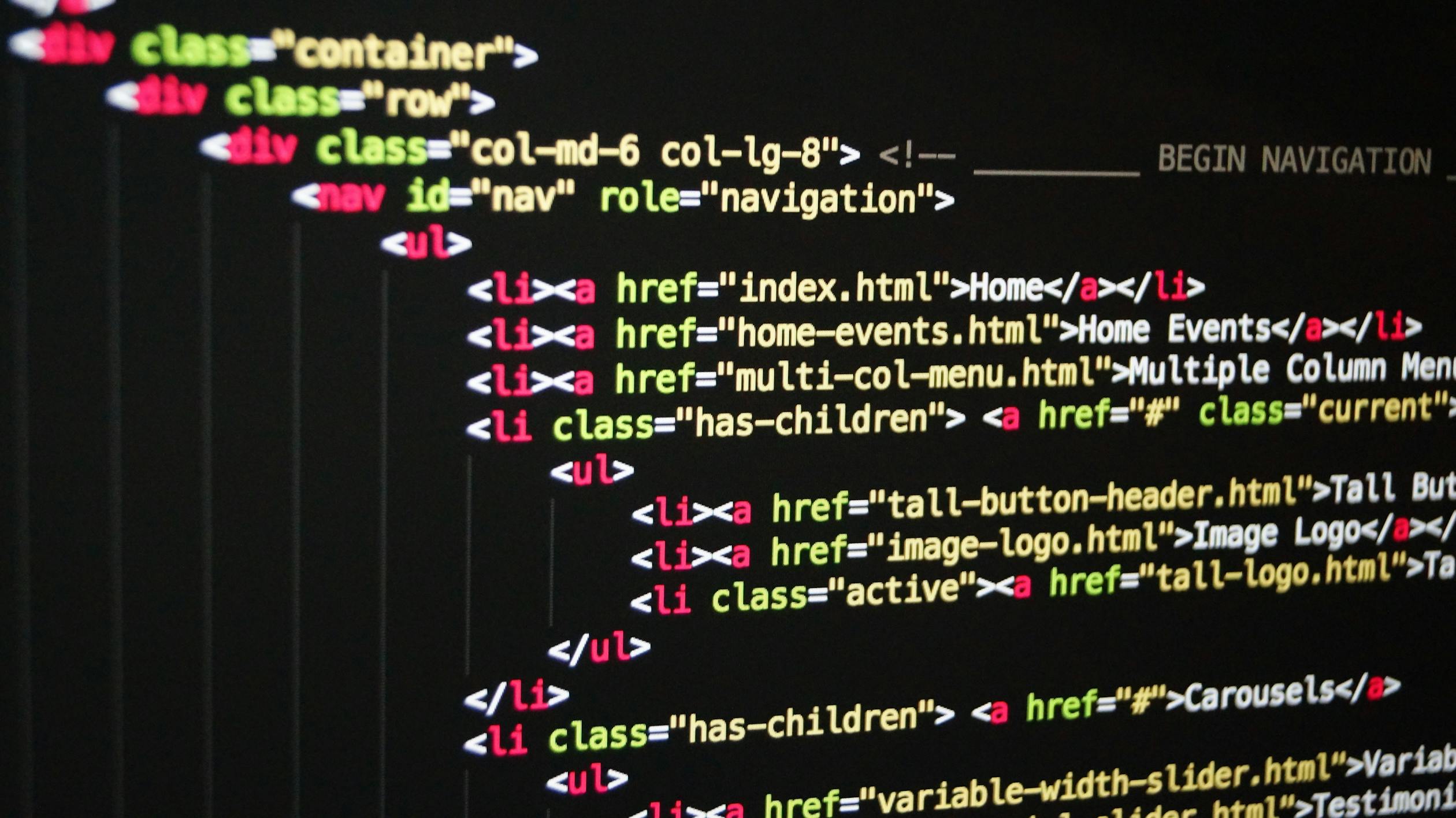
Laravel Events provide a way to decouple various parts of your application. They allow you to create and manage custom events that can be triggered and listened to throughout your application. This helps in building a more modular and maintainable codebase.
Setup Laravel Project
Before we start, make sure you have a Laravel project up and running. If you don't, you can create a new Laravel project using Composer:
composer create-project --prefer-dist laravel/laravel LaravelEvents
Once project is installed go to project folder
cd LaravelEvents
Create the Event
Let's start by creating the event that will be fired when an article is published. Run the following command to generate the event:
php artisan make:event OnPublishArticleEvent
Open the generated event file app/Events/OnPublishArticleEvent.php and update it as follows:
namespace App\Events;
use Illuminate\Broadcasting\InteractsWithSockets;
use Illuminate\Foundation\Events\Dispatchable;
use Illuminate\Queue\SerializesModels;
use App\Models\Article;
class OnPublishArticleEvent
{
use Dispatchable, InteractsWithSockets, SerializesModels;
public $article;
public function __construct(Article $article)
{
$this->article = $article;
}
}
Create the Listener
Next, let's create a listener that will handle the event and send email notifications to subscribers. Run the following command to generate the listener:
php artisan make:listener OnPublishArticleListener
Open the generated listener file app/Listeners/OnPublishArticleListener.php and update it as follows:
namespace App\Listeners;
use App\Events\OnPublishArticleEvent;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Queue\InteractsWithQueue;
use App\Mail\NewArticlePublished;
use Illuminate\Support\Facades\Mail;
class OnPublishArticleListener implements ShouldQueue
{
use InteractsWithQueue;
public function handle(OnPublishArticleEvent $event)
{
$subscribers = [] // Logic to fetch subscribers (You need to implement this)
foreach ($subscribers as $subscriber) {
Mail::to($subscriber->email)->send(new ArticlePublished($event->article));
}
}
}
Create the Mailable
Now, let's create the mailable class that represents the email sent to subscribers when a new article is published. Run the following command to generate the mailable:
php artisan make:mail ArticlePublished
Open the generated mailable file app/Mail/ArticlePublished.php and update it as follows:
namespace App\Mail;
use App\Models\Article;
use Illuminate\Bus\Queueable;
use Illuminate\Mail\Mailable;
use Illuminate\Queue\SerializesModels;
class ArticlePublished extends Mailable
{
use Queueable, SerializesModels;
public $article;
public function __construct(Article $article)
{
$this->article = $article;
}
public function build()
{
return $this->subject('New Article Published')
->view('emails.new-article-published');
}
}
Create Email Template
Create the email template resources/views/emails/new-article-published.blade.php:
<!DOCTYPE html>
<html>
<head>
<title>New Article Published</title>
</head>
<body>
<h1>New Article Published!</h1>
<p>A new article titled "{{ $article->title }}" has been published.</p>
<p>Read the article <a href="{{ route('articles.show', $article->id) }}">here</a>.</p>
</body>
</html>
Fire the Event
In your article publishing logic, make sure to fire the OnPublishArticleEvent event. For example, in your ArticleController:
use App\Events\OnPublishArticleEvent;
public function publishArticle(Article $article)
{
// Publish the article logic
event(new OnPublishArticleEvent($article));
// Return success response
}
Queue Configuration
Since sending emails can be time-consuming, it's a good idea to use queues to handle this process asynchronously. Make sure you have configured your queue driver in the .env file and have the queue worker running
QUEUE_CONNECTION=database
Testing
With everything set up, publish an article in your application and make sure the event is fired and the emails are sent to subscribers.
That's it! You've successfully implemented an event-based system in Laravel that sends email notifications to subscribers when a new article is published.
Remember that this tutorial provides a basic implementation, and you might need to adapt it to your specific application and requirements.
296 views